In JavaScript, you have the choice to declare variables using the keywords var, let, and const. However, what is the difference between JavaScript Let vs Var vs Const? That is accurately what I will discuss in this tutorial.
If you are new to using JavaScript, there are a few keywords you might encounter:
- Var and let can create a variables that you can change the another value
- The Const can make a constant variables that you can’t change the other value
- Developers should not use var anymore. They should use let or const instead.
- If you cannot change the value of a variable, it is better to use const“.
The first two things are probably easy to understand. But why we shouldn’t use “var“, or when to use “let” instead of “const“?
What is the difference JavaScript var vs let vs const?
To understand the differences in JavaScript Let vs Var vs Const, will consider three elements:
- Scope of variables
- Redeclaration and reassignment
- Hoisting
How to Use var to Declare Variables in JavaScript?
A scope of variables can be declared a var
Variables declared with “var” its whether global or local. Global variables are declared outside functions, while local variables are declared inside functions.
Now, let’s look at some examples, starting with global scope:
var num = 9
function print() {
var squareRoot = num * num
console.log(squareRoot)
}
console.log(num)
print()
The num variable is accessible everywhere because it is declared outside functions and has a global scope.
You can use it for both inside and outside functions.
Here’s an example code for local scope:
function print() {
var num = 20
var squareRoot = num * num
console.log(squareRoot)
}
print()
console.log(num )
In this example code, we created the number variable inside the print function, so it can only be used within that function.
It means that the variable cannot be accessed outside the function where it was created.
If you attempt to access the variable outside the function, you will get an error saying that the variable is not defined.
How to change and assign values to variables declared with var?
Variables created using the keyword “var” can be declared again and assigned a new value.
Let me explain with some examples.
To create a variable using “var,” follow these steps:
var num = 100
You can use the word “var” to declare a variable called “num” and give it an initial value of 100. If you don’t provide an initial value, the default value will be undefined.
var num
console.log(num)
The var keyword enables for redeclaration.
Here’s an example code:
var num = 100
console.log(num)
var num = 200
console.log(num)
In this example code, we changed the variable called num by using the word “var” and provide it an initial value of 200.
Using the word “var” also enables us to change the value of the variable later on.
In this code, we assigned the value 100 to the variable num by saying “var num = 100“.
We can change its value again anywhere in the code because we used “var” to declare it.
Here’s what I refer to:
var num = 100
console.log(num)
num = 200
console.log(num)
num = 300
console.log(num)
Output:
50
100
200
Here, we are not redeclaring it, instead, we’re giving it a new value. When we first said it with a starting value of 100.
Then we gave it a new value of 200 and later on another new value of 300.
How to hoist variables that are declared with var?
When you use the keyword “var” to declare a variable, it is moved to the starting of its scope (either the whole program or a specific part) before the code is executed.
This means that you can use the variable before you actually write the line where it is declared.
Here’s an example code:
console.log(num)
var num = 100
console.log(num)
The number variable in this code is accessible everywhere. When we use var to declare it, the variable is moved to the top of the code.
It means that we can use the variable before we actually declare it, without any errors.
However, the variable is moved with a default value of undefined. So until we assign an initial value to the variable, it will have the value of undefined.
This is an example of local scope:
function result() {
var squareRoot1 = num * num
console.log(squareRoot1)
var num = 10
var squareRoot2 = num * num
console.log(squareRoot2)
}
result()
Output:
NaN
100
Inside the function, it performs the following steps:
- It declares a variable called “squareRoot1” and assigns the square of another variable “num” to it.
- It prints the value of “squareRoot1” to the console.
- It declares a variable called “num” and assigns it the value 10.
- It declares a variable called “squareRoot2” and assigns the square of “num” to it.
- It prints the value of “squareRoot2” to the console.
How to Declare Variables using let in JavaScript?
Variables created using the “let” keyword can exist within different scopes, such as global, local, or block.
Block scope refers to variables declared within a block of code encapsulated by curly braces in JavaScript.
Example:
{
// an example block
}
You can see blocks in if, loop, switch, and some other statements. Any variables you can declare in these blocks using the word “let” will only be accessed within that block.
You cannot use these variables outside of the block.
Here’s an example that shows global, local, and block scope:
let num = 20
function result() {
let squareRoot = num * num
if (num < 60) {
var largerNumberSample = 50
let anotherLargerNumberSample = 90
console.log(squareRoot)
}
console.log(largerNumberSample)
console.log(anotherLargerNumberSample)
}
result()
Output:
In this example, the code initializes a variable “num” with a value of 20.
It defines a function “result” that calculates the square root of “num” and stores it in “squareRoot“.
Inside the function, an if statement checks if “num” is less than 60.
If true, it declares “largerNumberSample” (value: 50) using “var” and “anotherLargerNumberSample” (value: 90) using “let“.
It outputs “squareRoot“. However, accessing “largerNumberSample” and “anotherLargerNumberSample” outside the if statement will result in an error.
Finally, the code calls the “result” function.
How to redeclare and reassign variables declared with let Function
Similar to “var“, when you declare a variable using “let” you can change its value to something else, but you cannot declare it again.
Let’s look at an example of changing the value of a variable:
let num = 21
console.log(num)
num = 35
console.log(num)
Here, we changed the value from 21 to 35 after first setting it.
But if we attempt to change the value of a variable using ‘let’, it will cause an error.
Example code:
let num = 21
let num = 35
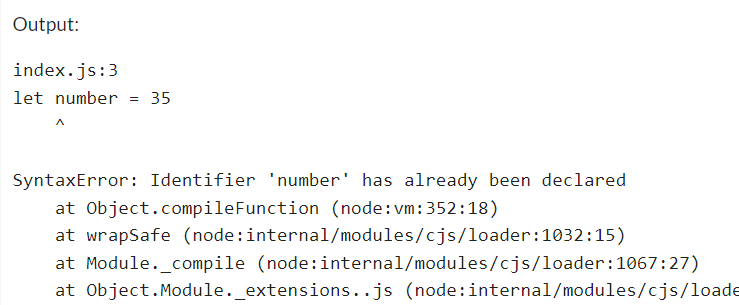
Here, we changed the value to 35 after initially setting it to 21.
However, if we attempt to change the value of a variable using “let”, it will cause an error.
How to Hoist Variables that are Declared using let?
Variables declared with “let” are hoisted without a default value, causing an error when accessed before initialization. Unlike “var”, they are not programmatically set to “undefined”.
Here’s an example code:
console.log(num)
let num = 50
In this example, we have a variable called “num” that is declared globally using the keyword “let“. If we attempt to use this variable before we declare it, we will get an error message that says “Cannot access ‘number’ before initialization“.
How to Declare Variables with const using JavaScript?
Variables declared with const are like let when it comes to scope. These variables can be global, local, or limited to a specific block.
For example:
const num = 10
function result() {
const square = num * num
if (num < 20) {
var largerNumberExample = 30
const anotherLargerNumberExample = 40
console.log(square)
}
console.log(largerNumberExample)
console.log(anotherLargerNumberExample)
}
result()
The code defines a constant variable “num” with a value of 10.
The “result” function calculates the square of “num” and logs it to the console.
Inside the function, there is a conditional statement that declares two variables: “largerNumberExample” using the “var” keyword, and “anotherLargerNumberExample” using the “const” keyword.
The square is logged to the console only if “num” is less than 20.
After the conditional statement, the code attempts to log “largerNumberExample” and “anotherLargerNumberExample” to the console.
Finally, the “result” function is called.
How to redeclare and reassign variables declared with const using JavaScript?
In this case, const is not similar to var and let. Const is used to declare constant variables, which are variables with values that cannot be changed.
These variables cannot be declared again, and they cannot be assigned different values. If you try to do this, it will cause an error.
An example with redeclaration:
const num = 8
const num = 20
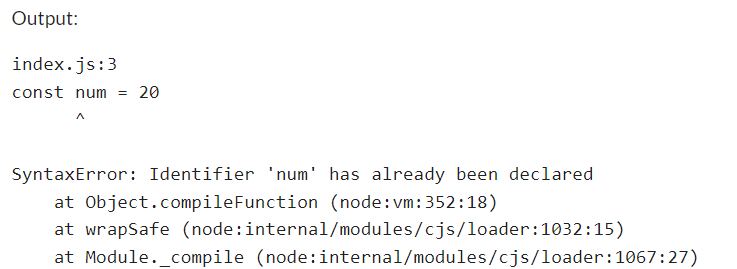
In the example code above, you can see there’s a syntax error in the code. The Identifier has already been declared.
Now, let’s look at an example with reassigning the value:
const number = 2
number = 14
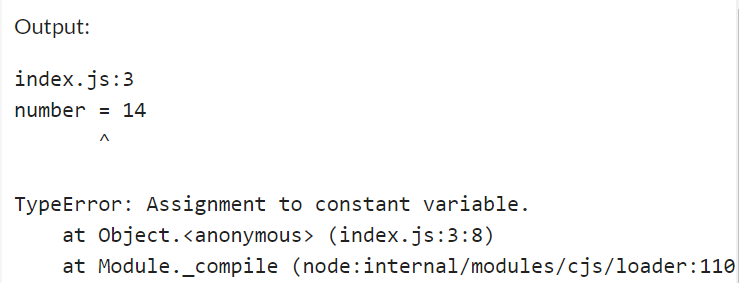
In this example, you can see there is an error where a constant variable is being assigned a value.
How to hoist variables declared with const using JavaScript?
Const variables, like let variables, are moved to the opening of their scope (global, local, or block) when the code is run.
However, const variables are not programmatically assigned a starting value.
On the other hand, var variables are also moved to the starting of their scope and are given a default value of “undefined“.
It means that you can use var variables before they are declared without experiencing any errors.
But if you attempt to access a const variable before it is declared, you will get an error message saying that the variable has not been initialized yet.
For example:
console.log(num)
const num = 100
In this example code, “num” is a variable that can be used anywhere in the program and cannot be changed once it’s set.
If are attempting to use this variable before we declare it, we will get an error message that says we can’t access “num” before it’s defined.
The same error will happen if “num” was a variable that can only be used in a specific part of the program.
Conclusion
In conclusion, it is recommended to use let or const instead of var, depending on whether you need a variable that can be reassigned or a constant variable.
Also, we have discussed the difference between JavaScript Let vs Var vs Const.
Understanding the differences between these keywords is important for writing clean and reliable JavaScript code.
Additional Resources
Here’s an article that can help you learn more about JavaScript: