In this article, we will learn about perfect number in Java. This article also has the best program examples to check whether the number is perfect or not.
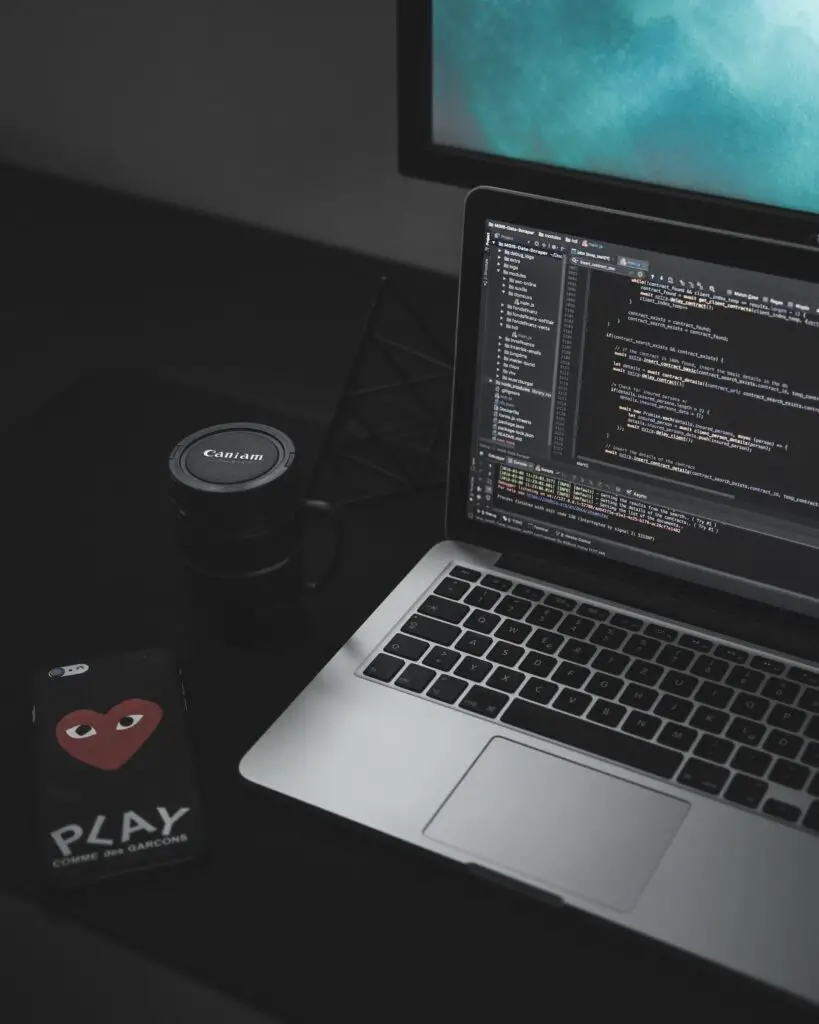
Any number may qualify as a Java Perfect Number if the total of its positive factors, excluding the number itself, equals the value of the number.
What is a perfect number in Java
In Java, a perfect number is one whose factors, minus the number itself, add up to the number itself. In other words, a number is called “perfect
” if the sum of its positive divisors, minus the number itself, equals the number itself. Let’s use an example to understand it.
For example:
28
is a perfect number because it can be divided by 1
, 2
, 4
, 7
, 14
, and 28
, and the total of these numbers is 28
. Don’t forget that we need to leave out the number itself. We didn’t add 28 because of this. Java perfect numbers include 6
, 28
, 496
, 8128
, 33550336,
and so on.
How do you calculate a perfect number?
A number is perfect if the sum of its proper divisors equals the sum itself. To find the correct factors of a number, write down all the numbers that divide the number except the number itself. If the total of the factors is 28, then 28 is a perfect number.
7 Ways to find the perfect number
The following are different ways in a Java program to check if a number is perfect or not.
- Using method
- Using for loop
- Using while loop
- Using functions
- Using recursion
- Using array
- Using OOPS (Object Oriented Programming)
Example program using method
import java.util.Scanner;
public class usingMethod
{
//function that checks if the given number is perfect or not
static long isPerfect(long num)
{
//variable stores the sum
long sum = 0;
//executes until the condition becomes false
for (int i = 1; i <= num / 2; i++)
{
if (num % i == 0)
{
//calculates the sum of factors
sum = sum + i;
} //end of if
} //end of for
//returns the sum of factors
return sum;
} //end of method
public static void main(String args[])
{
long number, s;
Scanner sc = new Scanner(System.in);
System.out.print("Enter the number: ");
//reads a number from the user
number = sc.nextLong();
//calling the function
s = isPerfect(number);
//compares sum with the number
if (s == number)
System.out.println(number + " is a perfect number");
else
System.out.println(number + " is not a perfect number");
}
}
Output:
Enter the number: 26 is not a perfect number
Example program using for loop
// Write Perfect Number program in Java using For Loop */
import java.util.Scanner;
public class PerfectNumberUsingFor {
private static Scanner sc;
public static void main(String[] args) {
int i, Number, Sum = 0;
sc = new Scanner(System.in);
System.out.println("\n Please Enter any Number: ");
Number = sc.nextInt();
for (i = 1; i < Number; i++) {
if (Number % i == 0) {
Sum = Sum + i;
}
}
if (Sum == Number) {
System.out.format("\n% d is a Perfect Number", Number);
} else {
System.out.format("\n% d is NOT a Perfect Number", Number);
}
}
}
Output:
28 is a Perfect Number
Example program using while loop
This example program using a while loop lets the user enter any number. Using this number, the while loop will check if the user’s input is a perfect number or not.
import java.util.Scanner;
public class PerfectNumberUsingWhile {
private static Scanner sc;
public static void main(String[] args) {
int i = 1, Number, Sum = 0;
sc = new Scanner(System.in);
System.out.println("Please Enter any Number: ");
Number = sc.nextInt();
while (i < Number) {
if (Number % i == 0) {
Sum = Sum + i;
}
i++;
}
if (Sum == Number) {
System.out.format("%d is a Perfect Number", Number);
} else {
System.out.format("%d is NOT a Perfect Number", Number);
}
}
}
Output:
28 is a Perfect Number
Example program using functions
The user can enter any integer value into this program, and we’ll send that value to the method we created.
This program will use the For Loop in this user-defined function to check if the user input is a perfect number.
import java.util.Scanner;
public class PerfectNumberUsingMethod {
private static Scanner sc;
public static void main(String[] args) {
int Number;
sc = new Scanner(System.in);
System.out.println("Please Enter any Number: ");
Number = sc.nextInt();
PerfectNumber(Number);
}
public static void PerfectNumber(int Number) {
int i, Sum = 0;
for (i = 1; i < Number; i++) {
if (Number % i == 0) {
Sum = Sum + i;
}
}
if (Sum == Number) {
System.out.format("%d is a Perfect Number", Number);
} else {
System.out.format("%d is NOT a Perfect Number", Number);
}
}
}
Output:
Please Enter any Number: 496 is a Perfect Number
Our function is designated as void in this instance and has no return value. We therefore refer to it as a function.
PerfectNumber (Number);
In the main() program, the Javac compiler will instantly jump to the following method when it hits the PerfectNumber(Number) line:
public static void PerfectNumber (int Number) {
Example program using OOPS (Object-Oriented Programming)
Object-Oriented Programming is used to divide the code in this program. First, we’ll make a class with a method for finding the sum of factors.
package FrequentPrograms;
public class PerfectNumber {
int i, Sum = 0;
public int FindPerfectNumber(int Number) {
for (i = 1; i < Number; i++) {
if (Number % i == 0) {
Sum = Sum + i;
}
}
return Sum;
}
}
We will make an instance of the above class and call the methods within the main program.
package FrequentPrograms;
import java.util.Scanner;
public class PerfectNumberUsingClass {
private static Scanner sc;
public static void main(String[] args) {
int Number, Sum = 0;
sc = new Scanner(System.in);
System.out.println("Please Enter any Number: ");
Number = sc.nextInt();
PerfectNumber pn = new PerfectNumber();
Sum = pn.FindPerfectNumber(Number);
if (Sum == Number) {
System.out.format("%d is a Perfect Number", Number);
} else {
System.out.format("%d is NOT a Perfect Number", Number);
}
}
}
Output:
Please Enter any Number: 496 is a Perfect Number
Example program using recursion
public class Main
{
static int number = 28;
static int sum = 0;
static int div = 1;
static int findPerfect(int number, int div) {
{
if (div <= number / 2)
{
if (number % div == 0)
{
sum += div;
}
div++;
findPerfect(number, div);
}
return sum;
}
}
public static void main(String args[])
{
int result = findPerfect(number, div);
if (result == number)
System.out.println("It is Perfect Number");
else System.out.println("It is not Perfect Number");
}
}
Output:
It is Perfect Number
Example program using array
public class PIES {
// Function to return the sum of
// all the proper factors of n
static int sumOfFactors(int n)
{
int sum = 0;
for (int f = 1; f <= n / 2; f++) {
// f is the factor of n
if (n % f == 0) {
sum += f;
}
}
return sum;
}
// Function to return the required sum
static int getSum(int[] arr, int n)
{
// To store the sum
int sum = 0;
for (int i = 0; i < n; i++) {
// If current element is non-zero and equal
// to the sum of proper factors of itself
if (arr[i] > 0 && arr[i] == sumOfFactors(arr[i])) {
sum += arr[i];
}
}
return sum;
}
// Driver code
public static void main(String[] args)
{
int[] arr = { 18, 10, 6, 6, 4 };
int n = arr.length;
System.out.print(getSum(arr, n));
}
}
Output:
12
Example program to find the perfect number between 1 and 1000
The user can put in the minimum and maximum values in this program. This Java program finds the perfect number between the minimum and maximum values.
// Java Program to Find Perfect Number between 1 to 1000
import java.util.Scanner;
public class PerfectNumbersbetweenMin {
private static Scanner sc;
public static void main(String[] args) {
int i, Number, Minimum, Maximum, Sum = 0;
sc = new Scanner(System.in);
System.out.println("Please Enter the Minimum Value: ");
Minimum = sc.nextInt();
System.out.println("Please Enter the Maximum Value: ");
Maximum = sc.nextInt();
//sum n system.out.println
for (Number = Minimum; Number <= Maximum; Number++) {
for (i = 1, Sum = 0; i < Number; i++) {
if (Number % i == 0) {
Sum = Sum + i;
}
}
if (Sum == Number) {
System.out.format("%d \t", Number);
}
}
}
}
Output:
Please Enter the Minimum Value: 1
Please Enter the Maximum Value: 20000
6 28 496 8128
Example program for perfect number inside a given range
The following program locates all the perfect integers that fall within a certain range.
public class PerfectNumberExample3 {
//function checks if the given number is perfect or not
static boolean isPerfectNumber(int num) {
//variable stores the sum of divisors
int sum = 1;
//determines all the divisors of the given number and adds them
for (int i = 2; i * i <= num; i++) {
if (num % i == 0) {
if (i * i != num)
sum = sum + i + num / i;
else
//calculates the sum of factors
sum = sum + i;
} //end of if
} //end of for
if (sum == num && num != 1)
//returns true if both conditions (above) returns true
return true;
//returns false if any condition becomes false
return false;
} //end of function
//driver code
public static void main(String args[]) {
System.out.println("Perfect Numbers between 2 to 10000 are: ");
//loop executes until the condition n<10000 becomes false
for (int n = 2; n < 10000; n++)
//calling function
if (isPerfectNumber(n))
//prints all perfect number between given range
System.out.println(n + " is a perfect number");
}
}
Output:
Perfect Numbers between 2 to 10000 are:
6 is a perfect number
28 is a perfect number
496 is a perfect number
8128 is a perfect number
What are the perfect numbers from 1 to 100?
There are only two perfect numbers from 1 to 100. These are 6
and 28
.
Why is 496 a perfect number?
In mathematics, 496
is most famous for being a perfect number, and it was one of the first numbers for which this was known. As a perfect number, it is equal to the Mersenne prime 31, 25 1, with 24 (25 1) equaling 496.
What are the perfect numbers from 1 to 10000?
Nicomachus noticed around 100 C.E. that perfect numbers strike a balance between extremes of excess and deficiency, like when the sum of a number’s positive divisors, excluding the number, is too big or too small, and falls in the “right” order. The only perfect numbers between 1, 10, 100, 1000, and 10000 are 6, 28, 496, and 8128.
What is the importance of perfect numbers?
Perfect numbers provide a solid number theory illustration of the principle of classification in the Fibonacci series.
Conclusion
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials which could help you a lot.