Discover the textContent property in JavaScript and how it can be used to access and manipulate the text content of an element.
This article provides a detailed explanation of the textContent property, including its differences from other properties like innerText and innerHTML, and how to use it effectively in your code.
So stick around as we go into great detail about textContent, exploring its various functionalities and giving valuable insights on how to use it effectively.
What is textContent property in JavaScript?
The textContent property is a handy tool for getting or setting the text content of a specific node and all its descendants.
It is similar to the nodeValue property but with one key difference: while nodeValue returns the value of the specified node, textContent returns content from all child nodes.
When you set the textContent property, it removes all child nodes and replaces them with a single new text node.
This makes it a powerful tool for accessing and manipulating the text content of an element.
It is also useful for understanding the differences between other properties like innerText and innerHTML and how to use them effectively in your code.
The following is the syntax to return the text content of a node:
element.textContent
or
node.textContent
The following is the syntax to set the text content of a node:
element.textContent = text
or
node.textContent = text
For instance, let’s just say you want to get the text content of an element with the id “sampleP.”
You can do this with the following code:
let text = document.getElementById("sampleP").textContent;
On the other hand, if you want to set the text content of that same element, you can use this code:
document.getElementById("sampleP").textContent = "New text content";
Here are the following browsers that support element.textContent:
✅ Chrome
✅ Edge
✅ Firefox
✅ IE
✅ Safari
✅ Opera
How to use .textcontent in JavaScript?
Here’s an example of how you can use the textContent property in JavaScript to get and set the text content of an HTML element:
// Get the element you want to manipulate
let myElement = document.getElementById("myElement");
// Get the current text content of the element
let currentText = myElement.textContent;
// Set the new text content of the element
myElement.textContent = "New text content";
In this example, we start by selecting the HTML element we want to work with using the getElementById method.
Once we have a reference to that element, we use the textContent property to retrieve its current text content and save it in a variable.
After that, if we want to change the text displayed in the element, we simply use the textContent property again to update it with the new content.
Here’s the complete HTML code example that uses the textContent property to get the text content of an element with the id “greetings” and display it in an alert box:
<!DOCTYPE html>
<html>
<head>
<title>textContent Example</title>
</head>
<body>
<h2 id="greetings">Hello, Itsourcecoders! Welcome to Itsourcecode.com </h2>
<script type="text/javascript">
let content = document.getElementById("greetings");
alert(content.textContent);
</script>
</body>
</html>
In this particular example, there’s an h2 element on the webpage with the id “greetings,” and inside it, there’s the text Hello, “Welcome to Itsourcecode.com.”
To work with this element using JavaScript, we use the getElementById method, which allows us to retrieve a reference to this specific element.
Next, we utilize the textContent property to access the actual text within the h2 element. Lastly, to display this text to the user, we make use of the alert function, which shows the content in an alert box on the screen.
Output:
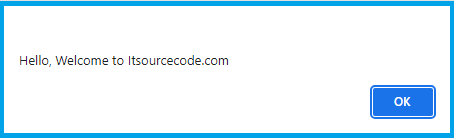
The differences between textContent, innerText, and innerHTML
In JavaScript, there are three properties you can use to work with the content of HTML tags: textContent, innerText, and innerHTML.
📌textContent
This gives you all the text inside an element, including hidden text, but without any HTML tags.
📌innerText
It also gives you the text inside an element, but without any HTML tags or extra spacing.
📌innerHTML
This property gives you everything inside an element, including text, spacing, and HTML tags.
So, depending on what you need, you can choose the property that suits your task best!
Conclusion
In conclusion, the article provides a thorough explanation of the textContent property in JavaScript.
It highlights the key difference between textContent and other similar properties like innerText and innerHTML.
This article demonstrates how to use textContent to get and set the text content of an HTML element, and it includes a practical example showcasing its usage.
We are hoping that this article provides you with enough information that help you understand on how to use JavaScript textContent.
You can also check out the following article:
Thank you for reading itsourcecoders 😊.