Unions in C Programming Language, a data type called a union, enables the storage of many data types in the same memory regions.
Only one union member can be accessed at a time, making it an effective technique to reuse the memory location.
A union can be declared and used almost identically to a structure.
What is Union in C?
A Union C is a user-defined type analogous to C structs.
In contrast to unions, which can only contain one member value at a time, structures allot adequate room to store all of its members.
Need for C Union
- Memory can be saved by using C unions. Consider a union as a section of memory that is used to store variables of various types in order to comprehend it better. The current data gets replaced with new data when we want to assign a new value to a field.
- Data members that are mutually exclusive can share memory thanks to C unions. When memory is valuable, as it is in embedded systems, this is quite significant. Unions are frequently utilized in embedded programming when direct memory access is required.
Difference between Structure and Union
The primary distinction between a union and a structure is that:
- The space allotted by structures allows them to store all of their fields. The first one is stored at the struct’s beginning, the second one is stored after that, and so on.
- Unions store all fields in the same location and only allot enough space to store the largest field that is listed.
What are unions in c?
Defining a Union C is similar to how you did when defining a structure, you must use the union statement to define a union.
For your software, the union statement creates a new data type with several members.
The union statement is formatted as follows:
union [union tag] { member definition; member definition; ... member definition; } [one or more union variables];
Each member definition is a typical variable definition, such as int I float f, or any other acceptable variable definition, and the union tag is optional.
It is optional to specify one or more union variables before the last semicolon at the end of the union specification.
The definition of a union type named Data with the three members a, ft, and str is given below.
union Data { int a; float ft; char str[20]; } data;
Now, a Data type variable can hold a string of characters, a floating-point value, or an integer.
It indicates that several types of data can be stored in a single variable, i.e., the same memory location.
Depending on your needs, you can utilize any built-in or user-defined data types inside a union.
Accessing C Unions Members
We employ the member access operator to gain access to any union member (.).
Between the name of the union variable and the union member that we want to access, the member access operator is coded as a period.
To define variables of the union type, use the term union. The example that follows demonstrates how to use unions in a program.
#include <stdio.h> #include <string.h> union Data { int a; float b; char str[200]; }; int main( ) { union Data data_usage; data_usage.a = 10; data_usage.b = 220.5; strcpy( data_usage.str, "C Programming Language Tutorials"); printf( "data_usage.a : %d\n", data_usage.a); printf( "data_usage.b : %f\n", data_usage.b); printf( "data_usage.str : %s\n", data_usage.str); return 0; }
Output of the above example:
data_usage.a : 1917853763
data_usage.b : 4122360580327794860452759994368.000000
data_usage.str : C Programming Language Tutorials
Here, we can see that the values of the I and f members of the union were corrupted since the memory region was taken up by the final value allocated to the variable, which is why the value of the str member is being printed so clearly.
You can test the above example here! ➡ C Online Compiler
Summary
We have learned about C unions, their syntax, and their usage in this C tutorial. Additionally, we discovered how C unions differ from C structures.
If you have any questions or suggestions about a Union C Programming Language with definitions and Examples, please feel free to leave a comment below.
About Next Tutorial
In the next post, I’ll create a Bit Fields in C and try to explain its many components in full detail.
I hope you enjoy this post on Union in C Programming Language with Definition and Examples, in which I attempt to illustrate the language’s core grammar.
PREVIOUS
NEXT
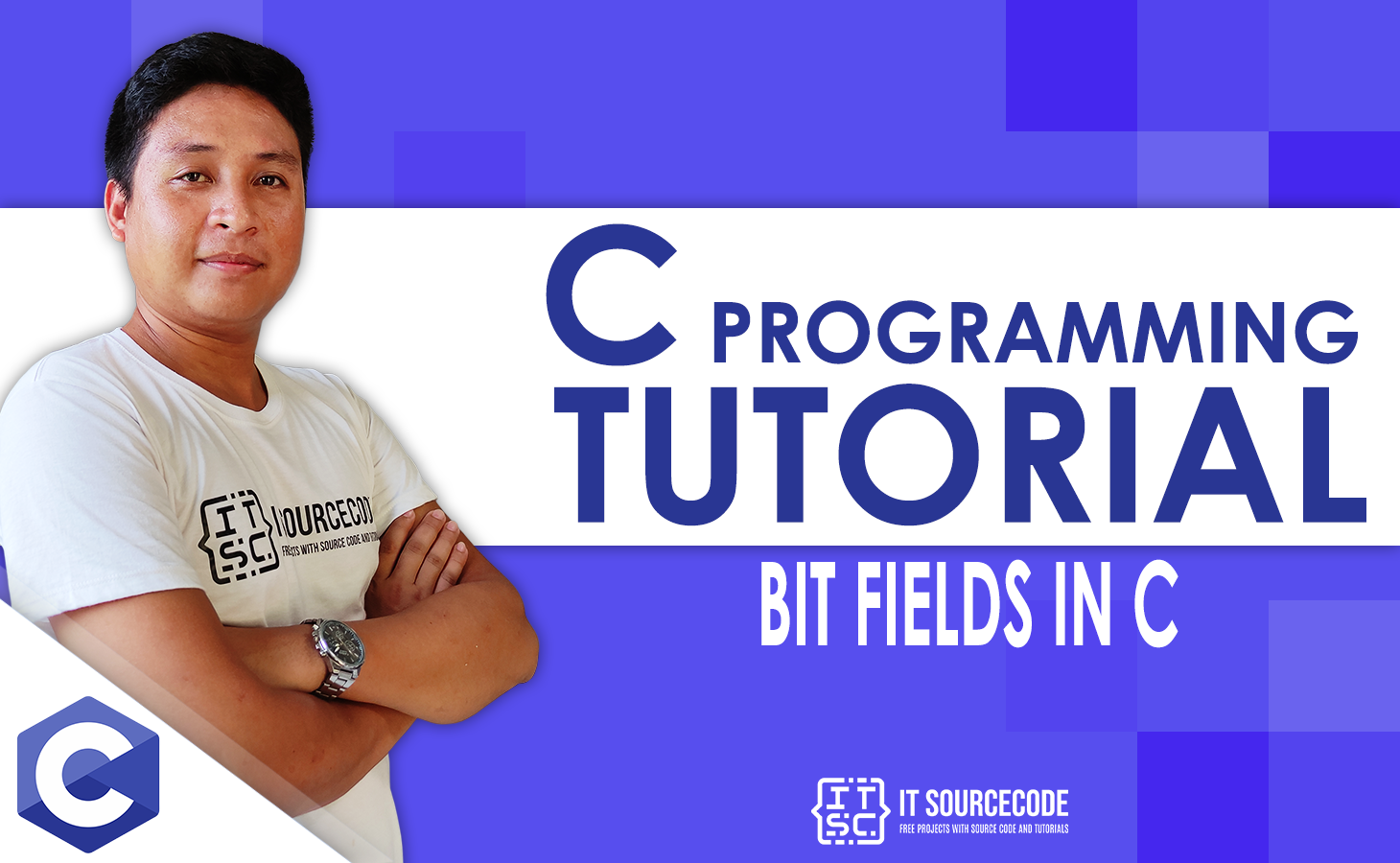
Bit Fields in C