Are you wondering how to create two-dimensional arrays or 2D arrays in JavaScript? Don’t worry!
We understand you because when working with JavaScript, we’re just knowledgeable about making and using lists called one-dimensional arrays.
But today, we will dive into the world of two-dimensional arrays in JavaScript.
In this article, you will learn how to create, use, and modify 2D arrays in JS with easy-to-follow examples.
What is a 2D array in JavaScript?
A 2D array or also known as two-dimensional array is an array of arrays, where each element of the outer array is itself an array.
It is a handy tool for arranging and working with data in a structured manner.
These are arrays that can hold things like numbers or words, either all the same kind or a mix of different kinds.
This allows us to represent data in a tabular format, with rows and columns.
How to create a 2D (two-dimensional) array in JavaScript?
To create a 2D array in JavaScript, you can follow these steps:
- Declare an empty array to hold the rows of the 2D array.
let sampleArray = [];
- Use a loop to add rows to the array. Each row is an array itself, representing a row of the 2D array.
for (let i = 0; i < 3; i++) {
sampleArray[i] = [];
}
- Use nested loops to add elements to each row of the 2D array.
for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
sampleArray[i][j] = i * 3 + j + 1; ✅
}
}
- After running this code, sampleArray will be a two-dimensional array with three rows and three columns, containing the values 1 through 9.
Here’s the complete code:
let sampleArray = [];
for (let i = 0; i < 3; i++) {
sampleArray[i] = [];
}
for (let i = 0; i < 3; i++) {
for (let j = 0; j < 3; j++) {
sampleArray[i][j] = i * 3 + j + 1; ✅
}
}
console.log(sampleArray);
Output:
[ [ 1, 2, 3 ], [ 4, 5, 6 ], [ 7, 8, 9 ] ]
Another example on creating a two-dimensional array in JavaScript
In JavaScript, you can create a 2D array by initializing an array with other arrays as its elements.
Here’s an example:
let sampleArray = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
console.log(sampleArray[0][1]) ✅
In this example, sampleArray is a 2D array with three rows and three columns.
To access an element in the array, you can use two sets of square brackets. The first set specifies the row index and the second set specifies the column index.
As you can see in the given example above, in order to access the element in the first row and second column, you have to use sampleArray[0][1], which would return the following.
Output:
2
You can also use loops to iterate over the elements in a 2D array.
For example, you can use a nested for loop to print all the elements in the array:
// Create a 2D array with 4 rows and 4 columns
let sampleArray = new Array(4);
for (let i = 0; i < sampleArray.length; i++) {
sampleArray[i] = new Array(4);
}
// Add some values to the array
sampleArray[0][0] = 'A';
sampleArray[0][1] = 'B';
sampleArray[0][2] = 'C';
sampleArray[0][3] = 'D';
sampleArray[1][0] = 'E';
sampleArray[1][1] = 'F';
sampleArray[1][2] = 'G';
sampleArray[1][3] = 'H';
sampleArray[2][0] = 'I';
sampleArray[2][1] = 'J';
sampleArray[2][2] = 'K';
sampleArray[2][3] = 'L';
sampleArray[3][0] = 'M';
sampleArray[3][1] = 'N';
sampleArray[3][2] = 'O';
sampleArray[3][3] = 'P';
// Print the array to the console
for (let i = 0; i < sampleArray.length; i++) {
let row = '';
for (let j = 0; j < sampleArray[i].length; j++) {
row += sampleArray[i][j] + ' '; ✅
}
console.log(row);
}
Output:
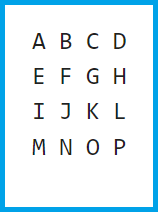
How to access 2D (two-dimensional) array in JavaScript?
You can access the elements of a 2D array in JavaScript using two sets of square brackets.
The first set of brackets specifies the row index, and the second set of brackets specifies the column index.
Here’s an example:
let sampleArray = [
[10, 20],
[30, 40],
[50, 60]
];
console.log(sampleArray[0][0]); ✅
console.log(sampleArray[0][1]); ✅
console.log(sampleArray[1][0]); ✅
console.log(sampleArray[1][1]); ✅
console.log(sampleArray[2][0]); ✅
console.log(sampleArray[2][1]); ✅
Output:
10
20
30
40
50
60
In our example code sampleArray is a 2D array with three rows and two columns.
We can access the elements in the first row using sampleArray[0][0] and sampleArray[0][1], and the rest of elements in the second and third row using two sets of square brackets.
You can also use loops to iterate over the elements in a 2D array.
For example:
let sampleArray = [
[10, 20],
[30, 40],
[50, 60]
];
for (let i = 0; i < sampleArray.length; i++) {
for (let j = 0; j < sampleArray[i].length; j++) {
console.log(sampleArray[i][j]); ✅
}
}
Output:
10
20
30
40
50
60
How to add all elements in a 2D (two-dimensional) array in JavaScript?
You can add all the elements of a 2D array in JavaScript using a nested loop.
Here’s an example:
let sampleArray = [
[10, 20],
[30, 40],
[50, 60]
];
let sum = 0;
for (let i = 0; i < sampleArray.length; i++) {
for (let j = 0; j < sampleArray[i].length; j++) {
sum += sampleArray[i][j]; ✅
}
}
console.log(sum);
As you can see, we have a 2D array sampleArray with three rows and two columns.
We use a nested loop to iterate over the elements in the array. The outer loop iterates over the rows, and the inner loop iterates over the columns.
We also use the += operator to add the value of each element to the sum variable.
After the loops have been completed, the sum variable contains the sum of all the elements in the array.
Output:
210
How to add, remove, and update elements in a 2D array in JavaScript?
You can add, remove, and update elements in a 2D array in JavaScript, using various methods such as push, pop, and splice.
The following are the examples that demonstrate how to perform these operations on a 2D array.
Let’s just say we have a 2D array called data that looks like this:
let data= [
["Anna" , 18],
["Bea" , 19]
];
This array has two rows and two columns. Now, let’s say we want to add a new row to the array. We can do this using the push method:
data.push(["Clare", 20]); ✅
Here’s the complete code:
let data= [
["Anna" , 18],
["Bea" , 19]
];
data.push(["Clare", 20]);✅
console.log(data)
This time, our array which is called data looks likes like the following code:
[ [ 'Anna', 18 ], [ 'Bea', 19 ], [ 'Clare', 20 ] ]
We’ve added a new row to the array! Next, let’s say we want to add a new column to the array.
We can do this by looping over each row and using the push method to add a new element to each row.
for (let i = 0; i < data.length; i++) {
data[i].push("female"); ✅
}
Here’s the complete code:
let data = [
["Anna", 18],
["Bea", 19],
["Claire", 20]
];
for (let i = 0; i < data.length; i++) {
data[i].push("female"); ✅
}
console.log(data);
Now, our array called data looks like the following code:
[
[ 'Anna', 18, 'female' ],
[ 'Bea', 19, 'female' ],
[ 'Claire', 20, 'female' ]
]
We already added a new column to the array!
Now, let’s say we want to remove the first row from the array. We can do this using the splice method, like this:
data.splice(0, 1); ✅
Here’s the complete code:
let data = [
[ 'Anna', 18, 'female' ],
[ 'Bea', 19, 'female' ],
[ 'Claire', 20, 'female' ]
];
data.splice(0, 1); ✅
console.log(data);
We’ve removed the first row from the array! Now, the array looks like this:
[ [ 'Bea', 19, 'female' ], [ 'Claire', 20, 'female' ] ]
This time, let’s say we want to remove the first column from the array.
We can do this by looping over each row and using the splice method to remove the first element from each row.
for (let i = 0; i < data.length; i++) {
data[i].splice(0, 1); ✅
}
Here’s the complete code:
let data = [
[ 'Anna', 18, 'female' ],
[ 'Bea', 19, 'female' ],
[ 'Claire', 20, 'female' ]
];
for (let i = 0; i < data.length; i++) {
data[i].splice(0, 1); ✅
}
console.log(data);
We already have removed the first column from the array! Our array which is called data will like the following code:
[ [ 18, 'female' ], [ 19, 'female' ], [ 20, 'female' ] ]
Now, let’s say we want to update one of the arrays in our example:
let data = [
[ 'Anna', 18, 'female' ],
[ 'Bea', 19, 'female' ],
[ 'Claire', 20, 'female' ]
];
data[0][0] = "updata sample"; ✅
console.log(data)
Here, have a 2D array called data that contains three rows and three columns.
Each row represents a person’s data, with the first element being their name, the second element being their age, and the third element being their gender.
The line data[0][0] = “updata sample”; updates the first element of the first row of the data array.
This changes the name of the first person from “Anna” to “update sample.”
After this update, the data array looks like this:
[
[ 'update sample', 18, 'female' ],
[ 'Bea', 19, 'female' ],
[ 'Claire', 20, 'female' ]
]
Conclusion
In conclusion, this article discusses the 2D (two dimensional) arrays in JavaScript, which are like tables of data made up of rows and columns.
You have learned how to create, access, and change elements in these arrays using practical examples.
With this newfound knowledge, you can now organize and manage data in a structured manner, making your JavaScript coding more powerful and efficient.
We are hoping that this article provides you with enough information that helps you understand the 2d array in JavaScript.
If you want to dive into more JavaScript topics, check out the following articles:
- Length of an array in JavaScript
- .charat method in JavaScript
- JavaScript string repeat documentation
Thank you for reading itsourcecoders 😊.