Are you wondering what are the JavaScript touch events? Confused what events to trigger in particular touch-enabled devices?
In this guide, you will definitely know what are the touch events in Javascript along with their purpose. Expect also example programs that demonstrate the touch events usage.
Let’s get started!
What is a JavaScript touch event?
The JavaScript touch event is a type of event that is triggered on touch-enabled devices such as smartphones or tablets, when the user interacts with the screen.
Additionally, these events allow JavaScript code to respond to various touch-based actions, such as tapping, swiping, or pinching.
Touch events are similar to mouse events in JavaScript but are specifically designed to handle touch interactions.
They provide information about the touch points on the screen, including their coordinates and changes in position over time.
This allows developers to create interactive and responsive web applications that can cater to touch-based inputs.
Kinds of JavaScript Touch Events
Here are some of the kinds of JavaScript touch events which commonly used:
Events | Description |
touchstart | This event is triggered when a finger touches the screen. |
touchmove | This event occurs when a finger moves across the screen. |
touchend | This event is triggered when a finger is lifted off the screen. |
touchcancel | This event is triggered when the touch event is canceled, such as when the touch points move outside the boundaries of the browser window. |
Example Programs of touch events in JavaScript
Here are the following examples which demonstrates the javascript touch events.
Example 1: Detecting a Tap
<!DOCTYPE html>
<html>
<head>
<title>Pinch and Zoom</title>
<style>
#myElement {
width: 200px;
height: 200px;
background-color: blue;
transform-origin: top left;
transition: transform 0.3s;
}
</style>
</head>
<body>
<div id="myElement"></div>
<script>
// Add touch event listeners to an element
var element = document.getElementById('myElement');
element.addEventListener('touchstart', function(event) {
// Prevent default touch behavior
event.preventDefault();
// Store initial finger positions
var touch1 = event.touches[0];
var touch2 = event.touches[1];
var initialDistance = getDistance(touch1, touch2);
// Add touchmove event listener
element.addEventListener('touchmove', handleTouchMove);
});
// Touchmove event handler
function handleTouchMove(event) {
// Prevent default touch behavior
event.preventDefault();
// Calculate current finger positions and distance
var newTouch1 = event.touches[0];
var newTouch2 = event.touches[1];
var currentDistance = getDistance(newTouch1, newTouch2);
// Calculate the scale factor
var scaleFactor = currentDistance / initialDistance;
// Apply scaling to the element
element.style.transform = 'scale(' + scaleFactor + ')';
}
// Add touchend event listener
element.addEventListener('touchend', function(event) {
// Remove touchmove event listener
element.removeEventListener('touchmove', handleTouchMove);
// Reset element scale
element.style.transform = 'scale(1)';
});
// Helper function to calculate distance between two touch points
function getDistance(touch1, touch2) {
var dx = touch1.clientX - touch2.clientX;
var dy = touch1.clientY - touch2.clientY;
return Math.sqrt(dx * dx + dy * dy);
}
</script>
</body>
</html>
Let’s consider an example where we have an HTML document containing various elements. One of these elements is a <div> (division) element, which has been assigned an ID of “myElement“.
We’ve set some styling properties for this particular <div> element, such as a blue background color and a size of 100×100 pixels.
Now, we want to add some interactivity to this <div> element. To achieve this, we attach an event listener specifically for the “touchstart” event.
In simpler terms, we want to detect when the user taps on this <div> element using their touch input.
When a tap occurs on the <div>, the “touchstart” event is triggered. To provide a customized behavior, we prevent the default action that usually takes place when a touch event happens. In this case, we don’t want any standard touch-related actions to occur.
After preventing the default touch behavior, we log a message to the console.
This message serves as a way to indicate that a tap has been detected on the <div> element.
By logging this message, we can track and observe the occurrence of taps on this specific element.
Expected Output: When the user taps on the blue div element, the console will display the message “Tap detected!”.
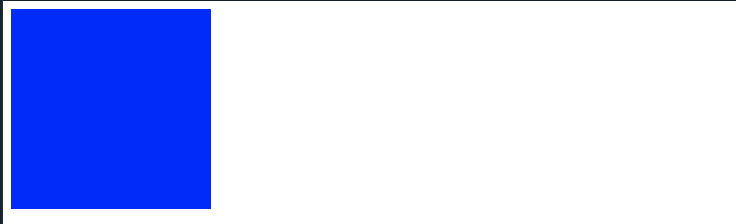
Example 2: Dragging Element
<!DOCTYPE html>
<html>
<head>
<title>Draggable Element</title>
<style>
#myElement {
width: 100px;
height: 100px;
background-color: green;
position: absolute;
}
</style>
</head>
<body>
<div id="myElement"></div>
<script>
// Add touch event listeners to an element
var element = document.getElementById('myElement');
element.addEventListener('touchstart', function(event) {
// Prevent default touch behavior
event.preventDefault();
// Get the initial touch position
var touch = event.touches[0];
var startX = touch.clientX;
var startY = touch.clientY;
// Add touchmove event listener
element.addEventListener('touchmove', handleTouchMove);
});
// Touchmove event handler
function handleTouchMove(event) {
// Prevent default touch behavior
event.preventDefault();
// Calculate the distance moved
var touch = event.touches[0];
var moveX = touch.clientX - startX;
var moveY = touch.clientY - startY;
// Update the element's position
element.style.left = moveX + 'px';
element.style.top = moveY + 'px';
}
// Add touchend event listener
element.addEventListener('touchend', function(event) {
// Remove touchmove event listener
element.removeEventListener('touchmove', handleTouchMove);
});
</script>
</body>
</html>
In this example, we have an HTML document with a draggable <div> element (with ID “myElement“) that has a green background and a size of 100×100 pixels.
We apply CSS styles to position it absolutely. We attach touch event listeners to this element. When the user touches the div (touchstart event), we prevent the default touch behavior and store the initial touch position.
We then add a touchmove event listener that tracks the movement of the touch. Inside the touchmove event handler, we calculate the distance moved by subtracting the current touch position from the initial touch position.
We update the element’s position by setting the left and top CSS properties. When the user lifts their finger off the screen (touchend event), we remove the touchmove event listener.
Expected Output: When the user touches the draggable green div element and moves their finger, the element will follow the finger’s movement on the screen.
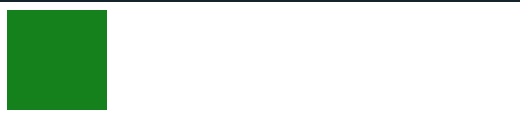
Example 3: Pinching And Zooming
<!DOCTYPE html>
<html>
<head>
<title>Pinch and Zoom</title>
<style>
#myElement {
width: 200px;
height: 200px;
background-color: red;
transform-origin: top left;
transition: transform 0.3s;
}
</style>
</head>
<body>
<div id="myElement"></div>
<script>
// Add touch event listeners to an element
var element = document.getElementById('myElement');
element.addEventListener('touchstart', function(event) {
// Prevent default touch behavior
event.preventDefault();
// Store initial finger positions
var touch1 = event.touches[0];
var touch2 = event.touches[1];
var initialDistance = getDistance(touch1, touch2);
// Add touchmove event listener
element.addEventListener('touchmove', handleTouchMove);
});
// Touchmove event handler
function handleTouchMove(event) {
// Prevent default touch behavior
event.preventDefault();
// Calculate current finger positions and distance
var newTouch1 = event.touches[0];
var newTouch2 = event.touches[1];
var currentDistance = getDistance(newTouch1, newTouch2);
// Calculate the scale factor
var scaleFactor = currentDistance / initialDistance;
// Apply scaling to the element
element.style.transform = 'scale(' + scaleFactor + ')';
}
// Add touchend event listener
element.addEventListener('touchend', function(event) {
// Remove touchmove event listener
element.removeEventListener('touchmove', handleTouchMove);
// Reset element scale
element.style.transform = 'scale(1)';
});
// Helper function to calculate distance between two touch points
function getDistance(touch1, touch2) {
var dx = touch1.clientX - touch2.clientX;
var dy = touch1.clientY - touch2.clientY;
return Math.sqrt(dx * dx + dy * dy);
}
</script>
</body>
</html>
In this example, we have an HTML document with a resizable <div> element (with ID “myElement“) that has a red background and a size of 200×200 pixels. We apply CSS styles to set the transform origin and transition for smooth scaling.
We attach touch event listeners to this element. When the user touches the element with two fingers (touchstart event), we prevent the default touch behavior and store the initial positions of both fingers.
We then add a touchmove event listener that tracks the movement of the fingers.
Inside the touchmove event handler, we calculate the current positions of the fingers and the current distance between them using the helper function getDistance().
Based on the change in distance, we calculate a scale factor to determine the amount of scaling to apply to the element. We update the element’s scale using CSS transformation.
When the user lifts both fingers off the screen (touchend event), we remove the touchmove event listener and reset the element’s scale to 1.
Expected Output: When the user touches the resizable red div element with two fingers and moves them closer (pinch), the element will zoom in. Conversely, when the user moves the fingers apart (zoom out), the element will zoom out smoothly due to the CSS transition effect.
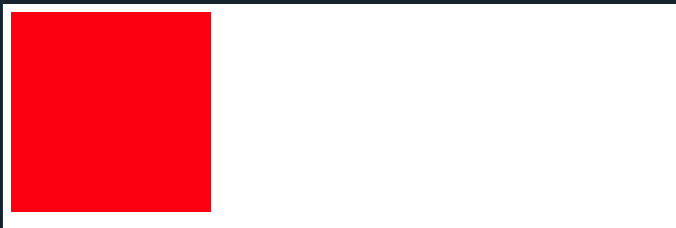
To learn more about JavaScript functions here are other resources you can check out:
- How to Replace div Content JavaScript
- How to get length of dictionary JavaScript | 3 Methods
- Not Equal In JavaScript | Explore How To Use With Examples
Conclusion
In conclusion, Javascript touch events are crucial as essential tools for web developers looking to create touch-eanabled websites with enhanced user interaction.
By harnessing the power of touch gestures, developers can provide users with intuitive and engaging experiences on a variety of devices.
Whether it’s a simple tap, a swipe, or a complex multi-touch interaction, JavaScript touch events enable developers to unlock the full potential of touchscreen devices and deliver seamless web experiences.