With the help of examples, we will learn about the many types of operators in C++ in this tutorial. An operator in programming is a symbol that performs operations on a value or variable.
Symbols that execute operations on variables and values are known as operators overloading. For instance, the addition operator + is used for addition, while the subtraction operator – is used for subtraction.
There are six different types of operators in C++ |
---|
1. Arithmetic Operators |
2. Assignment Operators |
3. Relational Operators |
4. Logical Operators |
5. Bitwise Operators |
6. Other Operators |
Arithmetic Operators in C++
To perform arithmetic operations on variables and data, arithmetic operators are used. As an example,
x + y;
The + operator is used to combine two variables, x and y. In C++, there are a variety of different arithmetic operators.
Assume variable A has a value of 12 and variable B has a value of 15.
Operator | Meaning of Operator | Description | Example |
---|---|---|---|
+ | addition or unary plus | Adds two operands to the equation. | X + Y= 27 |
– | subtraction or unary minus | The second operand is subtracted from the first. | X – Y= -3 |
* | multiplication | Both operands are multiplied. | X * Y= 180 |
/ | division | The numerator is divided by the denominator | Y / X = 1 |
% | remainder after division (modulo division) | After an integer division, the remainder of the modulus operator. | Y % X = 3 |
++ | Increases Increment | The increment operator adds one to the integer value. | X++ = 13 |
— | Decreases Increment | The increment operator reduces the value of an integer by one. | Y– = 14 |
Examples of Arithmetic Operators
#include <iostream>
using namespace std;
int main() {
int x, y;
x = 12;
y = 15;
// printing the sum of x and y
cout << "x + y = " << (x + y) << endl;
// printing the difference of x and y
cout << "x - y = " << (x - y) << endl;
// printing the product of x and y
cout << "x * y = " << (x * y) << endl;
// printing the division of x by y
cout << "x / y = " << (y / x) << endl;
// printing the modulo of x by y
cout << "x % y = " << (y % x) << endl;
return 0;
}
Output:
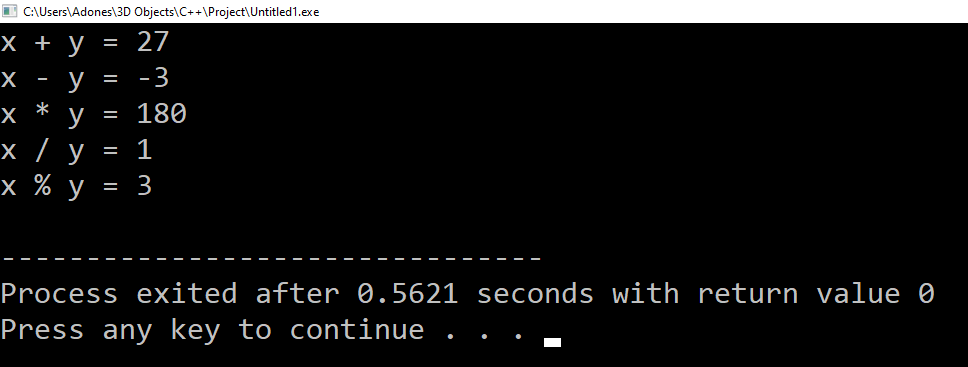
Assignment Operators in C++
Assignment operators are used to give a variable a value. The assignment operator’s left-side operand is a variable, while the assignment operator’s right-side operand is a value. Otherwise, the compiler will throw an error if the value on the right side is not the same data type as the variable on the left side(Operator Function in C++).
An Example of Assignment Operators
#include <iostream> using namespace std; int main() { int x, y; // 2 is assigned to x x = 43; // 7 is assigned to y y = 72; cout << "x = " << x << endl; cout << "y = " << y << endl; cout << "\nAfter x += y;" << endl; // assigning the sum of x and y to x x += y; // x = x +y cout << "x = " << x << endl; return 0; }
Output
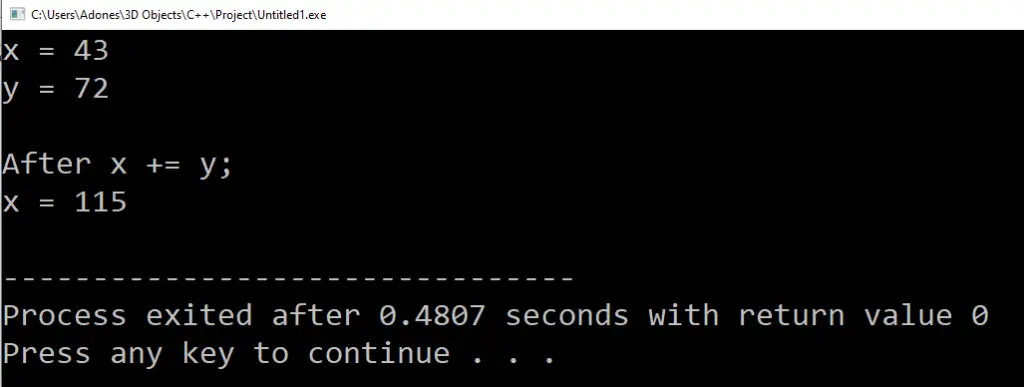
Relational Operators in C++
To check the relationship between two operands, a relational operator is utilized.
An Example for Relational Operators
#include <iostream> using namespace std; int main() { int x, y; x = 5; y = 9; bool result; result = (x == y); // false cout << "5 == 9 is " << result << endl; result = (x != y); // true cout << "5 != 9 is " << result << endl; result = x > y; // false cout << "5 > 9 is " << result << endl; result = x < y; // true cout << "5 < 9 is " << result << endl; result = x >= y; // false cout << "5 >= 9 is " << result << endl; result = x <= y; // true cout << "5 <= 9 is " << result << endl; return 0; }
Output
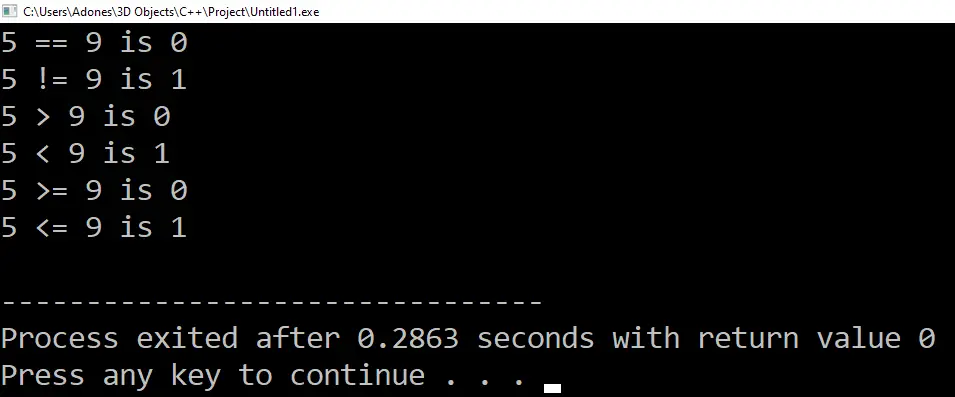
Logical Operators in C++
Logical operators are utilized to determine whether an expression is true or false. If the expression is true, it will return 1, but if it is false, it will return 0.
Operator | Description | Example |
---|---|---|
&& | It’s known as the logical AND operator. The condition is true if both operands are non-zero. | (A && B) is false. |
|| | The Logical OR Operator is a term used to describe a type of logic. The condition becomes true if any of the two operands is non-zero. | (A || B) is true. |
! | It’s known as the Logical NOT Operator. Its operand’s logical state is reversed using it. If a condition is true, the Logical NOT operator will turn it into a false condition. | !(A && B) is true. |
Bitwise Operators in C++
Bitwise operators are used in C++ to execute operations on single bits. They’re only compatible with char and int data types.
Operator | Description |
---|---|
& | Binary AND |
| | Binary OR |
^ | Binary XOR |
~ | Binary One’s Complement |
<< | Binary Shift Left |
>> | Binary Shift Right |
Summary
An operator function in C++ symbol that operates on a variable or value is known as an operator. Operators include arithmetic, logical, conditional, relational, bitwise, and assignment operators, among others Reference operator, and other specific sorts of operators are also available in C++.