In this article, you’ll discover the solution for the “attributeerror ‘str’ object has no attribute ‘get‘” error message.
This error message indicates that you are attempting to use a method that does not exist for the data type you are working with.
In this case, “get” is a method that belongs to a dictionary object, not a string object. That is why you keep getting this str’ object has no attribute ‘get’ error.
What is “str” object?
In Python, the “str” object is a data type used to represent a string of characters. A string is a sequence of characters enclosed in either single quotes (‘…’) or double quotes (“…”).
It is used to store and manipulate textual data such as strings, sentences, or entire documents. The str object is a built-in object type in Python.
Which means that it is part of the standard Python library and can be used without the need for any external modules or libraries.
What is “attributeerror str object has no attribute get error?
The “attributeerror: ‘str’ object has no attribute ‘get‘” error message typically occurs when you are trying to use the “get” method on a string object in Python.
In addition to that, the “get” method is used to retrieve the value of a dictionary for a given key, but since the “strings” object in Python doesn’t have this method, the interpreter definitely throws an error.
In Python, a dictionary is a data structure that stores key-value pairs, where each key is associated with a specific value. The “get” method is used to retrieve the value associated with a specific key in a dictionary.
In order to fix this error, ensure that you are trying to call the “get” method on a dictionary object and not on a string object. Double-check your code, making sure that you are using the appropriate data types and methods.
Solutions for “attributeerror str object has no attribute get” error
In this section, you’ll discover different solutions that you may use to fix the attributeerror: ‘str’ object has no attribute ‘get’ error that keeps bothering you right now.
If you try to use the “get” method on a string object like this:
my_string = 'Hello world' value = my_string.get('o')
Output:
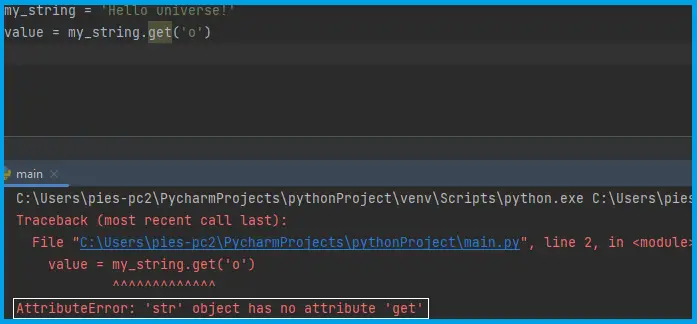
You will get the “attributeError: ‘str’ object has no attribute ‘get’” error, because the “get” method does not exist for string objects.
Solution 1:
You fix this error using a dictionary instead of a string. If you have a dictionary called “sample_dict” that contains the following key-value pairs:
Example 1:
sample_dict = {'a': 1, 'b': 2, 'c': 3}
You can use the “get” method to retrieve the value associated with the key ‘a’ like this:
sample_dict = {'a': 1, 'b': 2, 'c': 3} value = sample_dict.get('b') print(value)
Output:
2
Example 2:
sample_dict = {'Website': 'ITSOURCECODE', 'Year': 2023}
print(sample_dict.get('Website'))
print(sample_dict.get('Year'))
Output:
ITSOURCECODE
2023
Example 3:
sample_list = [1, 2, 3, 4, 5]
print(sample_list.index(4))
Output:
3
Solution 2
sample_str="AEIOU"
ele=sample_str[3]
print(ele)
Output:
O
Solution 3
obj="Input from usr" try: print(obj.get(2)) except: print(" Invalid Input type") print(type(obj))
Output:
Invalid Input type
When the above solutions do not resolve the error, you just have to check your code for typos and syntax errors, use the correct object type, and lastly verify object attributes and methods.
Related Articles for Python Errors
- Attributeerror: str object has no attribute write
- Attributeerror: module ‘jinja2’ has no attribute ‘contextfilter’
- Attributeerror: ‘dataframe’ object has no attribute ‘concat’
Conclusion
By following these solutions, you can easily fix the “attributeerror: ‘str’ object has no attribute ‘get‘” error and other similar errors that occur due to the misuse of the “get” method or the incorrect data type of the object.
Thank you very much for reading to the end of this article. Just in case you have more questions or inquiries, feel free to comment; we would love to hear some thoughts from you.
Aside from that, you can also visit our website for additional information.