Hello Guys! This tutorial is all about How to Create a Simple Currency Converter in PHP.
Currency Converter is a calculator that converts the value or quantity of one Currency into the relative values or quantities of other currencies.
So let’s get started:
First create a class and name it “converter.php” and add this code:
<?php class Converter{ private $rateValue; //I have base these rates on USD :) private $rates = [ 'USD' => 1.0, 'GBP' => 0.7, 'EUR' => 0.800284, 'YEN' => 109.67, 'CAN' => 1.23, 'PHP' => 51.74, ]; public function setConvert($amount, $currency_from){ $this->rateValue = $amount/$this->rates[$currency_from]; } public function getConvert($currency_to){ return round($this->rates[$currency_to] * $this->rateValue, 2); } public function getRates(){ return $this->rates; } } ?>
Next is to create our form that converts our set amount from one currency to another currencies. add this code in index.php:
<?php session_start(); include_once('Converter.php'); $converter = new Converter(); $rates = $converter->getRates(); ?> <!DOCTYPE html> <html> <head> <title>Simple Currency Converter in PHP</title> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" rel="stylesheet"> </head> <body>Simple Currency Converter in PHP
$currency){ ?> ">$currency){ ?> "><button type="submit" name="convert" class="btn btn-primary">Convert</button> </form> <?php if(isset($_SESSION['value'])){ ?><?php unset($_SESSION['value']); } ?> </div> </div> </div> </body> </html>
Lastly, we create our PHP code to convert the amount. and add this code in getconvert.php
<?php session_start(); require_once('Converter.php'); if(isset($_POST['convert'])){ $amount = $_POST['amount']; $currency_from = $_POST['currency_from']; $currency_to = $_POST['currency_to']; $converter = new Converter(); $converter->setConvert($amount, $currency_from); $result = $converter->getConvert($currency_to); $out = array(); $out['amount'] = $amount; $out['from'] = $currency_from; $out['result'] = $result; $out['to'] = $currency_to; $_SESSION['value'] = $out; header('location:index.php'); } else{ header('location:index.php'); } ?>
That’s it go and run the program now!
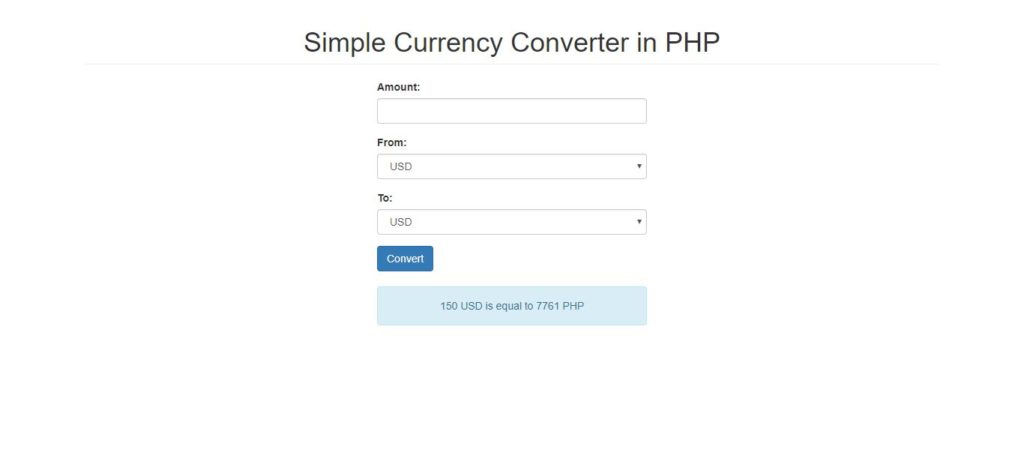
If you have any comments or suggestion about the How to Create a Simple Currency Converter in PHP Message us Directly
Download Source code here:
Other Articles you might read also:
I don’t know why I find it hard to implement this tutorial…
I am looking for the files so I can download them.
Click the download button below