In this article, you will learn of how to group JSON data in JavaScript. One of the simple task that developers often encounter is grouping JSON data.
Before we move on into the grouping JSON data, let’s understand first the meaning of JSON.
What is JSON?
JSON, is short for JavaScript Object Notation, is a lightweight data exchange format. It is generally used to relay data between a server and a web application, as well as to store and organize data within an application.
Also, JSON data contains key-value pairs and supports different data types, including strings, numbers, booleans, arrays, and nested objects.
Methods to Group JSON Data using JavaScript
Here are the following methods for grouping the JSON data using JavaScript.
Method 1: Sorting JSON Data
To completely group JSON data, it is usually useful to sort it based on a specific key.
JavaScript provides the sort() method, which enables you to sort an array of objects based on a given property.
Let’s see an example:
const person = [
{ name: 'Glenn', age: 27, city: 'Chicago' },
{ name: 'Caren', age: 29, city: 'San Diego' },
{ name: 'Eliver', age: 23, city: 'Seattle' },
{ name: 'jude', age: 26, city: 'Boston' },
];
// Sort the JSON data by city
person.sort((x, y) => (x.city > y.city ? 1 : -1));
console.log(person);
Output:
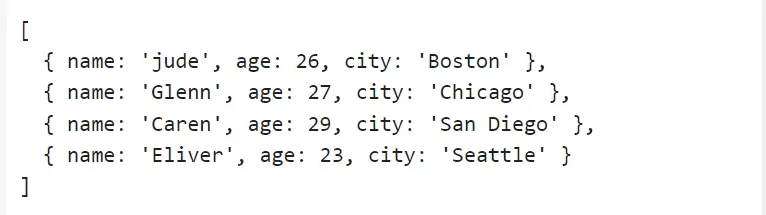
In the above code example, it sorts the person array based on the city property in ascending order.
Sorting the data provides a support for grouping it proficiently.
Method 2: Grouping JSON Data Using a Dictionary
To group JSON data, we can use a dictionary-like structure in JavaScript. One method to done this is by using JavaScript objects.
For example:
const person = [
{ name: 'Glenn', age: 27, city: 'Chicago' },
{ name: 'Caren', age: 29, city: 'San Diego' },
{ name: 'Eliver', age: 23, city: 'Seattle' },
{ name: 'jude', age: 26, city: 'Boston' },
];
const groupedDataExample = {};
person.forEach((persons) => {
if (!groupedDataExample[persons.city]) {
groupedDataExample[persons.city] = [];
}
groupedDataExample[persons.city].push(persons);
});
console.log(groupedDataExample);
Output:
In the above code example, we iterate over the person array and check if the present persons city key already exists as a key in the groupedDataExample object.
If not, we initialize it as an empty array. Then, we push the present person into the corresponding city’s array.
This process completely groups the JSON data based on the city.
Method 3: Grouping JSON Data Using Array Reduce
Another method for grouping JSON data is by using the reduce() method provided by JavaScript arrays.
The reduce() method enables us to increase a result based on the elements of an array.
Let’s look at an example code that uses reduce() method:
const person = [
{ name: 'Glenn', age: 27, city: 'Chicago' },
{ name: 'Caren', age: 29, city: 'San Diego' },
{ name: 'Eliver', age: 23, city: 'Seattle' },
{ name: 'jude', age: 26, city: 'Boston' },
];
const groupedDataExample = person.reduce((personalAccount, persons) => {
if (!personalAccount[persons.city]) {
personalAccount[persons.city] = [];
}
personalAccount[persons.city].push(persons);
return personalAccount;
}, {});
console.log(groupedDataExample);
Using reduce() method on person array, we group persons by city key. For each person, we add it to the persons array in the compiler (personalAccount). Finally, it will result: JSON data grouped by city.
FAQs
Yes, you can group JSON data based on multiple keys. To done this, you can change the grouping logic to consider multiple properties when grouping the data.
If the JSON data consists of nested objects, you can still group it using the same methods mentioned in this article
Absolutely! The grouping logic illustrated in this article serves as a starting point. It depends on your specific requirements, you can customize the grouping logic to suit your needs.
Yes, JavaScript supports asynchronous operations, and you can apply asynchronous methods to group JSON data.
Conclusion
In conclusion, grouping JSON data in JavaScript is a powerful methods that enables you to organize and manipulate data effectively.
By sorting the data and applying JavaScript objects or array reduction, you can effectively group JSON data based on different conducts.