In this article, we will explore the JavaScript callstack and how it works to run your code, and why it matters.
Let us discover how it affects your application’s performance and find out the best ways to make the most of it.
So bear with us until the end of this discussion to learn essential data on callstack in JavaScript.
What is the callstack in JavaScript?
The callstack in JavaScript is a mechanism used by the JavaScript interpreter to keep track of its progress in a script that involves multiple function calls.
It helps the interpreter remember which function is currently running and which functions are called from within that function.
Basic principle of callstack
The callstack follows a Last-In-First-Out (LIFO) principle, which means that the most recently called function is the first one to be completed and removed from the stack.
When a script starts executing, the JavaScript engine creates a global execution context and places it on the callstack.
Whenever a function is called, the engine creates a new execution context specific to that function and adds it to the callstack.
If a function calls another function, the engine creates yet another execution context for the called function and adds it to the callstack.
Once a function finishes executing, its execution context is taken off the callstack, and the engine resumes execution from where it left off. The script will stop running when the call stack becomes empty.
Note: Please do not be confused concerning the way we write “callstack” and “call stack.” Don’t worry they’re both the same.
Both “callstack” and “call stack” are acceptable ways to write the term in the context of JavaScript. The term refers to the same concept regardless of whether it is written as one word or two.
What is Push, Pop, and Top?
In stack data structures, there are three fundamental operations: Push, Pop, and Top.
A stack is essentially a collection of elements that adheres to the principle of Last-In-First-Out (LIFO), which means the most recently added element is the first one to be removed.
Push
When we call a function, it gets added to the top of the callstack. It creates a new “stack frame” containing information about the function call, like its arguments and local variables.
Pop
When a function finishes executing, its stack frame is removed from the callstack. This allows the calling function to resume its execution.
Top
The top operation lets us look at the function call at the top of the stack without removing it. We can see the details of the current function call without changing anything in the callstack.
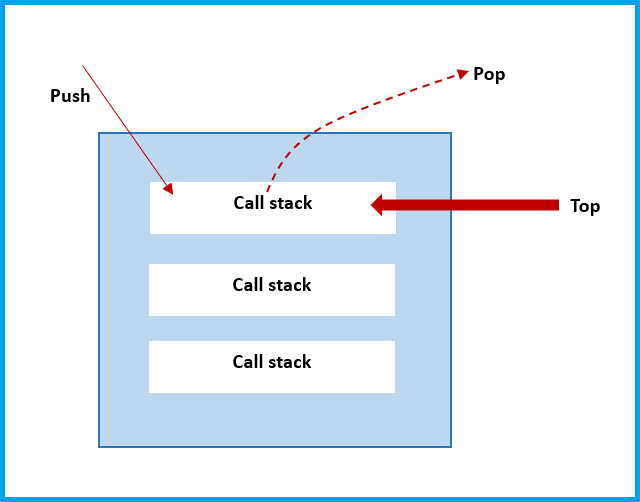
By utilizing these operations, you can add, remove, and examine elements within a stack in a controlled and predictable manner.
These operations help us manage function calls in JavaScript by keeping track of the order in which they are execute
Example of JavaScript callstack
Here’s the example code of call stack in JavaScript:
function multiply(x, y) {
return x * y;
}
function geometricMean(x, y) {
return Math.sqrt(multiply(x, y));
}
let z = geometricMean(100, 20);
console.log(z);
This code does two main things. First, it defines two functions called multiply and geometricMean(). Then, it uses these functions to calculate the geometric mean of two numbers.
The multiply function multiplies two numbers, x and y, together and returns their product.
The geometricMean() function also takes two numbers, x and y, as inputs. It uses the multiply function to find the product of these numbers, and then it takes the square root of the result.
The geometric mean is a special type of average that involves multiplying all the numbers together and then taking the nth root, where n is the total number of numbers.
Since we only have two numbers in this case, the geometric mean is found by taking the square root of their product.
In the code, a variable named z is defined and assigned the value of the geometric mean of 100 and 20. This is done by calling the geometricMean() function with the numbers 100 and 20 as arguments.
Finally, the code uses the console.log() function to display the value of z in the console.
Output:
44.721359549995796
How the JavaScript callstack works?
Here’s the step-by step guide on how the call stack works in JavaScript:
Step 1:
When the code is loaded into memory, the global execution context (for instance global () function) is pushed onto the stack.
After the initial setup is done in the creation phase, the global execution proceeds to the execution phase of its life cycle.
This progression can be observed in the provided image below:
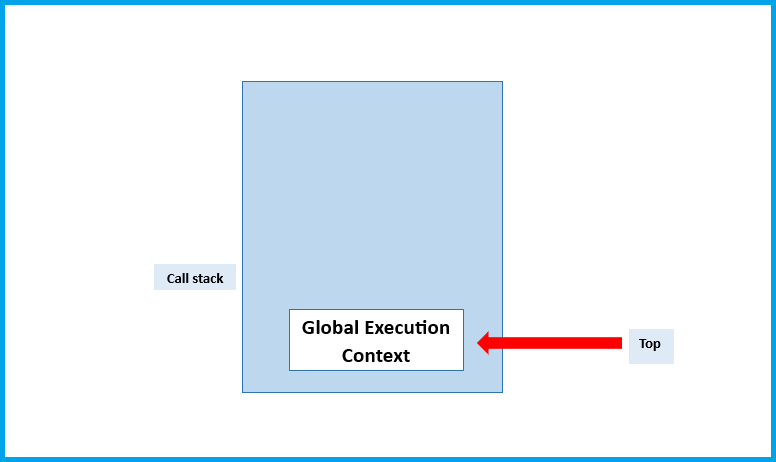
Step 2:
When you call the geometricMean() function, the JavaScript engine creates a special context for this function and puts it at the top of a stack called the “call stack.” This stack keeps track of all the functions being executed.
So, when the geometricMean() function is called, both the global() function and the geometricMean() function are added to this stack.
The geometricMean() function will be at the top of the stack, which means it’s the one currently being executed.
As shown in the following image:
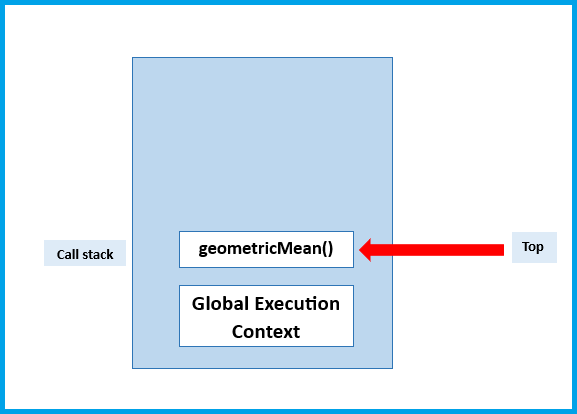
As you can see, the JavaScript engine begins executing the geometricMean() function because it’s the function currently on top of the stack.
Step 3:
In the code, the multiply() function is called within the definition of the geometricMean() function.
This means that the JavaScript engine creates a separate context for the multiply() function and places it at the top of the stack.
Now, the stack contains three functions: global(), geometricMean(), and multiply(), as you will see in the image below.
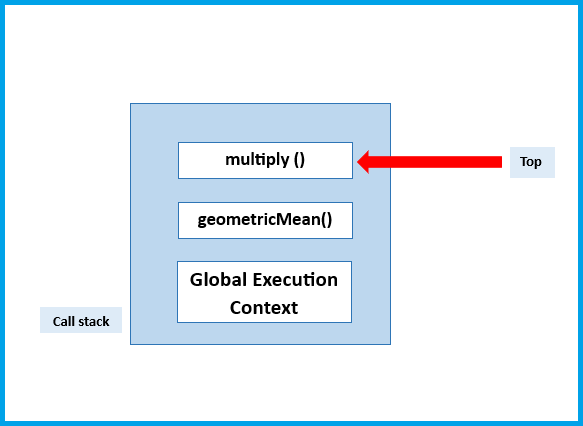
Step 4:
As you can see, there are two separate execution contexts for functions and one global execution context.
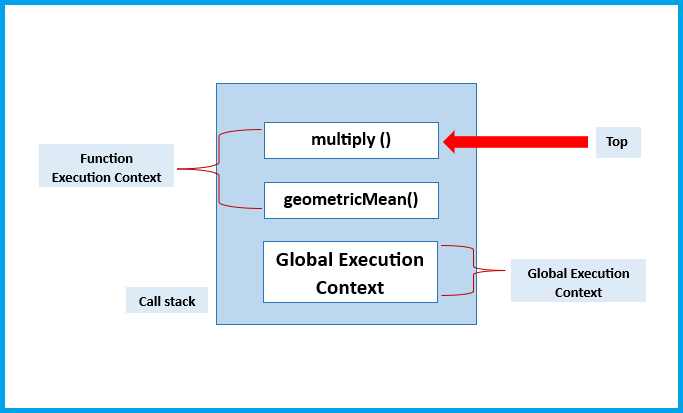
Step 5:
As part of its execution process, the JavaScript engine starts by running the multiply() function first and pops it out.
Kindly refer to the image below, Once the execution of this function is complete, it is taken off the call stack.
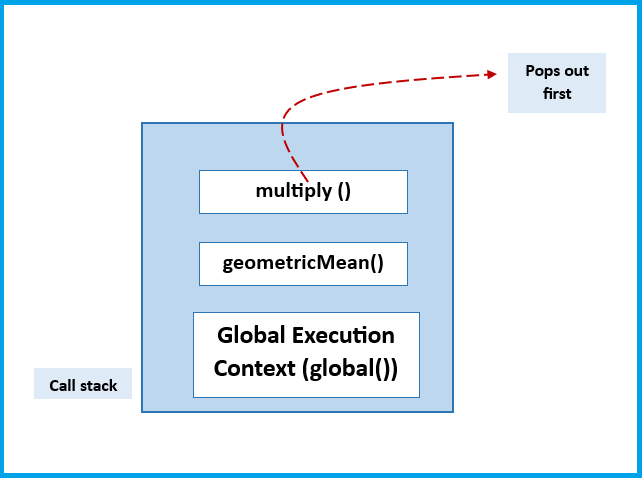
Step 6:
The same things will happen to geometricMean() function will be executed and then pops out from the call stack.
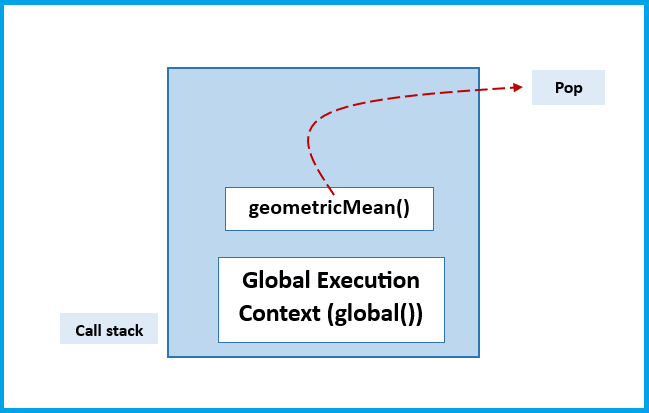
When the execution of both functions completed and there are no more functions left in the call stack to be executed, the JavaScript engine stops executing the call stack and moves on to other tasks.
Then, the call stack becomes empty:
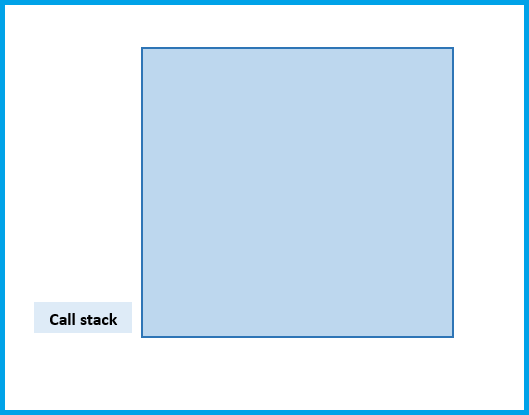
Conclusion
In conclusion, this article gives you a thorough explanation of the callstack in JavaScript and its significance in running code.
This article discusses the basic principles of the callstack, including the Last-In-First-Out (LIFO) principle, and explains the operations of push, pop, and top-in stack data structures.
Also, this article provides a step-by-step process of how the callstack works, from the initialization of the global execution context to the execution and removal of function contexts from the stack.
We are hoping that this article provides you with enough information that help you understand the JavaScript call stack.
You can also check out the following article:
Thank you for reading itsourcecoders 😊.