Learn how to handle the button double-click events in JavaScript using the dblclick event.
This article provides examples and explanations on how to use the addEventListener method to attach an event listener for the dblclick event to an element.
And how to handle the event within the event listener function.
Keep reading because this article equips you with the knowledge and skills to implement this functionality effectively.
What is double click event in JavaScript?
A double click event in JavaScript is an event that is triggered when a user clicks twice quickly on an element on a webpage.
This event can be used to trigger specific actions or behaviors, such as opening a file or zooming in on an image.
If you want something to happen when you double-click on a certain element, you can make it work using the addEventListener() method.
Here’s an example of how you can listen for a double-click event on an element in JavaScript:
element.addEventListener("dblclick", (event) => {
});
As you can see, element is the element that you want to listen for the double click event on.
The addEventListener() method takes two arguments: the first is the type of event to listen for (in this case, “dblclick”), and the second is a callback function that specifies what should happen when the event is triggered.
The callback function receives an event object as its first argument, which contains information about the event that was triggered.
Here’s an example of a complete HTML page that uses the dblclick event to handle double-clicks on an element using JavaScript:
<!DOCTYPE html>
<html>
<head>
<title>Double-click event example</title>
</head>
<body>
<button id="targetElementId">Try to Double-click me!</button>
<script>
var targetElement = document.getElementById("targetElementId");
✅
targetElement.addEventListener('dblclick', function(event) {
alert('You Double-click me! Hi, Welcome to Itsourcecode!');
});
</script>
</body>
</html>
In the above example code, first, we have a button element with the ID “targetElementId.”
After that, the JavaScript code attaches an event listener for the dblclick event to this element in order to detect double clicks on the button.
When the button is double-clicked, the event listener function is called and displays an alert message.
When you open this page in a web browser, you should see a button that says, “You Double-click me! Hi, Welcome to Itsourcecode!”
When you double-click the button, an alert message should be displayed.
Output:
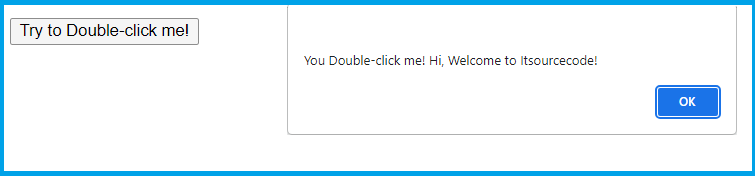
How to double-click on objects using JavaScript?
We can simulate a double-click on an object using JavaScript by dispatching a dblclick event.
Here’s an example of how to do it:
var targetElement = document.getElementById("targetElementId");
var clickEvent = document.createEvent('MouseEvents');
clickEvent.initEvent('dblclick', true, true); ✅
targetElement.dispatchEvent(clickEvent);
Here’s the complete code:
<!DOCTYPE html>
<html>
<head>
<title>Double-click simulation</title>
</head>
<body>
<button id="targetElementId">Try to Double-click me!</button>
<script>
var targetElement = document.getElementById("targetElementId");
var clickEvent = document.createEvent('MouseEvents');
clickEvent.initEvent('dblclick', true, true); ✅
targetElement.dispatchEvent(clickEvent);
targetElement.addEventListener('dblclick', function() {
alert('Double-clicked!');
});
</script>
</body>
</html>
In our example code above, we have a button element with the ID “targetElementId.”
The JavaScript code simulates a double-click on this element by dispatching a dblclick event.
We also add an event listener for the dblclick event on the button, which displays an alert message when the button is double-clicked.
When you open this page in a web browser, you should see a button that says “Try to Double-click me!”
When the page loads, the JavaScript code will automatically simulate a double-click on the button, causing the alert message to be displayed.
Output:
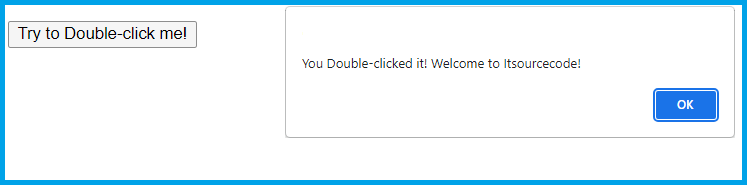
How to differentiate single click and double click in JavaScript?
Differentiating between a single click and a double click in JavaScript can be done in several ways.
An approach involves utilizing the event object’s detail property, which is accessible in modern browsers, including IE-9.
Here’s an example:
element.onclick = event => {
if (event.detail === 1) {
// it was a single click
} else if (event.detail === 2) {
// it was a double click
}
};
In this example, we add an event listener for the click event on an element.
When the element is clicked, the event listener function is called with an event object as its argument.
Afterwards, we can look at the event.detail property’s value to see if it was a single click or a double click.
Another way to differentiate between a single and double click is to use a timer.
Here’s an example:
var clicks = 0;
var timeout;
element.onclick = function(e) {
clicks++;
if (clicks == 1) {
timeout = setTimeout(function() {
console.log('single');
clicks = 0;
}, 250);
} else {
clearTimeout(timeout);
console.log('double');
clicks = 0;
}
};
In this example, we add an event listener for the click event on an element.
When the element is clicked, we increment a clicks counter and start a timer using setTimeout.
If another click occurs before the timer expires, we clear the timer using clearTimeout and consider it a double click.
Contrarily, if the timer ends, we think of it as a single click.
Conclusion
In conclusion, this article has provided a comprehensive guide to handling double-click events in JavaScript using the dblclick event.
You have learned how to attach event listeners to elements using the addEventListener method and how to respond to double-click events within the event listener functions.
This article has also demonstrated techniques to differentiate between single clicks and double clicks using the event. detail property and a timer-based approach.
We are hoping that this article provides you with enough information that helps you understand the double click in JavaScript.
If you want to dive into more JavaScript topics, check out the following articles:
Thank you for reading itsourcecoders 😊.