The “typeerror: ‘str’ object cannot be interpreted as an integer” error message is a common error message that you might encounter while doing your program in the Python programming language.
If you’re having trouble fixing this error, don’t worry, because we understand you; that’s why we’re here. Do you want to know why? well…
In this article, we are going to explore how to troubleshoot this “typeerror: str object cannot be interpreted as an integer” error message.
What is Python data types?
Before we jump into why the “str object cannot be interpreted as an integer” error, it is also important to have a basic understanding of Python data types. If you don’t know, Python has several built-in data types, such as:
- Integers
- Floats
- Strings
- and lists
Each data type has specific properties and behaviors that affect how they are used in Python scripts.
For instance, an integer is a whole number that is either positive, negative, or zero. On the other hand, a string is a sequence of characters enclosed in quotes, like “Hi, welcome to Itsourcecode.”
In Python, variables are assigned a data type based on the value assigned to them.
What is “typeerror: ‘str’ object cannot be interpreted as an integer” error message?
This “typeerror ‘str’ object cannot be interpreted as an integer” error message occurs when you are trying to perform mathematical operations on a string variable.
In Python, you cannot perform mathematical operations on strings, mainly because they are non-numeric data types.
So that is why, when you attempt to use a string in mathematical calculations, it will raise a “typeerror str object cannot be interpreted as an integer” error because it is unable to interpret the string as a number.
Furthermore, this error message indicates that you need to convert the string to a numeric data type before using it in mathematical operations.
What are the causes of “typeerror: str object cannot be interpreted as an integer” error?
There are various causes of “typeerror ‘str’ object cannot be interpreted as an integer in Python,” but we just get the most common one:
- The primary cause of this error in Python is attempting to perform mathematical operations on a string variable or on an empty string.
- Using an incorrect data type in function arguments.
- Passing the wrong data type to a function.
How to fix the “typeerror: ‘str’ object cannot be interpreted as an integer” error message?
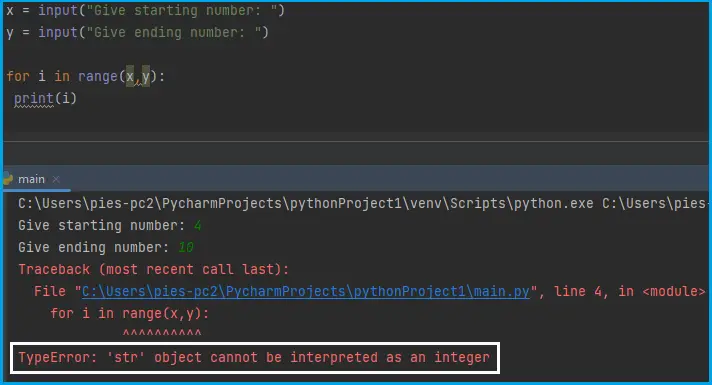
To resolve the “typeerror: str object cannot be interpreted as an integer” error message in Python, you need to ensure that you are performing mathematical operations on variables of the correct data type.
Here are some ways to fix the error:
1. Convert the string to an integer
You can use the ‘int‘ function to convert a string to an integer. If you have a variable “num” that contains a string value, you can convert it to an integer using the “int” function.
x = int(input("Give starting number: "))
y = int(input("Give ending number: "))
for i in range(x, y):
print(i)
Output:
Give starting number: 5
Give ending number: 10
5
6
7
8
9
Another example:
num = "5"
sum= int(num) + 5
print(sum)
Output:
10
2. Verify data type of variables
It is important to check the data type of variables before performing the operations. It ensures that you are not going to perform mathematical operations on string variables.
You can check the data type of a variable using the ‘type’ function as follows:
num = "5"
if type(num) == str:
print("num is a string")
else:
print("num is not a string")
In this example, the ‘if’ statement checks if the variable ‘num‘ is a string, and if it is, it prints a message indicating that it’s a string.
Output:
num is a string
3. Using string formatting to convert variables to strings
The “string” formatting is used to convert the integer variable “num_int” to a string value that can be included in the string.
# Initialize an integer variable
num_int = 5
# Convert the integer to a string using string formatting
string = "The number is {}.".format(num_int)
# Print the string
print(string)
Output:
The number is 5.
Conclusion
By executing the different solutions that this article has given, you can easily fix the “typeerror: ‘str’ object cannot be interpreted as an integer” error message in Python.
We are hoping that this article provides you with sufficient solutions; if yes, we would love to hear some thoughts from you.
You could also check out other “typeerror” articles that may help you in the future if you encounter them.
- Typeerror: minicssextractplugin is not a constructor
- Typeerror: ‘required’ is an invalid argument for positionals
- typeerror: object nonetype can’t be use in ‘await’ expression
Thank you very much for reading to the end of this article. Just in case you have more questions or inquiries, feel free to comment, and you can also visit our official website for additional information.