When we are working with arrays in Python, it is not inevitable that we may encounter an error that can interrupt our code’s execution. One of the errors is the ValueError: Setting an array element with a sequence.
This error typically occurs when we attempt to assign a sequence (such as a list or another array) to a single element in a NumPy array, but the shapes of the objects doesn’t match.
In this article, we will discuss its causes and significance. Also, we will provide clear examples to explain the issue and show practical solutions to resolve it.
By the end, you will have a solid understanding of how to solve the ValueError Setting an array element with a sequence and ensuring smooth execution of your Python code.
How the Error Occur?
Let’s try some examples to learn a better understanding of the ValueError: Setting an array element with a sequence.
Example 1: Assigning a list to a numpy array element:
import numpy as np
arr = np.array([1, 2, 3])
arr[0] = [4, 5, 6]
In this example, we are trying to assign a list [4, 5, 6] to the first element of the numpy array arr.
However, numpy arrays are homogeneous, meaning they can only hold elements of a single data type.
Since the element being assigned is a sequence (a list), which violates the homogeneous property, a ValueError will be raised.
Traceback (most recent call last):
File “C:\Users\Dell\PycharmProjects\Python-Code-Example\main.py”, line 4, in
arr[0] = [4, 5, 6]
ValueError: setting an array element with a sequence.
Example 2: Assigning a tuple to a numpy array element
import numpy as np
arr = np.zeros((2, 2))
arr[0, 0] = (1, 2)
In this case, we have a 2×2 numpy array arr filled with zeros. We are attempting to assign a tuple (1, 2) to the element at index (0, 0) of the array.
Since tuples are sequences, the error “Setting an array element with a sequence” will occur because numpy arrays require scalar values.
Traceback (most recent call last):
File “C:\Users\Dell\PycharmProjects\Python-Code-Example\main.py”, line 4, in
arr[0, 0] = (1, 2)
ValueError: setting an array element with a sequence.
How to Solve the Valueerror: setting an array element with a sequence?
To solve the valueerror: setting an array element with a sequence, here are the following solutions.
Solution 1: Assigning a list to a numpy array element
If you want to assign a sequence of values to a numpy array element, you can use slicing instead.
Here’s an updated solution:
import numpy as np
arr = np.array([1, 2, 3])
arr[0:3] = [4, 5, 6]
print(arr)
Output:
[4 5 6]
In this example, we use slicing arr[0:3] to assign the values [4, 5, 6] to the first three elements of the array.
This is to prevent the ValueError by operating on a slice rather than a single element.
Solution 2: Assigning a tuple to a numpy array element
To solve the error is to assign a tuple to a numpy array element, you need to convert the tuple to a scalar value using indexing or by accessing a specific element of the tuple.
Here’s an updated solution:
import numpy as np
arr = np.zeros((2, 2))
arr[0, 0] = (1, 2)[0]
print(arr)
Output:
[[1. 0.]
[0. 0.]]
In this example, we access the first element of the tuple (1, 2) by indexing it with [0].
Then we assign this scalar value 1 to the element at index (0, 0) of the numpy array arr.
By converting the tuple to a scalar, we can avoid the ValueError.
Solution 3: Reshaping the Sequence
To fix this error is to reshape the sequence. If the sequence has a different shape than the target array element, you can reshape it to match the desired shape.
You can use the NumPy reshape() function to adjust the dimensions accordingly.
import numpy as np
# Original sequence
sequence = [1, 2, 3, 4, 5, 6]
# Reshaping the sequence
reshaped_sequence = np.reshape(sequence, (2, 3))
# Output
print("Original sequence:")
print(sequence)
print("Reshaped sequence:")
print(reshaped_sequence)
Output:
Original sequence:
[1, 2, 3, 4, 5, 6]
Reshaped sequence:
[[1 2 3]
[4 5 6]]
In this example, the original sequence [1, 2, 3, 4, 5, 6] is reshaped into a 2×3 array using the np.reshape() function.
The resulting reshaped sequence is [[1, 2, 3], [4, 5, 6]].
Solution 4: Modifying Data Types
When encountering a ValueError due to incompatible data types, you can change the data types to match.
Use the astype() method to convert the sequence to the proper data type.
import pandas as pd
# Create a sample DataFrame with mixed data types
data = {'Name': ['John', 'Alice', 'Mike', 'Linda'],
'Age': ['25', '30', '35', '40'],
'Salary': ['2500', '3000', '3500', '4000']}
df = pd.DataFrame(data)
# Print the initial DataFrame
print("Initial DataFrame:")
print(df)
print()
# Convert 'Age' and 'Salary' columns to numeric data types
try:
df['Age'] = df['Age'].astype(int)
df['Salary'] = df['Salary'].astype(float)
except ValueError as e:
print(f"ValueError: {e}")
print("Unable to convert the data types.")
print()
# Print the modified DataFrame after type conversion
print("Modified DataFrame:")
print(df)
Output:
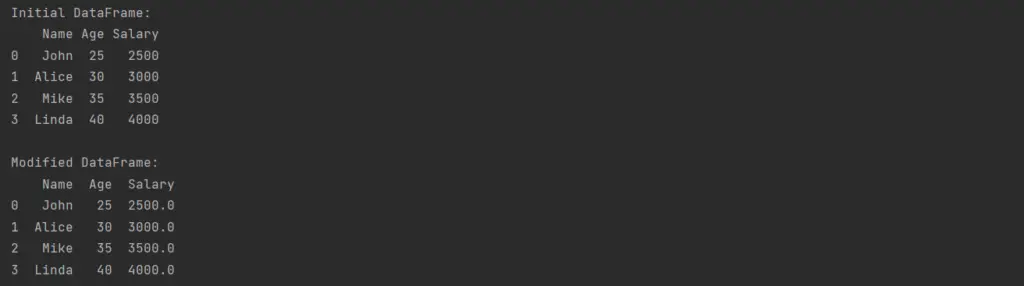
Solution 5: Adjusting Array Dimensions
When dealing with the non matching dimensions, you can adjust the dimensions of the target array or sequence to match each other.
Utilize the NumPy indexing and slicing techniques to modify the dimensions accordingly.
For example:
import numpy as np
# Create two arrays with different dimensions
arr1 = np.array([1, 2, 3]) # 1-dimensional array
arr2 = np.array([[4, 5, 6], [7, 8, 9]]) # 2-dimensional array
# Adjust dimensions of arr1 to match arr2
arr1_adjusted = arr1[:, np.newaxis] # Add a new axis to arr1
# Perform an operation with the adjusted arrays
result = arr1_adjusted + arr2
print(result)
Output:
[[ 5 6 7]
[ 8 9 10]]
Frequently Asked Questions (FAQs)
The ValueError Setting an array element with a sequence is an error that occurs when you attempt to assign a sequence (such as a list or another array) to a single element in a NumPy array, but the shapes of the objects do not match.
The ValueError occurs when you try to assign a sequence with incompatible dimensions or data types to a single element in a NumPy array.
Yes, you can assign a list to a single element in a NumPy array as long as the list and the array have compatible shapes and data types.
Conclusion
In conclusion, we’ve discussed on how errors occur and also provided practical solutions that can help you to understand and easily fix the error.