Have you ever wondered what makes TypeScript so powerful? Well, one of the key features is its arithmetic operators.
These operators are fundamental in performing calculations and manipulating data.
In this article, we’ll dive into the world of TypeScript arithmetic operators, exploring their definitions, uses, and practical applications.
What is an Operator?
An operator in TypeScript is a symbol or keyword that carries out operations on one or more pieces of data.
The data that operators manipulate is known as operands.
As we all know TypeScript is a strongly typed extension of JavaScript, so it encompasses all the conventional operators that JavaScript supports.
What is Arithmetic Operators?
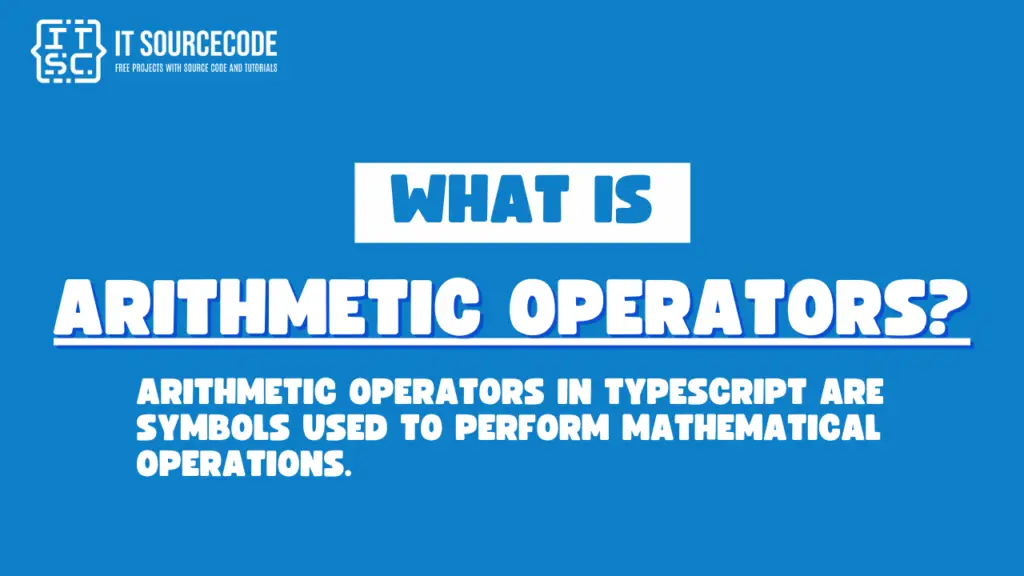
Arithmetic operators in TypeScript are symbols used to perform mathematical operations.
They are essential for tasks that involve calculations, whether it’s something as simple as adding two numbers or as complex as performing operations within an algorithm.
Arithmetic Operators:
These are used for basic math operations like addition (+), subtraction (-), multiplication (*), division (/), modulus (%), increment (++), and decrement (–).
1. Addition (+)
The + (Addition) operator is used to add two operands and will return the sum of the operands.
Syntax:
let result = operand1 + operand2;
Here’s an example:
let x: number = 30,
y: number = 20
let z: number = x + y ✅
console.log(z)
Output:
50
2. Subtraction (-)
The subtraction (-) operator subtracts the second operand from the first operand and will return the difference between the values.
Syntax:
let result = operand1 - operand2;
Here’s an example:
let x: number = 30,
y: number = 20
let z: number = x + y ✅
console.log(z)
Output:
10
3. Multiplication (*)
The Multiplication (*) operator multiplies the two operands and will return the product of the values.
Syntax:
let result = operand1 * operand2;
Here’s an example:
let x: number = 30,
y: number = 20
let z: number = x * y ✅
console.log(z)
Output:
600
4. Division (/)
The Division (/) operator divides the first operand by the second operand and returns the quotient.
Syntax:
let result = operand1 / operand2;
Here’s an example:
let x: number = 40,
y: number = 2
let z: number = x / y ✅
console.log(z)
Output:
20
5. Modulus (%)
The Modulus (%) operator performs a division of two operand and returns the remainder.
Syntax:
let result = operand1 % operand2;
Here’s an example:
let x: number = 45,
y: number = 2
let z: number = x % y ✅
console.log(z)
Output:
1
6. Increment (++)
The increment (++) operator increases the value of the variable by one. It can be used as a prefix (++operand) or postfix (operand++).
Here’s an example:
let x: number = 10
x++ ✅
console.log(x)
Output:
11
7. Decrement (–)
The decrement (++) operator decreases the value of the variable by one. It can be used as a prefix (–operand) or postfix (operand–).
Here’s an example:
let x: number = 10
x-- ✅
console.log(x)
Output:
9
Frequently Asked Questions (FAQs)
What is the difference between TypeScript and JavaScript in terms of arithmetic operations?
TypeScript and JavaScript share the same arithmetic operators and behavior. The primary difference is TypeScript’s type system, which provides additional type safety and error checking.
Can I perform arithmetic operations on non-numeric types in TypeScript?
While arithmetic operations are intended for numeric types, TypeScript can implicitly convert some non-numeric types, such as strings containing numbers, to numeric types during operations.
How does TypeScript handle division by zero?
Division by zero in TypeScript, like in JavaScript, results in Infinity or -Infinity, depending on the signs of the operands. It does not throw an error.
Conclusion
So, that’s the end of our discussion about the TypeScript arithmetic operators that provides foundation for performing calculations and manipulating data.
By mastering their use, you can write more efficient and effective code.
I hope this article has given you some insights and helped you understand the TypeScript Arithmetic Operators.
If you have any questions or inquiries, please don’t hesitate to leave a comment below.