Do you want to master the JavaScript slice() method with strings? Read on!
In this article, you’ll learn how to extract substrings from a string, understand how start and end indices work, and see practical examples.
Also, you will discover how to slice() returns a new string while leaving the original intact.
This guide is perfect for beginners and a great refresher for seasoned developers!
What is string slice method in JavaScript?
The slice() method in JavaScript is a built-in function used to extract and return a portion or “slice” of the provided input string.
How does it work?
✔ You determine the start and end points of the substring you wish to extract.
✔ The method then gives you a fresh string that contains that substring.
✔ If the starting point is equal to or larger than the string’s length, you’ll get an empty string in return.
✔ If the starting point is negative, it’s taken from the end of the string.
✔ If you leave out the ending point or if it’s equal to or larger than the string’s length, slice() will pull out everything from the starting point to the end of the string.
✔ If the ending point is negative, it’s taken from the end of the string.
Syntax
string.slice(startIndex, endIndex ) ✅
Parameters
startIndex✅ (required)
The start index is zero-based, and this is where the extraction started. In simple understanding, this is where you want to start slicing the string.
It’s an integer that represents the index of the character where the slice starts.
Here’s an example:
const samplestring = "Itsourcecode";
const substring = samplestring.slice(2); ✅
console.log({ substring });
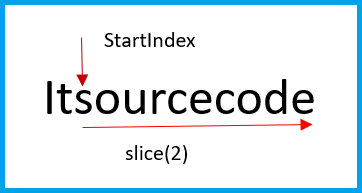
Output:
{ substring: 'sourcecode' }
Here’s another example if the startIndex is negative:
const samplestring = "Itsourcecode";
const substring = samplestring.slice(-2); ✅
console.log({ substring });
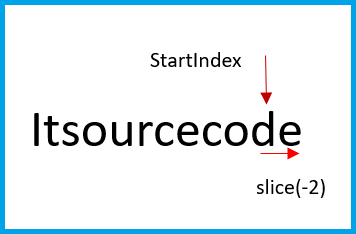
Output:
{ substring: 'de' }
Let’s proceed to our second parameter:
endIndex ✅ (optional)
The endIndex is zero-based works like a stopping point for the slice() method. This counting starts from the beginning of the string.
This is where you want to stop slicing the string. It’s an integer that represents the index of the character where the slice ends.
Here’s an example:
const samplestring = "Itsourcecode";
const substring = samplestring.slice(0, 12);
console.log({ substring });
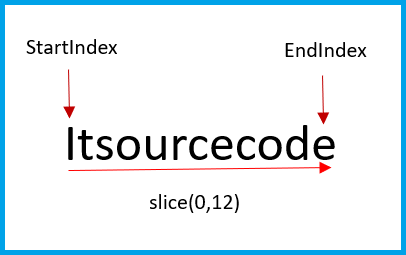
Output:
{ substring: 'Itsourcecode' }
Here’s another example if the endIndex is negative.
const samplestring = "Itsourcecode";
const substring = samplestring.slice(0,-4);
console.log({ substring });
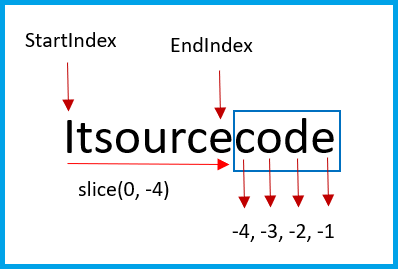
Output:
{ substring: 'Itsource' }
📌Take note: If startIndex or endIndex are negative, the values are taken from the end of the string or it is counted from backward.
Return value
The slice() method returns a new string that contains the extracted section. It doesn’t change the original string.
Different examples using string slice() method in JavaScript
Here are some examples of using the string slice() method in JavaScript:
Example 1: Get the first word
const samplestring = "Hi, Welcome to Itsourcecode.com";
let greeting = samplestring.slice(0, 2); ✅
console.log(greeting);
Output:
Hi
Example 2: Get the welcome message
const samplestring = "Hi, Welcome to Itsourcecode.com";
let welcomeMessage = samplestring.slice(4, 11); ✅
console.log(welcomeMessage);
Output:
Welcome
Example 3: Get the website name
const samplestring = "Hi, Welcome to Itsourcecode.com";
let websiteName = samplestring.slice(15); ✅
console.log(websiteName);
Output:
Itsourcecode.com
Example 4: Get the whole string
const samplestring = "Hi, Welcome to Itsourcecode.com";
let websiteName = samplestring.slice(0, 31);
console.log(websiteName);
Output:
Hi, Welcome to Itsourcecode.com
Those are the example codes using the string slice() method with their respective output.
As mentioned earlier, the slice() method doesn’t modify the original string but returns a new one.
The start and end indices are zero-based, and negative indices count from the end of the string.
Conclusion
In conclusion, the slice() method in JavaScript is a one of the useful tool for extracting substrings from a given input string.
By specifying start and end indices, you can obtain a fresh string containing the desired portion.
We are hoping that this article provides you with enough information that helps you understand the slice string JavaScript.
If you want to dive into more JavaScript topics, check out the following articles:
Thank you for reading Itsourcecoders 😊.