Learn and understand what is event loop in JavaScript and how the event loop manages asynchronous events.
Allowing it to handle multiple tasks at once. This mechanism works on a single thread, using a queue to process tasks in a non-blocking way.
Explore illustrations to see how callbacks are executed and understand how the event loop ensures efficient and responsive web applications.
Improve your JavaScript skills with this straightforward guide to the event loop.
What is event loop in JavaScript?
The event loop is a programming construct that waits for and dispatches events or messages in a program.
It works by making a request to some internal or external “event provider” (that generally blocks the request until an event has arrived), then calls the relevant event handler (“dispatches the event”).
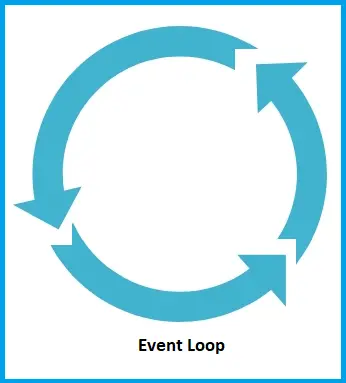
The event loop is a mechanism in JavaScript that manages the execution of code, especially asynchronous operations, on a single thread.
Here’s the illustration of single thread and multi thread:
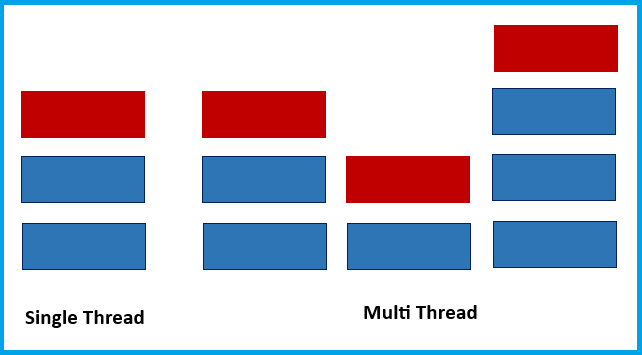
It uses data structures like the call stack, the event queue, and the Web API to process events and tasks, and to move them between different environments.
The event loop gives the illusion of multi-threading and non-blocking behavior, but it can also cause delays or blocking if the tasks are too long or complex.
The event loop can be used with or without libraries like Node.js, React, or Angular.
In simple terms, the event loop is responsible for executing code in a non-blocking manner. When a script runs, it is added to the call stack.
If the script contains asynchronous operations such as setTimeout or fetch, these operations are sent to the Web API to be processed.
Here’s the illustration of the functioning of the event loop well:
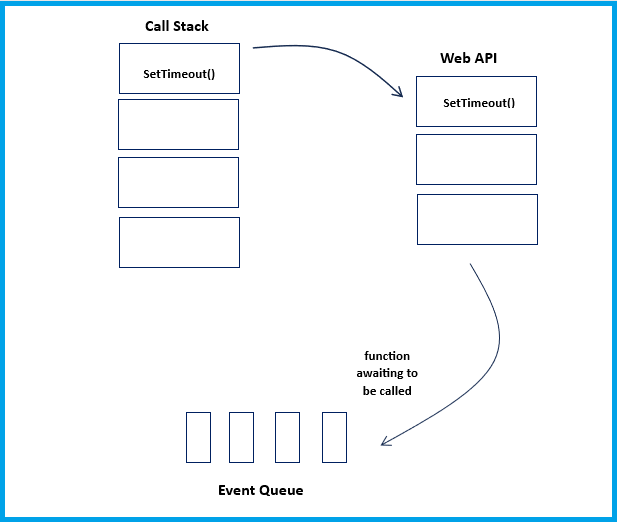
Once the operation is complete, a callback function is added to the event queue. The event loop checks if the call stack is empty.
If it is, it takes the first callback from the event queue and adds it to the call stack to be executed.
Here’s the illustration:
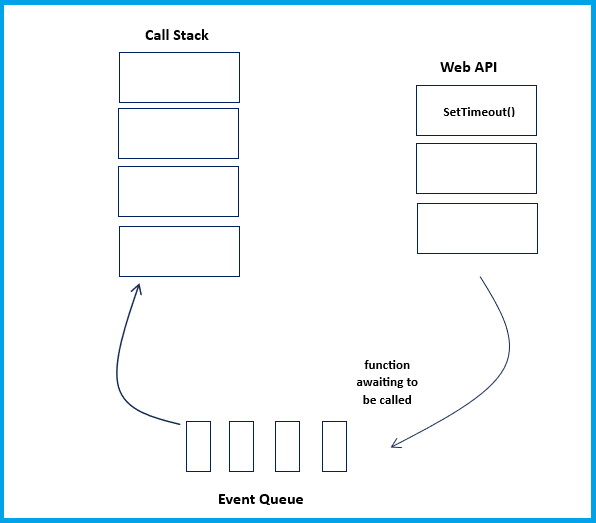
This process continues until all callbacks have been executed and the call stack is empty.
Here’s the illustration when the callbacks has been executed:
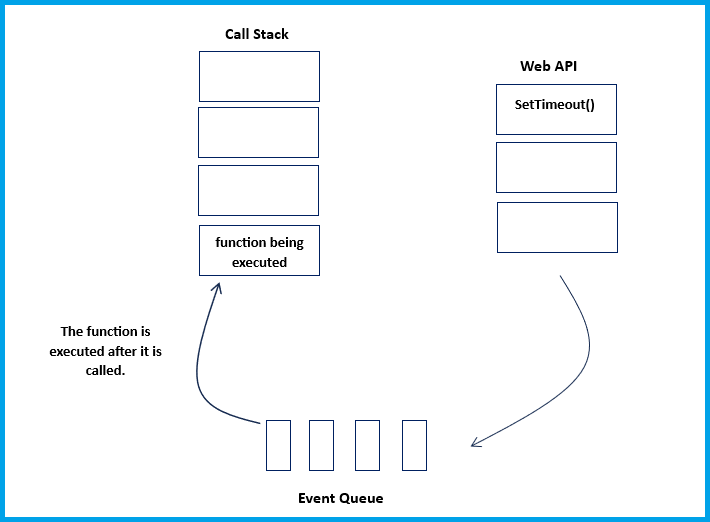
The event loop is important because it takes the first event from the Event Queue and puts it on the stack (which is like a to-do list). In this case, the event is a callback function.
Then, the function is executed, and it may also call other functions inside it.
The event loop is an essential part of how JavaScript works and allows for efficient and responsive web applications.
How event loop works in JavaScript?
As what we mentioned earlier, the event loop is a mechanism that allows JavaScript to handle asynchronous code and perform multiple tasks concurrently, even though it has a single-threaded model.
This means that JavaScript can only do one thing at a time, but the event loop allows it to handle multiple tasks by processing messages in a queue.
Here’s the illustration of event loop in JavaScript.
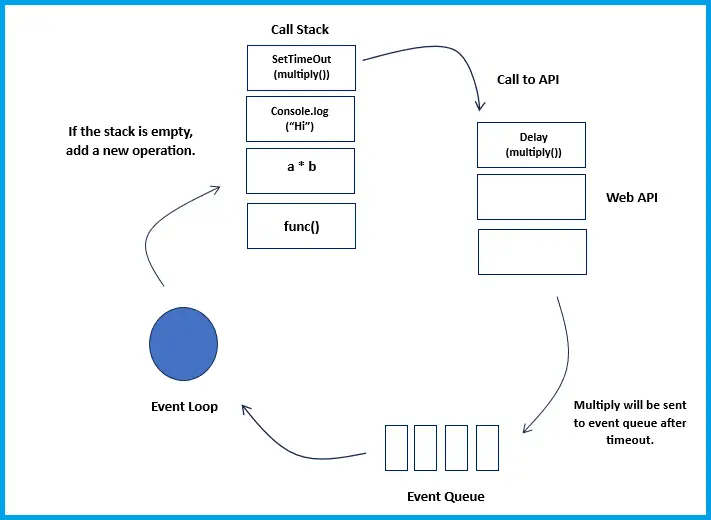
Each message in the queue has an associated function that gets called to handle the message. The event loop processes messages one at a time, starting with the oldest message in the queue.
When the function stack becomes empty, the event loop pulls the next message out of the queue and places it onto the function execution stack.
This gives the illusion of being multithreaded even though JavaScript is single-threaded.
The event loop is an important concept to understand when working with asynchronous code in JavaScript because it allows for non-blocking behavior and efficient handling of multiple tasks.
How the event loop works with setTimeout()?
Here’s an example that demonstrates how the event loop works with setTimeout():
console.log('Start');
setTimeout(function() {
console.log('Timeout');
}, 0);
console.log('End');
In this example, we have three console.log() statements.
The first one logs ‘Start’ to the console, the second one is inside a setTimeout() function and logs ‘Timeout’ to the console after 0 milliseconds, and the third one logs ‘End’ to the console.
When you run this code, you might expect the output to be:
Start
Timeout
End
However, the actual output is:
Start
End
Timeout
This is because the setTimeout() function does not run immediately after its timer expires.
Instead, it adds a message to the queue, which will be processed by the event loop when the function stack becomes empty.
In this case, the function stack is not empty until after the third console.log() statement has been executed, so the message added by setTimeout() is not processed until after ‘End’ has been logged to the console.
This example demonstrates how the event loop works with asynchronous code in JavaScript. Even though the timer for setTimeout() has expired, its callback function does not execute immediately.
Instead, it waits for its turn in the queue and is processed by the event loop when the function stack becomes empty.
Conclusion
In conclusion, this article discusses the event loop in JavaScript, which is a crucial mechanism that allows the language to handle asynchronous code and perform multiple tasks concurrently, even though JavaScript has a single-threaded model.
It utilizes a queue to manage messages, and when the function stack becomes empty, the event loop processes messages one at a time, giving the illusion of being multithreaded.
Understanding the event loop is essential for the efficient handling of asynchronous operations and for creating responsive web applications.
We are hoping that this article provides you with enough information that help you understand what is event loop in JavaScript.
You can also check out the following article:
Thank you for reading itsourcecoders 😊.