How to Make Media Player Using Visual Basic.net
In this tutorial, i will be teaching you How to Make Media Player Using Visual Basic.net Programming Language that is capable of playing sounds,Videos and viewing of images in different format. So let’s get started. But since Media Player does not exist in the component portion of toolbox, we need to add the Windows Media Player to the toolbox. To do this, just follow the given steps.
What is Visual Basic’s purpose?
The third-generation programming language was created to aid developers in the creation of Windows applications. It has a programming environment that allows programmers to write code in.exe or executable files. They can also utilize it to create in-house front-end solutions for interacting with huge databases. Because the language allows for continuing changes, you can keep coding and revising your work as needed.
However, there are some limits to the Microsoft Visual Basic download. If you want to make applications that take a long time to process, this software isn’t for you. That implies you won’t be able to use VB to create games or large apps because the system’s graphic interface requires a lot of memory and space. Furthermore, the language is limited to Microsoft and does not support other operating systems.
What are the most important characteristics of Visual Basic?
Microsoft Visual Basic for Applications Download, unlike other programming languages, allows for speedier app creation. It has string processing capabilities and is compatible with C++, MFC, and F#. Multi-targeting and the Windows Presentation Framework are also supported by the system, allowing developers to create a variety of Windows apps, desktop tools, metro-style programs, and hardware drivers.
- Go to the toolbox and Right click
- Then select Choose Items and the Customize Toolbox dialog box will open.
- And select Windows Media Player on the COM Components.
- Then click “OK“
- And finally, Windows Media Player control will appear on the current tab.
After this process we can add now Windows Media Player to our Form and the default name of this control is “AxWindowsMediaPlayer1”. Then you are free to change the name of this object based on what you desire for instance you to name it as “WMPlayer” so that it could be more easily to read and remember.
The next process is that we’re going to add other controls to our form such as Listbox, FolderBrowserDialog, MenuStrip and StatusStrip.
Plan the objects and Properties
Object |
Property |
Settings |
Form1 | Name | mainFrm |
Text | Personal Media Player | |
StartPosition | CenterScreen | |
ControlBox | False | |
AxWindowsMediaPlayer1 | Name | myPlayer |
Listbox | Name | List |
MenuStrip1 | Name | MenuStrip1 |
StatusStrip1 | Name | StatusStrip1 |
FolderBrowserDialog1 | Name | FolderBrowserDialog1 |
On the MenuStrip1 we need to add two main menus such Libraries and View. The Libraries has also sub menus like Music, Videos, Images and Exit. And for the View sub menu is only Playlist Editor. This should look like as shown below.
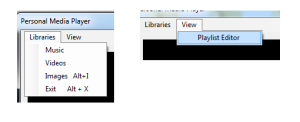
And the final design is look like as shown below.
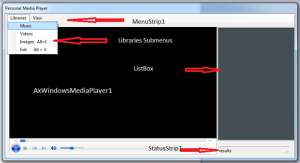
After designing our user interface let’s proceed in adding functionalities to our program. First step double click the main form or we have name it into “mainFrm” to shift our view Designer into view Code. Then on the mainFrm_Load add this code.
[vb]
list.Items.Clear() ‘ clear all currect content of the list
list.Hide() ‘ it will hide the on the main form
myPlayer.Width = 787 ‘ it will resize the width of myPlayer into 787
[/vb]
and on below of our Public Class mainFrm add this declaration of variable that will hold later path of our folder. And it will like this.
[vb]
Public Class mainFrm
Dim folderpath As String
[/vb]
After adding this code we’re going create a sub procedure that we will be using it for our program later.
[vb]
Public Sub jokenresult()
If list.Items.Count > 0 Then
list.Show()
myPlayer.Width = 577
statresult.Text = list.Items.Count & ” Items”
Else
list.Hide()
myPlayer.Width = 787
End If
End Sub
[/vb]
Next we will add functionality to the one sub menu items under libraries the Music. To do this just simply double click the Music sub menu. Then you will be redirected to source code view and add this code so it should now look like as shown below.
[vb]
Private Sub MusicToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MusicToolStripMenuItem.Click
Try
‘it will open the folder dialog where you can select where is the specific folder of your music
FolderBrowserDialog1.ShowDialog()
If DialogResult.OK Then
‘if true that if you click ok on the folder dialog box then
‘it will get the selected path of your folder and store it into di variable
Dim di As New IO.DirectoryInfo(FolderBrowserDialog1.SelectedPath)
‘in this line of code it will get all the specific file that has the .mp3 extension and store it into diar1 variable
Dim diar1 As IO.FileInfo() = di.GetFiles(“*.mp3”)
Dim dra As IO.FileInfo
‘and in this line it will gather all information with regardsto fullpath and names of all files and store it to the folderpath variable
folderpath = di.FullName.ToString
list.Items.Clear()
‘ list the names of all files in the specified directory
For Each dra In diar1
Dim a As Integer = 0
‘ a = a + 1
list.Items.Add(dra)
Next
‘it will call the sub procedure jokenresult() to perform some actions
jokenresult()
End If
Catch ex As Exception
‘if errors occur then the program will catch it and send it back to the user.
MsgBox(ex.Message, MsgBoxStyle.Information)
End Try
End Sub
[/vb]
And this is the sample running program playing a selected music.
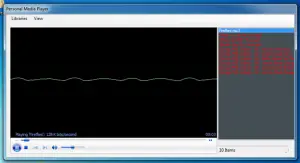
And this is the sample running program playing a selected Movie.
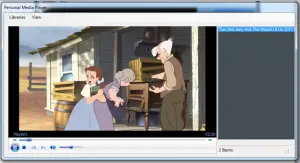
And finally this is all of the source code.
[vb]
‘Description: Personal Media Player that enables user to play Music,Video and pictures etc…
‘Author: Joken Villanueva
‘Date Created:March 23, 2011
‘Modified By:
Public Class mainFrm
Dim folderpath As String
Private Sub MusicToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MusicToolStripMenuItem.Click
Try
‘it will open the folder dialog where you can select where is the specific folder of your music
FolderBrowserDialog1.ShowDialog()
If DialogResult.OK Then
‘if true that if you click ok on the folder dialog box then
‘it will get the selected path of your folder and store it into di variable
Dim di As New IO.DirectoryInfo(FolderBrowserDialog1.SelectedPath)
‘in this line of code it will get all the specific file that has the .mp3 extension and store it into diar1 variable
Dim diar1 As IO.FileInfo() = di.GetFiles(“*.mp3”)
Dim dra As IO.FileInfo
‘and in this line it will gather all information with regardsto fullpath and names of all files and store it to the folderpath variable
folderpath = di.FullName.ToString
list.Items.Clear()
‘ list the names of all files in the specified directory
For Each dra In diar1
Dim a As Integer = 0
‘ a = a + 1
list.Items.Add(dra)
Next
‘it will call the sub procedure jokenresult() to perform some actions
jokenresult()
End If
Catch ex As Exception
‘if errors occur then the program will catch it and send it back to the user.
MsgBox(ex.Message, MsgBoxStyle.Information)
End Try
End Sub
Public Sub jokenresult()
If list.Items.Count > 0 Then
list.Show()
myPlayer.Width = 577
statresult.Text = list.Items.Count & ” Items”
Else
list.Hide()
myPlayer.Width = 787
End If
End Sub
Private Sub list_SelectedIndexChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles list.SelectedIndexChanged
‘the myPlayer will play or display something from the list based on the user selected item
myPlayer.URL = folderpath & “\” & list.SelectedItem.ToString
End Sub
Private Sub VideosToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles VideosToolStripMenuItem.Click
Try
FolderBrowserDialog1.ShowDialog()
If DialogResult.OK Then
Dim di As New IO.DirectoryInfo(FolderBrowserDialog1.SelectedPath)
Dim diar1 As IO.FileInfo() = di.GetFiles(“*.*”)
Dim dra As IO.FileInfo
folderpath = di.FullName.ToString
list.Items.Clear()
For Each dra In diar1
list.Items.Add(dra)
Next
jokenresult()
End If
Catch ex As Exception
MsgBox(ex.Message, MsgBoxStyle.Information)
End Try
MsgBox(folderpath)
End Sub
Private Sub ImagesToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ImagesToolStripMenuItem.Click
Try
FolderBrowserDialog1.ShowDialog()
If DialogResult.OK Then
Dim di As New IO.DirectoryInfo(FolderBrowserDialog1.SelectedPath)
Dim diar1 As IO.FileInfo() = di.GetFiles(“*.jpg”)
Dim dra As IO.FileInfo
folderpath = di.FullName.ToString
list.Items.Clear()
For Each dra In diar1
list.Items.Add(dra)
Next
jokenresult()
End If
Catch ex As Exception
MsgBox(ex.Message, MsgBoxStyle.Information)
End Try
End Sub
Private Sub ExitToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles ExitToolStripMenuItem.Click
Me.Close()
End Sub
Private Sub PlaylistEditorToolStripMenuItem_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles PlaylistEditorToolStripMenuItem.Click
‘in this line if the playlist editor is click then the list will sho on the form.
If PlaylistEditorToolStripMenuItem.Checked = True Then
list.Show()
myPlayer.Width = 577
Else
list.Hide()
myPlayer.Width = 787
End If
End Sub
Private Sub mainFrm_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
list.Items.Clear() ‘ clear all currect content of the list
list.Hide() ‘ it will hide the on the main form
myPlayer.Width = 787 ‘ it will resize the width of myPlayer into 787
End Sub
End Class
[/vb]
You can download the source Code here.Mediaplayer
Readers might read also: