The error Only Size-1 arrays can be converted to Python scalars is shown when you pass an array to a function that expects a single value.
In many numpy methods, the only kind of parameter that is allowed is a scalar value. So, if you give the method either a one-dimensional or a multidimensional array, it will throw this error. Since more and more methods only accept a single parameter, you can expect to see this error a lot.
TypeError: Only Size-1 Arrays Can Be Converted To Python Scalars?
Only Size 1 Arrays Error is a TypeError that happens when you use an array as a parameter in a function or method that only accepts a single scalar value.
Many functions have built-in ways to handle errors so that programs don’t crash and the inputs to the function are checked. If the validation isn’t done, the Python program will immediately crash, which can cause problems.
This error is shown by numpy.int() and numpy.float() because they have parameters with only one value. TypeError comes from an invalid data type, so sending an array as a parameter can cause it.
Due to the above mistakes, you need to be extra careful when using NumPy. A common mistake I saw was this:
import numpy as ny
import matplotlib.pyplot as matplot
def ycord(xcord):
return ny.int(xcord)
xcord = ny.arange(1, 5, 2.7)
matplot.plot(xcord, ycord(xcord))
matplot.show()
This is what we get as a result of the above input:
TypeError: only size-1 arrays can be converted to Python scalars
How To Solved TypeError: Only Size-1 Arrays Can Be Converted To Python Scalars?
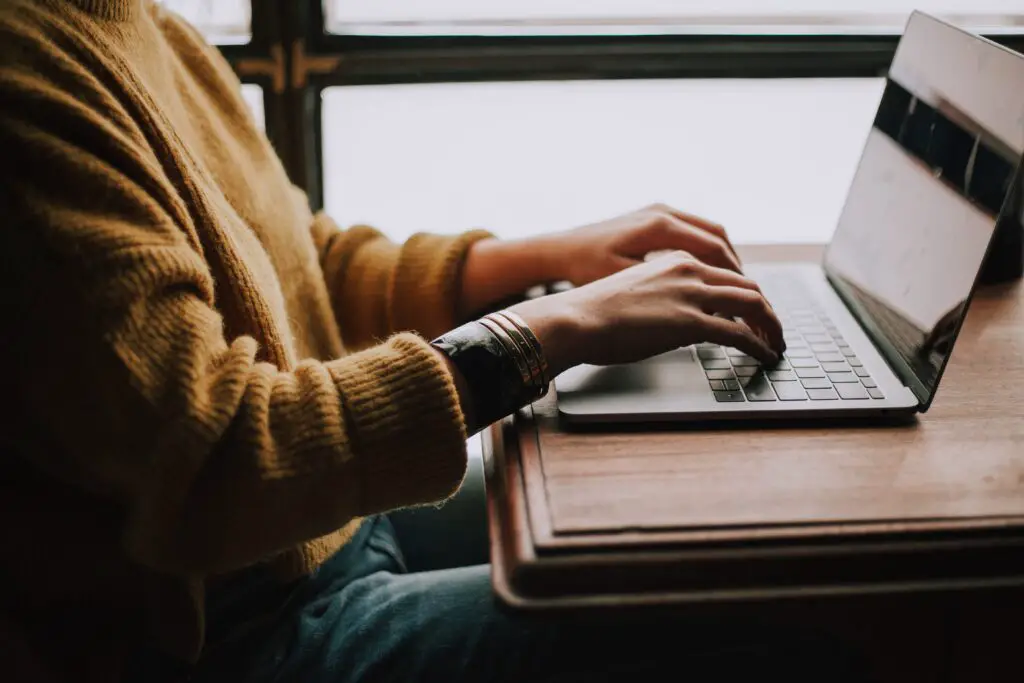
Solution 1: [SOLVED] TypeError: Only Size-1 Arrays Can Be Converted To Python Scalars
We use the.vectorize function in the first method. The.vectorize function is basically a for loop that takes an array and returns a single numpy array.
We go through the array x one by one with this method and return a single value. So, we don’t get the “only arrays with size 1 can be turned into Python scalars” typeerror.
Code and Explanation
import numpy as ny
import matplotlib.pyplot as matplot
xcord = ny.arange(1, 5, 2.5)
ycord = ny.vectorize(ny.int)
matplot.plot(xcord, ycord(xcord))
matplot.show()
As you can see, we no longer need to define a function because we used the.int function inside the.vectorize function, which loops through the array and returns a single array that the.int function can use.
Solution 1 Output
This will be the output of the given solution above.
Solution 2
I also found a method that uses the.astype method. This method “casts” an array into a certain type, which in our case is “int.”
Even though this method works, I prefer the first one because this method casts a string into an int, which is not a good thing to do.
Code and Explanation
import numpy as ny
import matplotlib.pyplot as matplot
def ycord(xcord):
return xcord.astype(int)
xcord = ny.arange(1, 5, 2.5)
matplot.plot(xcord, ycord(xcord))
matplot.show()
When you get the Typeerror: message, you can do one of these two things. Only arrays of size 1 can be turned into Python scalars.
Conclusion
Numpy module has given us thousands of helpful ways to solve hard problems quickly. Each of these methods has its own set of rules and parameters that we must follow.
The Only Size 1 Arrays Python Error shows up when you give a function that takes a scalar value an invalid data type. By using the suggestions and solutions in the post, you can fix this error right away.
Recommendation
For recommendation, If you want to explore your knowledge in programming especially python, I have here the complete course for Python Tutorials for Beginners.
Inquires
However, If you have any questions or suggestion about the tutorial to solve TypeError: Only Size-1 Arrays Can Be Converted To Python Scalars, Please feel free to comment below, Thank You!