In this article, we will discuss PHP trait and how this trait
can be helpful to your programming career, we will also provide a detailed explanation as well as sample programs to easily understand this topic.
In PHP, trait is one way that can enable developers to (reuse
) methods of an independent classes which exist in the different inheritance hierarchies.
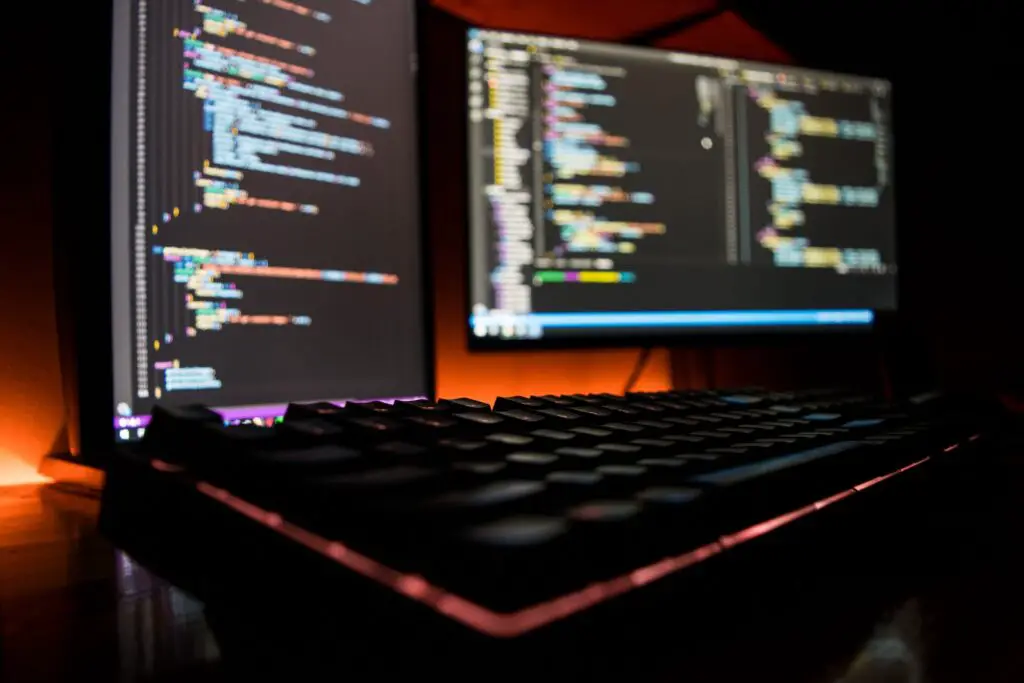
What is a PHP trait?
The traits in PHP are a process for a code to reuse in a single inheritance language. This trait
was intended for reducing some limitations of one inheritance which can be enabled a developer to reuse the sets of methods in a free way into several single classes which stored in different class hierarchies.
In addition, a trait is very similar to a class
, yet it was only intended to a group of functionality in a consistent and fine-grained way. Which is not possible to initialize a trait
on their own. It was an addition to traditional inheritance and also enables the horizontal composition of the behavior.
The trait keyword is used to declare traits in PHP.
Syntax:
<?php
trait TraitName {
// some code...
}
?>
The use keyword is used to trait a class.
Syntax:
<?php
class MyClass {
use TraitName;
}
?>
For example:
<?php
trait message1 {
public function msg1() {
echo "methods freely in several independent classes! ";
}
}
class Welcome {
use message1;
}
$obj = new Welcome();
$obj->msg1();
?>
Output:
methods freely in several independent classes
Multiple traits in PHP
The multiple traits can be easily inserted into a class by simply listing them as a good practice in the use statement, which is separated by commas.
For example:
<?php
trait Hello {
public function sayHello() {
echo 'Glenn Magada Azuelo';
}
}
trait World {
public function sayWorld() {
echo 'and';
}
}
class MyHelloWorld {
use Hello, World;
public function sayExclamationMark() {
echo 'Angel Jude Suarez';
}
}
$o = new MyHelloWorld();
$o->sayHello();
$o->sayWorld();
$o->sayExclamationMark();
?>
Output:
Glenn Magada Azuelo and Angel Jude Suarez
Traits Composed from Traits in PHP
Just other classes they can also make use of traits
and other traits
. By simply using one or more traits in the trait definition, which can be collected entirely or partially of the members which are defined in those other traits.
For example:
<?php
trait Hello {
public function sayHello() {
echo 'I am Glenn Magada Azuelo';
}
}
trait World {
public function sayWorld() {
echo ' A Writer And Computer Programmer';
}
}
trait HelloWorld {
use Hello, World;
}
class MyHelloWorld {
use HelloWorld;
}
$o = new MyHelloWorld();
$o->sayHello();
$o->sayWorld();
?>
Output:
I am Glenn Magada Azuelo A Writer And Computer Programmer
Abstract Trait Members in PHP
The abstract class trait members are used to support all the abstract methods in order to impose all the imposed requirements upon the base class, protected, and private methods are exhibiting.
For example:
<?php
trait Hello {
public function sayHelloWorld() {
echo 'Hello'.$this->getWorld();
}
abstract public function getWorld();
}
class MyHelloWorld {
private $world;
use Hello;
public function getWorld() {
return $this->world;
}
public function setWorld($val) {
$this->world = $val;
}
}
?>
Output:
Program did not output anything!
Static traits in PHP
These traits can be defined as a static variable
, static properties, and static methods.
Example program for static variable:
<?php
trait Counter {
public function inc() {
static $num = 0;
$num = $num + 1;
echo "$num\n";
}
}
class num1 {
use Counter;
}
class num2 {
use Counter;
}
$o = new num1(); $o->inc(); // echo 1
$p = new num2(); $p->inc(); // echo 1
?>
Output:
1
1
Example program for static properties:
<?php
trait StaticExample {
public static $static = 'Glenn Magada Azuelo';
}
class Example {
use StaticExample;
}
echo Example::$static;
?>
Output:
Glenn Magada Azuelo
Example program for static methods:
<?php
trait StaticExample {
public static function doSomething() {
return 'Doing something';
}
}
class Example {
use StaticExample;
}
Example::doSomething();
?>
Is PHP trait good?
The traits are totally good in terms of the interfaces which has been offered as substitute. In addition, interfaces are not intended for the use in this way. But rather act as a contracts which have been forced to any classes that can be implemented to deliver its entire functionality.
When did PHP introduce traits?
The traits are introduced in PHP 5.4 version
which the main purpose is to overcome the problem of a single inheritance.
When should I use traits?
- If you want to (reuse) some methods and properties to your codes between multiple classes with the use of trait, this is the best way for extending a class. In this case, the trait is the better option because it does not become a part of the hierarchy type.
- The trait can save a lot of time in some manual (copy and paste-ing) by offering the compile-time (copy and paste-ing) instead.
Can PHP traits have constructors?
In PHP, traits can have a constructor but must be declared as public (an error will occur if it is private or protected).
In addition, you must be familiar enough when using constructors in a trait. There are some instances which may lead to an unintended collision in the composing classes and code duplication.
Can I create object of trait in PHP?
The traits can be an object which can be created because this is very similar to a class. Yet it was only for grouping methods in a consistent and fine-grained way.
The PHP doesn’t allow to create an instance of the trait such as an instance of a class and also there is no concept of a trait in an instance.
Related Articles
- PHP Unlink Function With Example Program
- PHP Coding Standards with Best Example
- PHP Class Variable (Declaration With Examples)
- Const PHP Class (With Example Programs)
- PHP Variable Types (Understanding Variable Types with Examples)
Summary
This article discusses trait and also tackles multiple traits, traits composed from traits, abstract trait members, static traits, when did PHP introduce traits, traits on constructors, and create object on traits.
I hope this lesson has helped you learn a lot. Check out my previous and latest articles for more life-changing tutorials that could help you a lot.