One of the simple tasks in JavaScript is to print the contents of a nested object. In this article, you will have to learn how to print nested object in JavaScript.
Whether you are a beginner or a professional developer, this article will provide you with the knowledge and proficiency needed to print nested objects effectively.
Method to Print Nested Object in JavaScript
Printing a nested object in JavaScript needs changing through the object’s properties and accessing their values.
Let’s move on into the different methods you can apply to finish this task.
Method 1: Using a Recursive Function
The first method to print a nested object in JavaScript is by using a recursive function.
This method allows you to change through the object’s properties and their nested objects recursively.
Here’s an example code of how you can apply a recursive function to print a nested object:
function printNestedObjectExample(obj, indent = 0) {
for (let value in obj) {
if (typeof obj[value] === 'object') {
console.log(`${' '.repeat(indent)}${value}:`);
printNestedObjectExample(obj[value], indent + 2);
} else {
console.log(`${' '.repeat(indent)}${value}: ${obj[value]}`);
}
}
}
// Usage
const person = {
name: 'Jude',
age: 27,
address: {
street: '15 Suarez St',
city: 'Los Angeles',
country: 'USA'
}
};
printNestedObjectExample(person);
Output:
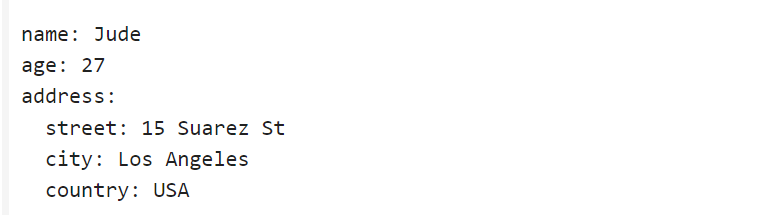
This recursive method enables you to handle objects with inconsistent levels of nesting, making it a functional solution for printing nested objects.
Method 2: Using JSON.stringify
Another method for printing nested objects is by using the JSON.stringify method.
This method changes a JavaScript object to a JSON string illustration.
Here’s an example code that uses the JSON.stringify to print a nested object:
const person = {
name: 'Jude',
age: 27,
address: {
street: '15 Suarez St',
city: 'Los Angeles',
country: 'USA'
}
};
const nestedObjString = JSON.stringify(person, null, 2);
console.log(nestedObjString);
In this example code, the JSON.stringify method provides a suitable method to print nested objects in a structured JSON format.
However, Do not forget that this method will convert the object to a string, so you won’t be able to access individual properties directly.
FAQs
To print a nested object, you can use a recursive function that changes through the object’s properties and handles nested objects.
Yes, there are several libraries available that provide service functions to print nested objects.
One of the popular library is lodash, which offers a .get method to retrieve nested properties and a .forEach method for iterating over objects.
Yes, you can customize the output format when printing nested objects. The recursive function method enables you to format the output as per your requirements.
To print nested objects in a browser console, you can use console.log or console.dir. Both methods accept objects as parameters and display them in a structured format.
Conclusion
In conclusion, printing nested objects in JavaScript can be accomplished using different methods.
Whether you choose a recursive function or the JSON.stringify method, understanding these methods will allow you to work efficiently with nested objects in your JavaScript applications.
By applying the knowledge outlined in this article, you can confidently handle and print nested objects, making your code more readable and maintainable.