Are you struggling right now to fix this “typeerror a bytes like object is required not str” error message in Python?
In this article, we’ll delve into how to troubleshoot this “typeerror a bytes-like object is required not ‘str'” error message that you encounter.
This is because we understand that it might be hard for you.
If you don’t know what this error means or why it occurs in your code, that needs to be resolved.
So, what we did was compile all the important details that will help you fix this “python typeerror a bytes like object is required not str” error.
What is a bytes-like object in Python?
A bytes-like object in Python is a sequence of bytes that can be used in place of a bytes object.
This includes objects like bytearray, memoryview, and io.BytesIO.
What is “typeerror a bytes like object is required not str”?
The “typeerror a bytes-like object is required not ‘str'” is an error message in Python.
That occurs when you are trying to pass a string to a function or method that expects a bytes-like object.
Such as:
→ bytearray
→ memoryview
→ certain types of buffers
This error message indicates that the function or method that you are using requires a bytes object or a bytearray object instead of a string.
Furthermore, this error can happen when you are working with binary files or network sockets.
For example:
message = "Itsourcecode!"
message_bytes = message.encode('utf-8')
file = open("file.txt", "wb")
file.write(message)
file.close()
As a result, it will throw an error message.
TypeError: a bytes-like object is required, not 'str'
To fix this error, you may need to convert your string to a bytes-like object using the appropriate method or function, such as bytes() or encode().
To resolve this error we just the replace “file.write(message)” with “file.write(message_bytes)” as you can see below:
message = "Itsourcecode!"
message_bytes = message.encode('utf-8')
file = open("file.txt", "wb")
file.write(message_bytes)
file.close()
What are the root causes of the “typeError a bytes-like object is required, not str” error?
The following are the common root causes of this “Python a bytes like object is required not str” error:
✔ Encoding and decoding errors
This error can occur when you are trying to encode or decode data using the wrong method or encoding type.
✔ Working with binary data
This error can also happen when you try to work with binary data, such as files or network sockets, and you pass a string instead of a bytes-like object.
✔ Mismatched data types
Also include if you are passing a string to a function that expects a different data type, such as an integer or a float.
How to fix the “typeerror a bytes like object is required not str”
After you understand this error thoroughly, let’s jump into the solutions.
Here are the different solutions you may use to fix the “typeerror a bytes-like object is required not ‘str'” error:
1. Use encode method appropriately
Use the encode() method to convert the string to bytes.
mystring = "Itsourcecode!"
mybytes = mystring.encode()
print(mybytes)
Output:
b'Itsourcecode!'
2. Use bytes() function
Use the bytes() function to convert the string to bytes.
mystring = "Itsourcecode!"
mybytes = bytes(mystring, "utf-8")
print(mybytes)
Output:
b'Itsourcecode!'
3. If you have a file you are trying to read or write, open it in binary mode (“rb” for reading and “wb” for writing)
Example code for reading:
with open("file.txt", "rb") as f:
contents = f.read()
decoded_contents = contents.decode()
print(decoded_contents)
The example code opens a file named “file.txt” in binary mode for reading and assigns it to the variable f.
Output:
Welcome to Itsourcecode!
Here’s the complete code and the result:
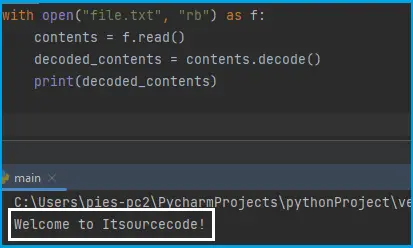
Here’s the file.txt and the result:
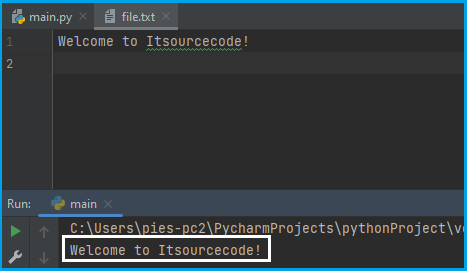
Example code for writing:
message = "Itsourcecode!"
message_bytes = message.encode('utf-8')
file = open("file.txt", "wb")
file.write(message_bytes)
file.close()
Output:
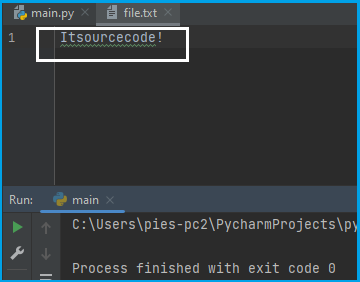
4. Converting a string to bytes using the encode() method when trying to concatenate bytes-like objects
If you are trying to concatenate bytes-like objects, make sure that all of them are bytes-like objects. You can convert a string to bytes using the encode() method:
my_bytes = b"It, "
my_string = "sourcecode!"
my_bytes += my_string.encode()
print(my_bytes)
Output:
b'It, sourcecode!'
Note: In general, the solution to this “bytes-like object is required, not ‘str'” error is to make sure that you are working with the correct data type.
Conclusion
By executing the different solutions that this article has already given, you can easily fix the “TypeError: a bytes-like object is required, not ‘str'” error message while working with Python.
We are hoping that this article provides you with sufficient solutions.
You could also check out other “typeerror” articles that may help you in the future if you encounter them.
- Typeerror unsupported operand type s for dict and dict
- Typeerror: this.clienginector is not a constructor
- Typeerror byte indices must be integers or slices not str
Thank you very much for reading to the end of this article.