Find out how to use the JavaScript hasattribute() method to determine if a DOM element has a specific attribute.
This article provides a comprehensive guide that includes clear explanations and code examples so that it is easy for you to use hasattribute in JavaScript.
This tutorial will cover its syntax, parameters, return values, and practical examples to help you understand its usage.
What is hasAttribute() method in JavaScript?
The hasAttribute() is a built-in method in JavaScript that is part of the DOM API. It helps you find out if a specific attribute exists on an HTML element.
This method returns a boolean value when called, true if the attribute exists, and false if it doesn’t.
In a simple understanding, the hasAttribute() method tells you if a specific attribute exists or not. It returns true if the attribute is found, and false if it’s not.
Here’s the syntax for hasAttribute() method:
element.hasAttribute(attributeName);
In this case, the term “element” represents the HTML element you want to check if the attribute exists.
The “attributeName” is a string that specifies the name of the attribute you like to verify.
Note: You can use the setAttribute() method to add a new attribute or change the value of an existing attribute on an element.
Parameters
The hasAttribute() method takes only one parameter:
The attributeName parameter is important and needs to be a string. It should contain the name of the attribute you want to check. Remember, the attribute name is case-sensitive.
Return Value
The hasAttribute() method returns a boolean value:
✅ true
If the attribute you specified exists on the element.
✅ false
If the attribute you specified does not exist on the element.
JavaScript hasAttribute() Example code
Here’s the HTML code:
!DOCTYPE html>
<html>
<body>
<p>Try to click the button to check if it has the "onclick" attribute.</p>
<button id="elem" onclick="change()">Try it</button>
<script>
function change() {
var a = document.getElementById("elem")
var b = a.hasAttribute("onclick");
console.log(b);
}
</script>
</body>
</html>
Here’s the Web View:
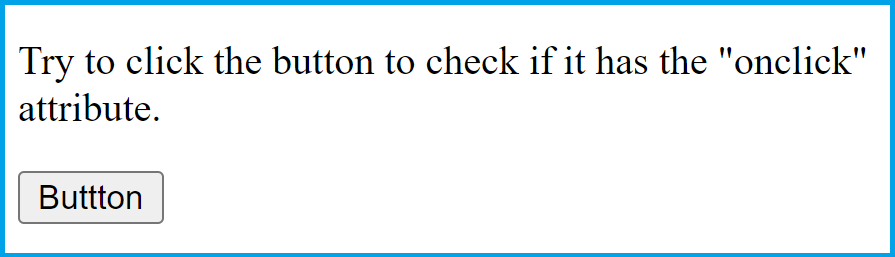
When we click the button, the console shows the result as “true.” This means that the button element with the ID “elem” has the attribute we passed as a parameter.
Here’s the console output:
true
On the other hand, you can also use the following HTML sample code:
<!DOCTYPE html>
<html>
<body>
<p>Try to click the button to check if it has the "onclick" attribute.</p>
<button id="myBtn" onclick="myFunction()">Try it</button>
<p id="demo"></p>
<script>
function myFunction() {
var sample = document.getElementById("myBtn").hasAttribute("onclick");
document.getElementById("demo").innerHTML = sample;
}
</script>
</body>
</html>
Conclusion
In conclusion, this article explains how to use the hasAttribute() method in JavaScript.
This method helps you find out if a specific attribute exists on an HTML element. When you call the hasAttribute() method, it returns true if the attribute is found and false if it’s not.
The article also provides the syntax for using the method, which involves specifying the HTML element you want to check and the name of the attribute you want to verify.
Moreover, article offers a clear and straightforward explanation of how to determine if a DOM element has a specific attribute using JavaScript.
If you’re confused about whether the DOM Element hasAttribute() method and the hasAttribute method in JavaScript are the same, the answer is yes.
The hasAttribute() method is a method of the DOM Element interface in JavaScript.
When people refer to the hasAttribute method in JavaScript, they are usually referring to the hasAttribute() method of the DOM Element interface.
We are hoping that this article provides you with enough information that helps you understand the JavaScript hasattribute() method.
You can also check out the following article:
- How to clamp numbers in JavaScript using math.clamp()?
- Wait until an element exists in JavaScript
- How to get ID from array of objects in JavaScript?
Thank you for reading itsourcecoders 😊.