The getBoundingClientRect() method is a crucial element of JavaScript. It is a key component of the Document Object Model (DOM) manipulation toolbox.
This approach allows for greater accuracy when estimating the location and dimensions of a supplied HTML element in relation to the visible viewport.
Moreover, the getBoundingClientRect() method provides developers with the ability to orchestrate:
- dynamic layouts
- collision detection
and other complicated interactive functions by encapsulating critical characteristics such as:
- top
- right
- bottom
- left
- width
- height
So this article aims to unviel the intricacies of the getBoundingClientRect() method, revealing its significance and demonstrating how it may be used to create richer online experiences.
What is JavaScript getBoundingClientRect?
The getBoundingClientRect() method is a fundamental feature of the JavaScript DOM (Document Object Model). It returns a set of properties that describe the position and dimensions of a specified element in relation to the viewport.
These properties include:
- top: The distance from the top edge of the viewport to the top edge of the element.
- right: The distance from the left edge of the viewport to the right edge of the element.
- bottom: The distance from the top edge of the viewport to the bottom edge of the element.
- left: The distance from the left edge of the viewport to the left edge of the element.
- width: The width of the element.
- height: The height of the element.
What is the use of getBoundingClientRect in JavaScript?
In JavaScript, the getBoundingClientRect() method is used to retrieve the exact position and dimensions of an element on a webpage relative to the viewport (the visible area of the web browser).
Syntax:
const rect = element.getBoundingClientRect();
Parameters:
The getBoundingClientRect() method does not take any parameters. It is called on a DOM element, and it returns a DOMRect object containing the position and dimensions information of that element relative to the viewport.
Example Usage:
const element = document.getElementById('myElement'); // Replace 'myElement' with the ID of your element
const rect = element.getBoundingClientRect();
console.log('Top:', rect.top);
console.log('Right:', rect.right);
console.log('Bottom:', rect.bottom);
console.log('Left:', rect.left);
console.log('Width:', rect.width);
console.log('Height:', rect.height);
In the example above, element is a reference to the DOM element you want to get the position and dimensions for.
The rect object contains the calculated properties like top, right, bottom, left, width, and height, which you can use for various purposes in your JavaScript code.
How to use getBoundingClientRect in JavaScript?
Here’s how you can use the getBoundingClientRect() method in JavaScript:
Select an Element
- First, you need to select the HTML element for which you want to retrieve the position and dimensions.
const element = document.getElementById('myElement'); // Replace 'myElement' with the ID of your element
Call getBoundingClientRect()
- Next, call the getBoundingClientRect() method on the selected element. This will return a DOMRect object containing position and dimensions information.
const rect = element.getBoundingClientRect();
Access the Properties
- Now, you can access the properties of the DOMRect object to get specific information about the element’s position and dimensions. Common properties include top, right, bottom, left, width, and height.
console.log('Top:', rect.top);
console.log('Right:', rect.right);
console.log('Bottom:', rect.bottom);
console.log('Left:', rect.left);
console.log('Width:', rect.width);
console.log('Height:', rect.height);
You can use these properties for positioning, layout adjustments, collision detection, or any other purposes in your JavaScript code.
Here’s the complete example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>getBoundingClientRect Example</title>
<style>
body {
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #f0f0f0;
}
#myElement {
width: 200px;
height: 150px;
background-color: #3498db;
color: white;
text-align: center;
line-height: 150px;
font-size: 18px;
}
</style>
</head>
<body>
<div id="myElement">Element</div>
<script>
// Select the element
const element = document.getElementById('myElement');
// Get the DOMRect object
const rect = element.getBoundingClientRect();
// Access and use the properties
console.log('Top:', rect.top);
console.log('Right:', rect.right);
console.log('Bottom:', rect.bottom);
console.log('Left:', rect.left);
console.log('Width:', rect.width);
console.log('Height:', rect.height);
</script>
</body>
</html>
Make sure to replace ‘myElement‘ with the actual ID of the HTML element you want to work with. This code will log the position and dimensions information of the element in the browser’s console.
Output:
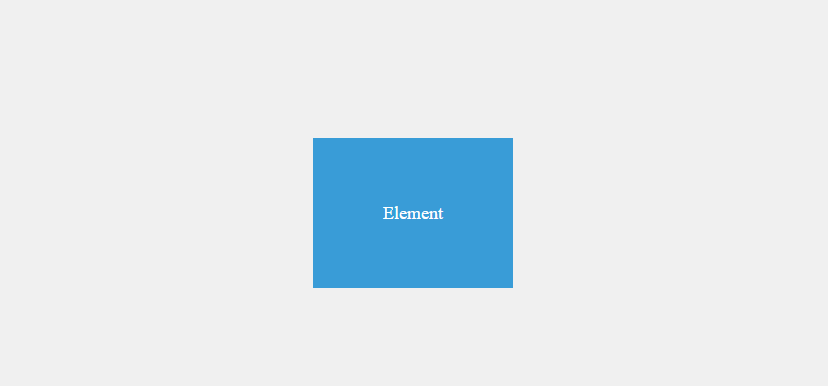
I think we already covered everything we need to know about this article trying to share.
Nevertheless, here are other functions you can learn to enhance your JavaScript skills.
- JavaScript Document write: Examples, Syntax, and Benefits
- How To Reduce Unused JavaScript?
- How To Use JavaScript mapvalues Method?
Conclusion
In conclusion, the ‘getBoundingClientRect()’ method emerges as an essential tool in the JavaScript DOM armory, providing a precise insight into the spatial dimensions of HTML components in the viewport.
Developers may securely manage element positioning, construct responsive designs, and engineer complicated interactions that adapt to different screen sizes and device orientations after reading this article.