What is an escape character in JavaScript?
A backslash (\\
) in JavaScript is used as an escape character.
It allows us to insert special characters into a string by changing their original meaning.
For instance, if you want to include a double quote inside a string which is also enclosed by double quotes, you can use an escape character to do so.
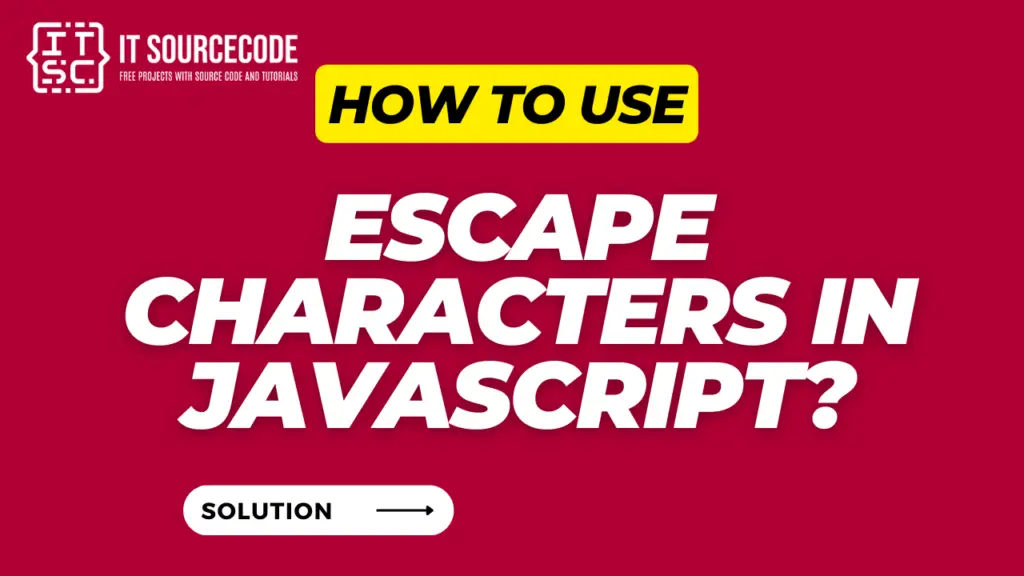
How to use escape characters in JavaScript?
In JavaScript, escape characters are used to insert special characters into strings.
The escape character is a backslash (\). Here’s how you can use them:
Inserting special characters:
You can insert special characters into strings using escape sequences.
For example, to insert a double quote inside a string, you can use \”.
Here’s an example:
let string= "Greetings, \"Hello, welcome to Itsourcecode!\"";
In this example, \” is the escape sequence for a double quote.
The output of this code would be:
Greetings, Hello, welcome to Itsourcecode!
Using escape sequences:
JavaScript supports several escape sequences for different characters.
Here are a few examples:
\' : Single quote
\" : Double quote
\\ : Backslash
\n : New line
\r : Carriage return
\t : Tab
\b : Backspace
\f : Form feed
You can use these escape sequences to insert the corresponding characters into your strings.
Escaping characters in a string:
If you have a string and you want to escape all special characters in it, you can use the JSON.stringify() method.
Here’s an example:
let sampleString = 'This is a "test" string';
let escapedString = JSON.stringify(myString).slice(1, -1);
In this particular case, we’re using JSON.stringify() to handle all the special characters in the string.
After that, we apply .slice(1, -1) to get rid of the double quotes that JSON.stringify() adds to the string.
Conclusion
In conclusion, escape characters in JavaScript are a powerful tool that allows you to insert special characters into strings, which can enhance the readability and functionality of your code.
They are easy to use and supported by all modern browsers, making them a fundamental part of JavaScript programming.
Whether you’re inserting special characters, formatting text, or escaping characters in a string, understanding and using escape characters can help you write more effective and efficient JavaScript code.
You can also check the following article: