The “attributeerror: module ‘jinja2’ has no attribute ‘contextfilter‘” is an error message in Python. That is raised when you are trying to access the contextfilter decorator from the Jinja2 templating library, but the attribute is not found in the module.
In this article, we are going to show you the solution for this “attributeerror: module ‘jinja2’ has no attribute ‘contextfilter” error, so continue to read on to discover the effective solution you may use.
Before we get into the solution, let’s first figure out what the error is all about.
What is contextfilter?
The contextfilter is a decorator in the Jinja2 templating engine for Python that is used to define a filter function. That takes the context of the template rendering into account when processing data.
In addition to that, the “contextfilter” class was added to Jinja2 in version 2.10 as a way to provide more flexibility and power to custom filters. However, it is not supported in the latest versions of Jinja2, which is why some users who are using the latest version of Jinja2 encounter an error.
What is module ‘jinja2’?
The jinja2 module is a popular templating engine for Python that is used to generate dynamic content in web applications, command-line tools, and other software projects.
Aside from that, jinja2 module provides a Python implementation of the Jinja template engine. That includes: a range of functions, filters, and other tools that can be used to customize and extend the behavior of the engine.
To use Jinja2 in a Python program, you typically import the module and create an environment object. That encapsulates the settings and configuration for the template engine.
Here’s an example code using jinja2 to render a simple template:
from jinja2 import Template
template = Template("Hi, {{ name }}!")
rendered_template = template.render(name="Welcome to ITSOURCODE")
print(rendered_template)
Result:
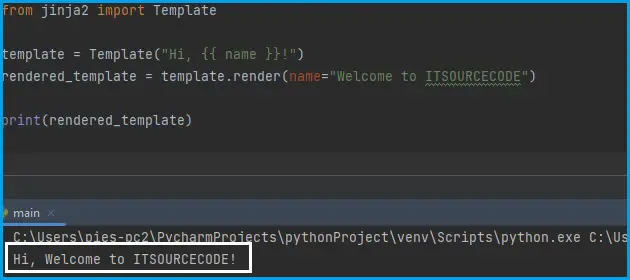
What is “attributeerror: module ‘jinja2’ has no attribute ‘contextfilter'”error?
The error “attributeError: module ‘jinja2’ has no attribute ‘contextfilter‘” happens if you’re using a version of Jinja2 that doesn’t support the contextfilter attribute.
In a simple words, it indicates that the “contextfilter” attribute, which is a function used to create context-sensitive filters for Jinja2 templates, is not available in the “jinja2” module.
In addition to that, this error typically occurs when you are using Jinja2 version 3.0 or the latest versions because the contextfilter attribute was removed or deprecated in the latest version that has been released.
What are the causes of “attributeerror: module ‘jinja2’ has no attribute ‘contextfilter'”error?
This error can occur for various reasons, such as:
- Misspelling of the attribute name.
- An outdated version of Jinja2 that does not support the “contextfilter” attribute.
- It is also possible that the Jinja2 module was not imported correctly resulting in the “contextfilter” attribute not being found.
Solution for “attributeerror: module ‘jinja2’ has no attribute ‘contextfilter'” error
Here’s the following solutions that you can use to fix the error that you are currently facing:
Using pass_context decorator instead of contextfilter
If you’re using an older version of Jinja2 that still supports contextfilter. You can update your code using pass_context decorator instead of contextfilter.
from jinja2 import pass_context
@pass_context
def my_filter(context, value):
return value.upper()
Here’s the example code using pass_context decorator:
from jinja2 import Environment, pass_context
@pass_context
def my_filter(context, value):
return value.upper()
env = Environment()
env.filters['my_filter'] = my_filter
template = env.from_string("{{ my_variable | my_filter }}")
rendered_template = template.render(my_variable="hello world")
print(rendered_template)
Output:
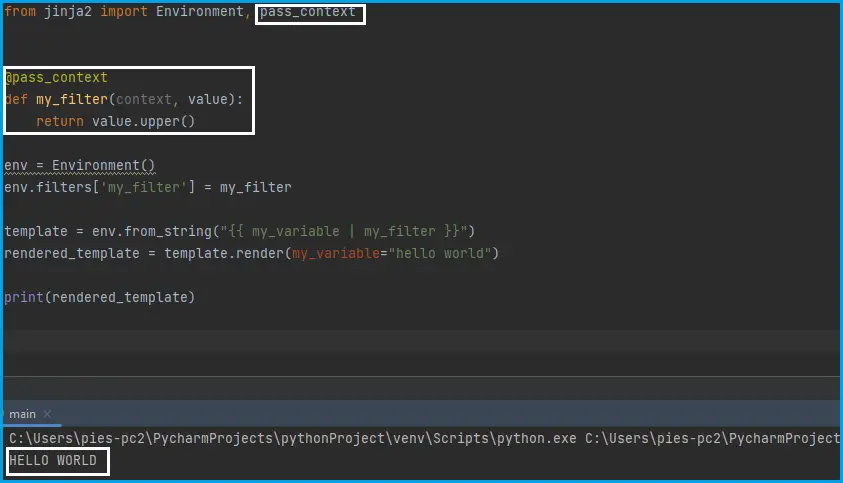
The pass_context decorator is available in Jinja2 2.10 and later and provides similar functionality to contextfilter.
Then, if you’re using a version of Jinja2 that doesn’t support contextfilter or pass_context. You may need to refactor your code to use a different approach.
If you’re using an older version of Jinja2 that still supports contextfilter. You can continue to use it, but be aware that it may not be supported in future versions.
Related Articles for Python Errors
- Attributeerror: ‘dataframe’ object has no attribute ‘concat’
- Attributeerror: ‘api’ object has no attribute ‘search’
- Attributeerror: s3 object has no attribute load_string
Conclusion
Now, you can easily fix the error because this article provides solutions for the “attributeerror: module jinja2 has no attribute contextfilter.” Which is a big help in solving the error that you are currently facing.
We are hoping that this article provide an enough solution to fix the module ‘jinja2’ has no attribute ‘contextfilter’ error message.
Thank you very much for reading to the end of this article. Just in case you have more questions or inquiries, feel free to comment; we would love to hear some thoughts from you. Aside from that, you can also visit our website for additional information.