When it comes to functioning with arrays in JavaScript, it’s important to understand how to manage the data stored for them adequately and How to Move Item in Array JavaScript.
One of the simple operation is moving items within an array. In this article, you will have to learn the different techniques and methods to finish this task successfully.
Even if you’re a beginner or an professional JavaScript developer, this article will provide you with the knowledge and understanding to move items in an array easily.
Methods to Move Item in Array JavaScript
To start, here are the following methods to move item in Array using JavaScript.
The JavaScript provides several built-in methods that simplify this process.
Method 1: Using the splice() Function
The splice() function enables you to add or remove elements from an array.
To move an item, you need to define the starting index and the number of elements to eliminate, followed by the new item(s) you want to insert.
Here’s an example code that uses the splice function:
const sampleArray = ['orange', 'strawberry', 'mango'];
function printArrayExample(sampleArray) {
console.log('Array contents:', sampleArray.join(', '));
}
// Print array before splice
printArrayExample(sampleArray);
// Perform splice operation
const removedFruits = sampleArray.splice(1, 1);
sampleArray.splice(2, 0, ...removedFruits);
// Print array after splice
printArrayExample(sampleArray);
Output:
In the example above, we first remove the item at index 1 (‘strawberry‘) using splice() function.
Then, we insert the removed item at index 2, completely moving it within the array.
Method 2: Using the slice() Function
Another method is to use the slice() method in combination with other array handling techniques, such as concat() or the spread operator (…).
Let’s see an example code that uses the slice () function:
const person = ['jude', 'glenn', 'caren'];
const personToMove = person[1];
const newArraySample = person.slice(0, 1).concat(person.slice(2));
const finalArray = [newArraySample[0], personToMove, ...newArraySample.slice(1)];
// Print method to display array contents
function printArray(array) {
console.log(array.join(', '));
}
// Call the print method to display finalArray
printArray(finalArray);
In the above example, we extract the person to be moved (‘glenn‘) from the original array using slice() function and store it in a separate variable.
Then, we make a new array by linking the elements before and after the item to be moved.
Finally, we insert the person back into the proper position using the spread operator.
Advanced Techniques for Moving Array Items in JavaScript
Now that we have understand the basics, let’s move on into some advanced techniques to move items within an array using JavaScript.
1. Using the sort() Function
The sort() function is essentially used for sorting arrays. However, with a little ingenuity, we can use it to move items as well.
By specifying a custom sorting function, we can control the order in which elements are arranged.
Let’s see an example code:
const person = ['jude', 'caren', 'glenn'];
person.sort((a, b) => {
if (a === 'caren') return -1;
if (b === 'caren') return 1;
return 0;
});
const printArraySample = (arr) => {
for (let x = 0; x < arr.length; x++) {
console.log(arr[x]);
}
};
printArraySample(person);
In this example, we specify a sorting function that set up the element ‘caren‘. By assigning a higher or lower value to elements based on our proper order, we can completely move the ‘caren‘ element within the array.
2. Using the map() function
The map() function provides a refined solution for moving array items based on precise conditions.
By mapping over the array and reorganizing the elements according to our conduct, we can manage the proper element movement.
Here’s an example code:
const elementArray = ['Caren', 'Jude', 'Glenn'];
const itemToMove = 'Jude';
const printArraySample = (arr) => {
console.log('Array:', arr.join(', '));
};
console.log('Original Array:');
printArraySample(elementArray);
const newExampleArray = elementArray.map((item) => {
if (item === itemToMove) return undefined;
return item;
}).filter((item) => item !== undefined);
newExampleArray.push(itemToMove);
console.log('Modified Array:');
printArraySample(newExampleArray);
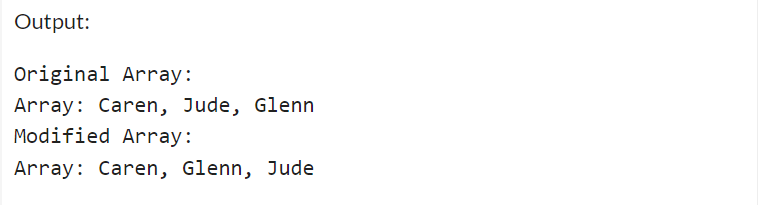
When you run this code, it will first print the original array, and then it will print the modified array after removing the itemToMove and adding it back to the end.
The printArraySample method is specified to display the elements of the array separated by commas.
FAQs
Yes, you can move numerous elements within an array simultaneously. The techniques mentioned earlier can be adapted to handle multiple items.
If you don’t know the index of the item you want to move, you can use methods like indexOf() or findIndex() to locate the item’s index within the array.
Yes, you can move items conditionally by accumulating the if statements or other logical checks into your moving logic.
Yes, you can move items to an exact position within the array. The techniques mentioned earlier enable’s you to control the placement of items by defining the proper index.
Conclusion
In conclusion, you have learned the different techniques for moving items within an array in JavaScript.
We started with the basic methods, such as splice() and slice(). Then, we also discussed the advanced techniques such as using sort() and map() to achieve more specific item movements.
By applying these techniques, you can confidently manage the contents of arrays and create a powerful JavaScript applications.