This article focuses on a particular error known as the “ReferenceError: prompt is not defined“ error, providing an in-depth analysis of its specifics.
As someone working with JavaScript, it is common to come across errors during code development.
In fact, these errors serve as valuable indicators, helping us identify issues in our code and leading us toward effective solutions.
Why is Referenceerror prompt is not defined?
The “ReferenceError: prompt is not defined” error occurs when you attempt to use the prompt function in JavaScript, but it is not recognized or defined in the current environment.
The prompt function is typically used in web browsers to display a dialog box that allows the user to input data.
However, the prompt function is not available in all JavaScript environments, such as Node.js or some server-side frameworks.
If you encounter this error, it means that you are trying to use the prompt
function in an environment where it is not supported or available.
Here’s an example program that could result in a “ReferenceError: prompt is not defined” error:
var name = prompt("Please enter your name:");
console.log("Hello, " + name + "!");
In this program, we’re trying to use the prompt function to ask the user to enter their name and store it in the name variable.
Then, we’re printing a greeting message to the console.
However, suppose you run this code in an environment that doesn’t support the prompt function, such as Node.js or some other non-browser environment.
In that case, you will encounter the “ReferenceError: prompt is not defined” error.
This error occurs because the prompt function is not recognized or available in that particular environment.
How to fix Referenceerror prompt is not defined?
To fix the “ReferenceError: prompt is not defined” error, you need to ensure that you’re running your code in an environment that supports the prompt function.
Here are a few solutions with example programs:
Use a Web Browser
The prompt function is typically available in web browsers. You can create an HTML file and run it in a web browser to use the prompt function.
Here’s an example:
<!DOCTYPE html>
<html>
<head>
<title>Example Program</title>
<script>
var name = prompt("Please enter your name:");
console.log("Hello, " + name + "!");
</script>
</head>
<body>
<h1>Hello, World!</h1>
</body>
</html>
This HTML file prompts the user to enter their name using the prompt function and logs a greeting message to the console.
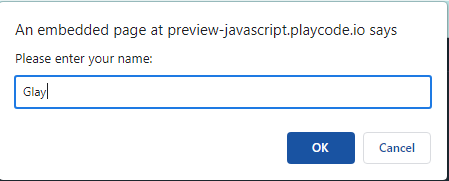
Output:
Hello, Glay!
Use a Different Input Mechanism
If you’re not in a web browser environment, you can use a different input mechanism provided by your specific environment.
For example, in a Node.js environment, you can use the readline module to read user input from the command line.
Here’s an example:
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout
});
rl.question('Please enter your name: ', function(name) {
console.log('Hello, ' + name + '!');
rl.close();
});
In this Node.js example, we use the readline module to create an interface for reading user input.
The question method prompts the user to enter their name, and the provided callback function processes the input and logs a greeting message to the console.
Use a JavaScript Framework or Library
If you’re working with a specific JavaScript framework or library, check its documentation to find out if it provides an alternative input mechanism.
Many frameworks offer their own methods or components for user input.
For example, in React, you can use form elements and event handlers to capture user input.
Here’s a simple example using React:
import React, { useState } from 'react';
function App() {
const [name, setName] = useState('');
const handleInputChange = (event) => {
setName(event.target.value);
};
return (
<div>
<input type="text" value={name} onChange={handleInputChange} />
<p>Hello, {name}!</p>
</div>
);
}
export default App;
In this React example, we use the useState hook to create a state variable called name.
The handleInputChange function is triggered when the input value changes, updating the name state.
The greeting message is rendered dynamically based on the entered name.
Alternative solution for prompt is not defined
Another effective solution is we have to install “prompt-sync“. Then make sure we have also installed an updated version of npm and node.
To do this enter the following code in the terminal:
npm install prompt-sync
Another solution is by using the google console. In this case, we will obtain the user’s name, we use a prompt dialog box.
After the user enters their name, it will be displayed on the screen.
Here’s how you can access the developer tools in Chrome: Open Chrome, go to the menu, select “More Tools,” and then choose “Developer Tools” (you can also use the shortcut Ctrl+Shift+I).

Anyway, here are other fixed errors you can refer to when you might encounter them.
Conclusion
To sum it up, the “ReferenceError: prompt is not defined” error occurs when you try to use the prompt function in an environment where it is not recognized or available. This error is typically encountered in non-browser environments such as Node.js or specific server-side frameworks.
We hope this guide has assisted you in resolving the error effectively.
Until next time! 😊