The “attributeerror: ‘dataframe’ object has no attribute ‘concat‘” error message usually occurs when the concat() function is called on a DataFrame object, but it doesn’t have that method.
In addition to that, if there is a mistake in the way you are using the concat() method, this kind of error is easy to troubleshoot.
If you’re struggling to fix this ‘dataframe’ object has no attribute ‘concat‘, stop worrying because in this article we are going to show you the solution and explain in detail what this error is all about.
What is “attributeerror: ‘dataframe’ object has no attribute ‘concat'”error?
This error, attributeerror: ‘dataframe’ object has no attribute ‘concat,’ usually occurs when you are trying to use the concat() method on DataFrame object but the object does not have this method.
Fortunately, the pandas package provides the concat() method to join two dataframes. This error is easy to fix; you just have to pass a list of DataFrames to the pd.concat function.
Please take a look at our example code below:
import numpy as np
import pandas as pd
np.random.seed(0)
df1 = pd.DataFrame(zip(list('abcde'), list('aeiou')), columns=['consonants', 'vowels'])
df2 = pd.DataFrame(range(5), columns=['numbers'])
pd.concat([df1,df2],axis=1)
Result:
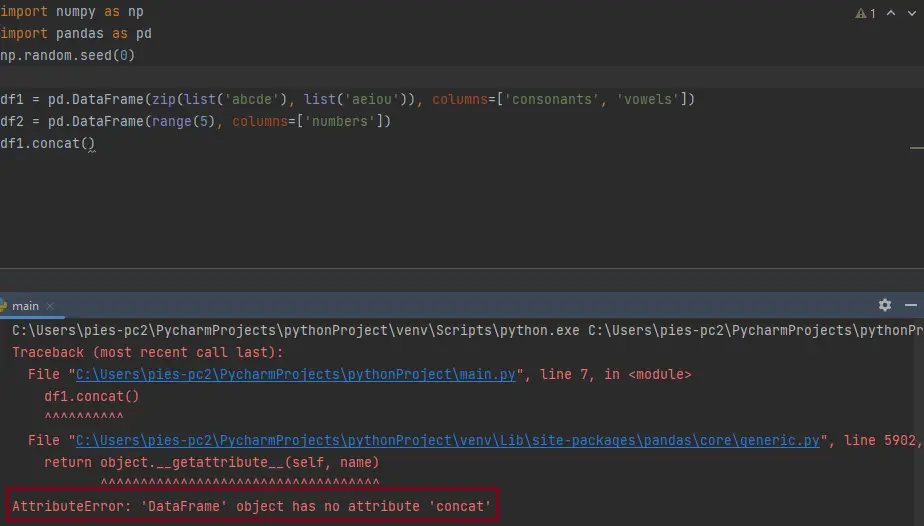
To fix this error, you should first make sure that you have imported the Pandas module and initialized your DataFrame object correctly.
Ensure that you are using the concat() method correctly, and that you are using it on a valid Pandas DataFrame object.
What are the possible causes of attributeerror: ‘dataframe’ object has no attribute ‘concat’ error?
- The most common cause of this error is incorrect syntax used to call the concat() function. It is essential to use the correct syntax to call this function; otherwise, you may encounter this error.
- When you are using an outdated version of Pandas, you may get this error. The concat() function was introduced in version 0.19.0 of pandas. If you are using a version older than this, you will not have access to this function.
- When you have conflicting package versions, this error may occur. For example, if you have both the pandas and dask packages installed, they may conflict with each other and result in this error.
Solution for “attributeerror: ‘dataframe’ object has no attribute ‘concat'” error
Here’s the solution for the ‘dataframe’ object has no attribute ‘concat‘ error that can help you easily fix it.
Please take a look at our example code below:
import numpy as np
import pandas as pd
np.random.seed(0)
df1 = pd.DataFrame(zip(list('abcde'), list('aeiou')), columns=['consonants', 'vowels'])
df2 = pd.DataFrame(range(5), columns=['numbers'])
result = pd.concat([df1, df2], axis=1)
print(result)
Here’s the corrected code that creates the result variable to store the concatenated dataframe and then prints it using the print() function. It will give you the desired result.
Result:
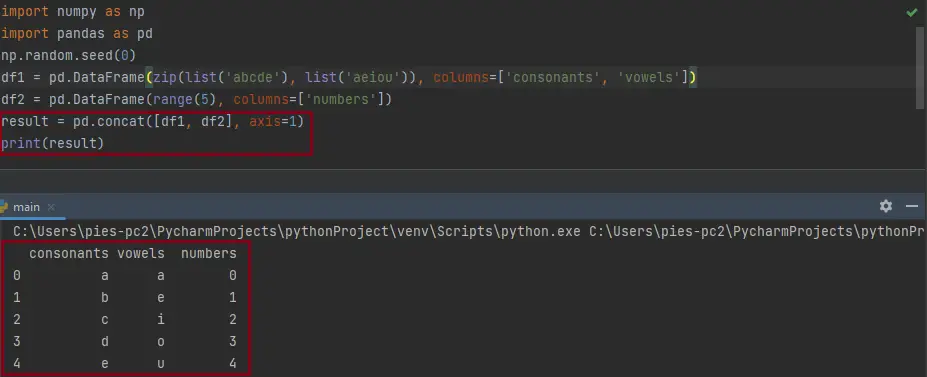
Remember: To concatenate, you need two pd.DataFrame() as an argument of the pandas.concat() method.
Frequently Asked Questions (FAQs)
The concat function is used to concatenate two or more Pandas DataFrames into a single one.
The reason why this attribute error occurs is that you are not using the pandas.concat() method appropriately. You need to apply the concat() method to the single dataframe to fix the error.
Related Articles for Python Errors
- Attributeerror: ‘api’ object has no attribute ‘search’
- Attributeerror: s3 object has no attribute load_string
- Attributeerror: ‘numpy.ndarray’ object has no attribute ‘columns’
Conclusion
This time you can easily fix the error because this article provides solutions for the “attributeerror: ‘dataframe’ object has no attribute ‘concat’”, which is a big help in solving the error that you are currently facing.
Thank you very much for reading to the end of this article. Just in case you have more questions or inquiries, feel free to comment, and you can also visit our website for additional information.