One of the fundamental functions in Javascript is the document.write method, which enables developers to generate content dynamically and manipulate the DOM (Document Object Model) in real time.
This article will explore the depths of javascript document write, exploring its examples and application as well as its benefits.
What is JavaScript Document Write?
JavaScript’s document.write() function is used to dynamically generate and insert content into an HTML document while it is being parsed and loaded by a web browser.
It allows you to add text, HTML tags, or JavaScript code directly into the document.
When document.write() is called, the specified content is written to the document at the location where the script tag is placed.
This means that if document.write() is called after the document has finished loading, it will overwrite the entire content of the document, effectively replacing the existing content.
Syntax
document.write(content);
Parameter
The parameter of the document.write() function is the content that you want to write to the document. It can be a string literal or a variable that holds a string value.
Return Value
The document.write() function does not have a return value. It is a void function, which means it does not return any specific value.
Example Programs of JavaScript document write
Example 1: Writing Text
This example shows how to use document.write() to write plain text to the document.
<!DOCTYPE html>
<html>
<body>
<script>
document.write("Hello, @itsourcecode!");
</script>
</body>
</html>
Output:
Hello, @itsourcecode!
Example 2: Writing HTML Tags
In this example, document.write() is used to write HTML tags to the document.
<!DOCTYPE html>
<html>
<body>
<script>
document.write("<h1>This is My heading</h1>");
document.write("<p>This is My paragraph.</p>");
</script>
</body>
</html>
Output:
This is My heading
This is My paragraph.
Example 3: Writing JavaScript Code
document.write() can also be used to write JavaScript code for the document. In this example, a simple script that displays an alert is written using document.write().
<!DOCTYPE html>
<html>
<body>
<script>
document.write("<script>alert('Welcome to, Itsourcecode!');</script>");
</script>
</body>
</html>
Output:
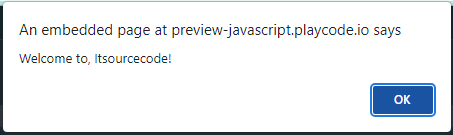
It’s important to note that these examples illustrate the usage of document.write(), but it is not typically recommended to use document.write() extensively in modern web development. Alternative approaches like manipulating the DOM directly or using frameworks and libraries provide more flexibility and control over the content of the document.
Benefits of using document.write in JavaScript
Using document.write offers several advantages when it comes to web development.
Let’s explore some of the key benefits:
1. Dynamic Content Generation
document.write empowers developers to generate content dynamically based on certain conditions or events.
This dynamic approach enables the creation of interactive and personalized web experiences for users.
2. Easy DOM Manipulation
With document.write, developers can effortlessly manipulate the DOM.
It allows the insertion of content at specific positions within the HTML document, making it possible to modify or extend the page’s structure and appearance dynamically.
3. Seamless Integration
The document.write method seamlessly integrates with other Javascript functions and frameworks.
It can be combined with event handlers, conditional statements, and loops to achieve complex interactions and behaviors on a webpage.
4. Quick Prototyping
document.write serves as a valuable tool for rapid prototyping.
It allows developers to quickly test and visualize ideas without the need for a complex setup or external libraries.
Alternative Approach for JavaScript document write
DOM Manipulation:
Instead of directly writing content to the document, you can create and modify elements in the Document Object Model (DOM).
For example, you can use methods like createElement, appendChild, or innerHTML to dynamically add or modify content on your web page.
Example:
// Create a new paragraph element
var paragraph = document.createElement("p");
paragraph.textContent = "This is a dynamically created paragraph.";
// Append the paragraph to a container element
var container = document.getElementById("container");
container.appendChild(paragraph);
innerHTML:
The innerHTML property allows you to set the HTML content of an element. You can use it to replace or add HTML content to an existing element.
Example:
// Replace the content of an element with new HTML
document.getElementById("myElement").innerHTML = "<p>This is the new content.</p>";
// Append HTML content to an element
document.getElementById("myElement").innerHTML += "<p>This is additional content.</p>";
textContent:
If you want to set or change only the text content of an element, you can use the textContent property.
Example:
// Set the text content of an element
document.getElementById("myElement").textContent = "This is the new text content.";
These alternatives provide more flexibility and control over the content manipulation in the DOM compared to document.write.
Anyway here are some of the functions you might want to learn and can help you:
- Toarray JavaScript: Simplifying Array Manipulation
- JavaScript AssertEquals Explained: Ensuring Equality in Testing
Browsers Supports JavaScript document.write()
Most modern web browsers support JavaScript’s document.write() method.
Here are some popular web browsers that support document.write():
- Google Chrome
- Mozilla Firefox
- Apple Safari
- Microsoft Edge
- Opera
It’s important to note that the usage of document.write() is generally discouraged in modern web development.
Conclusion
In conclusion, the document.write method in Javascript serves as a valuable tool for dynamic content generation and DOM manipulation. It offers developers the ability to create engaging and interactive web experiences.
By following best practices and understanding its capabilities, document.write can be harnessed effectively to enhance web development projects.