What is TypeScript for loop?
A for loop in TypeScript is a type of definite loop that has a control structure that lets you repeat a block of code a certain number of times.
Syntax:
for (initial value, condition, step increment)
Here’s a further explanation of how the for loop works:
- Initialization:
Before the loop starts, you have to set up a variable. This is usually a counter variable.
For example:
let i = 0;
- Condition:
Condition is a test that checks if the loop should continue. If the condition is true, the loop will keep going. If it’s false, the loop will stop.
For example:
i < 10;
- Final Expression
Final Expression is a piece of code that runs after each loop. It’s often used to update the counter variable.
For example:
i++
For loop Flowchart in TypeScript
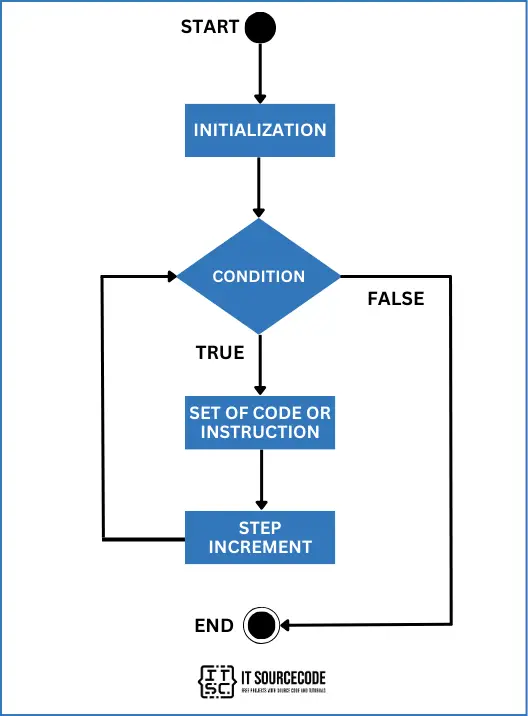
Example:
Let’s take a look at the example code below.
for (let i = 0; i < 5; i++) {
console.log("Hello, welcome to Itsourcecode!"); ✅
}
Output:
Hello, welcome to Itsourcecode!
Hello, welcome to Itsourcecode!
Hello, welcome to Itsourcecode!
Hello, welcome to Itsourcecode!
Hello, welcome to Itsourcecode!
TypeScript offers two unique kinds of ‘for’ loops that are suitable for different situations:
- for…of loop
- for…in loop
What is for…of Loop?
You can use the “for…of” loop to go through items like arrays or lists. It’s easier than using the old ‘for’ loop.
Syntax:
for (var item of array) {
// use item here
}
Example:
let Grades = [85, 87, 90, 95, 99];
for (var result of Grades) { ✅
console.log(result);
}
Output:
85
87
90
95
99
Apart from that, we can use the ‘for…of’ loop to retrieve a character from the string value
Here’s an illustration:
let SampleString = "ITSOURCECODE";
for (var result of SampleString) { ✅
console.log(result);
}
Output:
"I"
"T"
"S"
"O"
"U"
"R"
"C"
"E"
"C"
"O"
"D"
"E"
What is for…in Loop?
Another version of the ‘for’ loop is ‘for…in’. It can work with an array, list, or tuple.
The ‘for…in’ loop goes through a list or collection and gives back an index during each cycle.
Syntax:
for (var val in list) {
//statements
}
Example:
let subjects = ['Programming', 'Web Development', 'Software Development', 'Operating Systems.', 'Data Structures.', 'Networking & Communication']
for(var i in subjects) ✅
{
console.log(i, subjects[i]);
}
Output:
0 Programming
1 Web Development
2 Software Development
3 Operating Systems.
4 Data Structures.
5 Networking & Communication
Remember:
You can also use ‘let’ instead of ‘var’. However, keep in mind that var and let have different scoping rules.
var is function-scoped, which means it’s available within the function it’s declared in.
let is block-scoped, which means it’s only available within the block it’s declared in.
In simple understanding, the key difference is that a variable declared with ‘let’ won’t be reachable outside the ‘for…in‘ loop.
Conclusion
It’s a wrap, we’re done studying the for loop in Typescript, which has a control structure that lets you repeat a block of code a certain number of times.
We’ve also discussed the two unique kinds of ‘for’ loops: for…of loop and for…in loop that you can also use instead of for loop.
Moreover, the ‘for’ loop is a tool that programmers use a lot. It lets you run a piece of code many times, based on a condition you set.
I hope that this tutorial has helped you gain a clear understanding of TypeScript for loop and how to use for loop in your code.
If you have any questions or inquiries, please don’t hesitate to leave a comment below.