TypeScript is a powerful superset of JavaScript that adds static types and advanced features to the language, enhancing its capabilities for large-scale application development.
One often overlooked but highly useful feature of TypeScript is its support for bitwise operators.
These operators perform operations directly on the binary representations of numbers, making them invaluable for tasks that require low-level data manipulation.
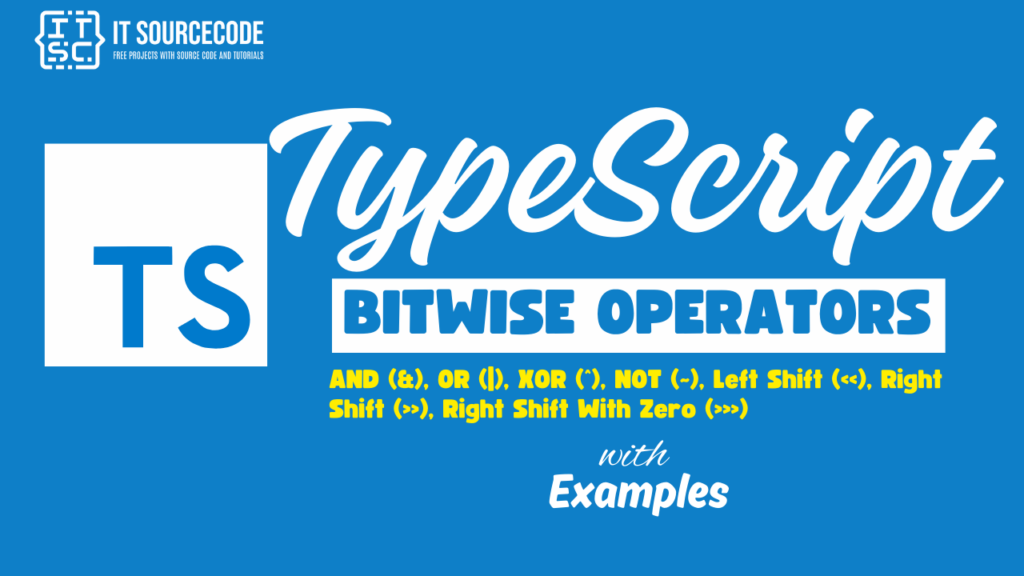
Understanding the TypeScript Bitwise Operators
TypeScript Bitwise operators work at the bit level of data representation.
They allow you to manipulate individual bits within a binary number, providing a high level of control over data processing.
These are operators that are typically used for manipulating bits and performing bitwise operations on one or more operands.
In a nutshell, the Bitwise operators in TypeScript allow you to manipulate individual bits of binary numbers.
The main bitwise operators in TypeScript include:
- AND (&)
- OR (|)
- XOR (^)
- NOT (~)
- Left Shift (<<)
- Right Shift (>>)
- Right Shift With Zero (>>>)
List of Bitwise operators in TypeScript
Each of these Bitwise operators serves a specific purpose and can be combined to perform complex operations.
Let’s explore each operator in detail, with examples to illustrate their usage.
1. AND (&) operator
AND (&) operator carries out a Boolean AND operation on each bit of its integer inputs.
Here’s an example:
console.log(4 & 5)
Here’s the explanation:
In binary representation, 4 is 0100 and 5 is 0101.
The bitwise AND operation (&) compares each bit of the first operand to the corresponding bit of the second operand.
If both bits are 1, the corresponding result bit is set to 1. Otherwise, the corresponding result bit is set to 0. So, 0100 & 0101 results in 0100, which is 4 in decimal.
Therefore, the output of the console.log(4 & 5) is:
Output:
4
2. OR (|) operator
The OR (|) operator carries out a Boolean OR operation on each bit of its integer inputs.
Here’s an example:
console.log(5 | 6)
Output:
5
3. XOR (^) operator
The XOR (^) operator carries out a Boolean exclusive OR operation on each bit of its integer arguments.
An exclusive OR implies that either the first operand is true, or the second operand is true, but not both.
Here’s an example:
console.log(4 ^ 5)
Output:
1
Why the output is 1?
The bitwise XOR operation (^) examines each bit of the first value and matches it with the respective bit of the second value.
If the bits are not the same, the corresponding bit in the result is marked as 1.
If not, the matching bit in the result is marked as 0. So, 0100 ^ 0101 results in 0001, which is 1 in decimal.
4. NOT (~) operator
The NOT (~) operator is a unary operator that works by flipping or reversing all the bits in the operand.
Here’s an example:
console.log(~4)
As you’ve seen in our previous examples, 4 is 0100 in binary. The bitwise NOT operation (~) flips each bit of its operand.
So, ~0100 results in 1011, which is -5 in decimal (in two’s complement representation).
Therefore, the output is:
-5.
5. Right Shift ( >>) operator
The bitwise right shift operator (>>) shifts the bits of the number to the right by a number of bits determined by the right operand.
Here’s an example:
console.log(4 >> 5)
So, 4 >> 5 results in 0000, which is 0 in decimal.
Therefore, the output is:
0
6. Left Shift (<<) operator
The bitwise left shift operation (<<) shifts the bits of the number to the left by the specified number of places.
Here’s an example:
console.log(4 << 5)
In other words, moving a value one position to the left is the same as doubling it, while shifting it two positions to the left is like quadrupling it, and so forth.
So, 4 << 5 results in 10000000, which is 128 in decimal.
Therefore, the output is:
128
7. Right Shift With Zero ( >>> ) operator
This operator functions similarly to the “>>” operator, with the only difference being that the bits shifted on the left side are consistently zero.
Here’s an example:
console.log(4 >>> 5);
The bitwise right shift with zero operator (>>>) shifts the bits of the number to the right by the specified number of places.
So, 4 >>> 5 results in 0000, which is 0 in decimal.
Therefore, the output is:
0
Conclusion
Finally, we’re done exploring the TypeScript Bitwise Operators with Examples, which allow you to manipulate individual bits within a binary number, providing a high level of control over data processing.
I hope this article has given you some insights and helped you understand the TypeScript Bitwise Operators.
If you have any questions or inquiries, please don’t hesitate to leave a comment below.