TypeScript has several types of decision-making statements. These statements are based on conditions that the program checks.
In decision-making structures, the programmer must define at least one condition for the program to assess.
If the condition is true, a set of statements are executed. If it is false, a different set of statements is executed.
Here are the different types of Decision-making in TypeScript:
- if statement
- if-else statement
- if-else-if ladder
- nested if statement
Here is an example of a typical structure of decision-making statements in TypeScript.
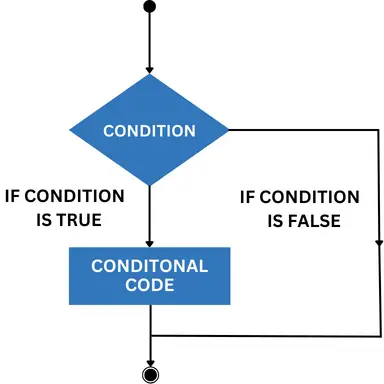
Types of Decision-making Statements in TypeScript
Here are the different types of Decision-making in TypeScript:
1. If Statement
This is a basic way of making decisions. It determines if the statements will be run or not by evaluating the condition and returning true if the condition is met.
Syntax:
if(condition) {
// code to be executed
}
If Statement Flow Diagram
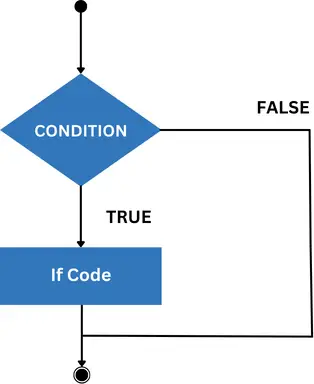
Example
let x: number = 10;
if (x > 5) {
console.log("x is greater than 5");
}
You can test the above example here! ➡TyspeScript Online Compiler
Output:
x is greater than 5
2. If-else statement
An if statement only produces a result when its condition is met. However, if we want to have an output even when the condition isn’t met, we should use an if-else statement.
The if-else statement evaluates the condition. If the condition is true, it runs the code in the if block, and if the condition is false, it runs the code in the else block.
Syntax
if(condition) {
// code to be executed
} else {
// code to be executed
}
If Statement Flow Diagram
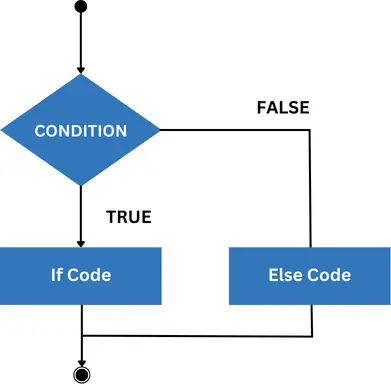
Example
let x: number = 10;
if (x > 5) {
console.log("x is greater than 5");
} else {
console.log("x is not greater than 5");
}
You can test the above example here! ➡TyspeScript Online Compiler
Output:
x is greater than 5
3. if-else-if ladder
In this scenario, a user can choose from several alternatives. The execution begins from the top and proceeds downwards.
If a condition is met, the corresponding statement is executed and the remaining conditions are skipped.
If no condition is found to be true, the final ‘else’ statement is returned.
Syntax
if(condition1){
//code to be executed if condition1 is true
}else if(condition2){
//code to be executed if condition2 is true
}
else if(condition3){
//code to be executed if condition3 is true
}
else{
//code to be executed if all the conditions are false
}
if-else-if ladder Statement Flow Diagram
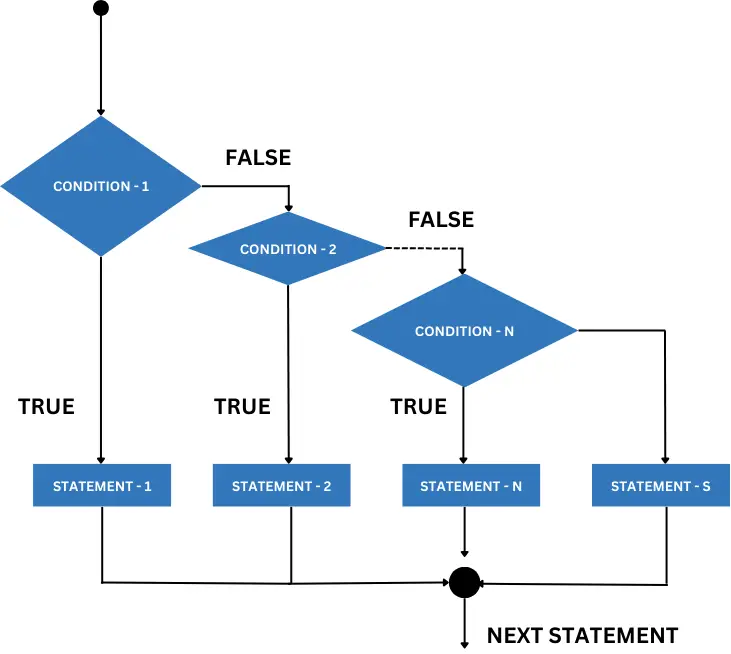
Example
let value = 15;
if (value < 10) {
console.log("Value is less than 10");
} else if (value >= 10 && value < 20) {
console.log("Value is between 10 and 20");
} else if (value >= 20 && value < 30) {
console.log("Value is between 20 and 30");
} else {
console.log("Value is 30 or more");
}
You can test the above example here! ➡TyspeScript Online Compiler
Output:
Value is between 10 and 20
4. nested if statement
A nested if statement refers to an if statement that is contained within the body of another if or else statement.
Syntax
if(condition1) {
//Nested if else inside the body of "if"
if(condition2) {
//Code inside the body of nested "if"
}
else {
//Code inside the body of nested "else"
}
}
else {
//Code inside the body of "else."
}
nested if statement Flow Diagram
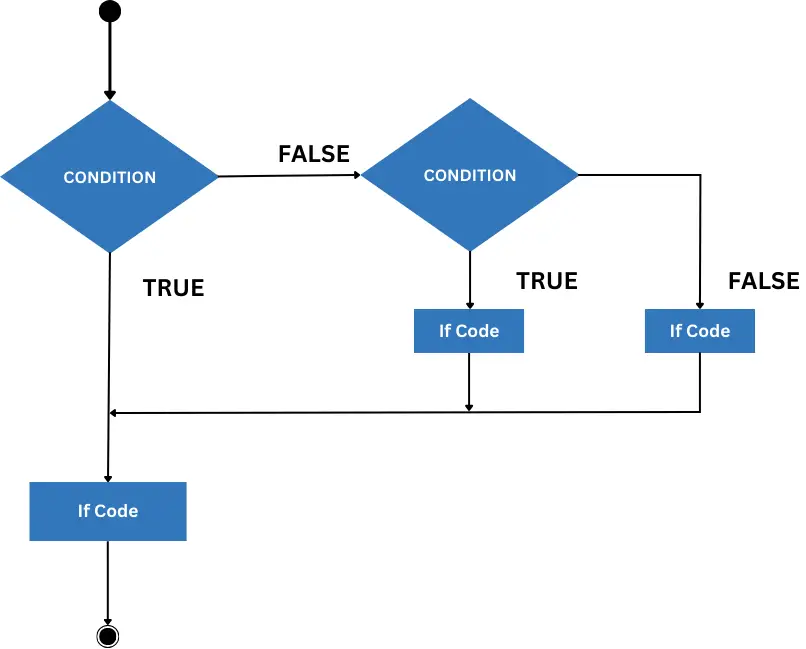
Example
let value = 25;
if (value > 10) {
console.log("Value is greater than 10");
if (value < 20) {
console.log("Value is less than 20");
} else if (value >= 20 && value < 30) {
console.log("Value is between 20 and 30");
} else {
console.log("Value is 30 or more");
}
} else {
console.log("Value is 10 or less");
}
You can test the above example here! ➡TyspeScript Online Compiler
Output:
Value is greater than 10
Value is between 20 and 30
Conclusion
Now that you have gained knowledge about decision-making statements in TypeScript, you are on the verge of developing more robust and efficient code.
Begin to incorporate all the if-else statements you have learned today into your programming.
I hope that this tutorial helped you understand how to use decision-making statements in TypeScript.
If you have any questions or inquiries, please don’t hesitate to leave a comment below.