Today will equip you with the knowledge of how to dynamically add options to a select element in JavaScript.
When it comes to web forms, the <select> element is a fundamental component that allows users to make choices from a dropdown list.
In this article, we will hand you the solutions for adding options to a select element in JavaScript.
Solutions on how to add options to select in JavaScript
You can easily add options to a select dropdown using JavaScript. Just follow these simple steps:
Solution 1: Creating and appending option elements
One way to add options to a select element is to create new option elements and append them to the select element as its child.
Here’s an example that creates options from 1 to 100 in a select with id=”selectElementId”:
const min = 1;
const max = 100;
const select = document.getElementById("selectElementId");
for (let i = min; i <= max; i++) {
const opt = document.createElement("option");
opt.value = i;
opt.innerHTML = i;
select.appendChild(opt);
}
Here’s the complete code:
<!DOCTYPE html>
<html>
<head>
<title>Add Options to Select in JavaScript</title>
<style>
/* Center the content */
body {
text-align: center;
}
</style>
</head>
<body>
<h1>How to Add Options to Select in JavaScript</h1>
<select id="mySelect"></select>
<script>
const min = 1;
const max = 100;
const select = document.getElementById("mySelect");
for (let i = min; i <= max; i++) {
const opt = document.createElement("option");
opt.value = i;
opt.innerHTML = i;
select.appendChild(opt);
}
</script>
</body>
</html>
Output:
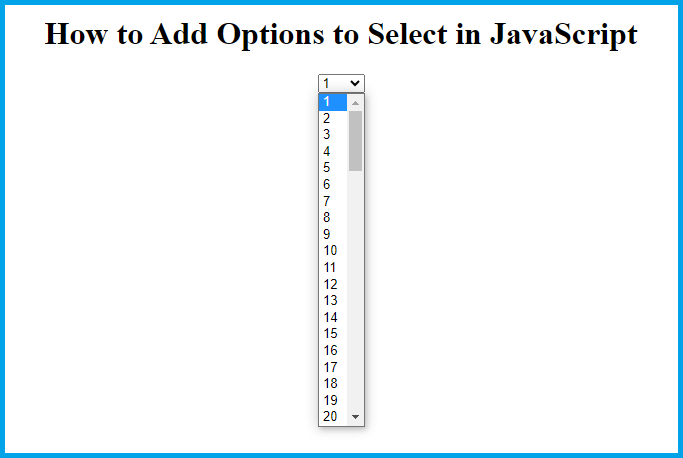
Solution 2: Using the add() method
Another way to add options to a select element is to use the add() method of the select object.
This method takes two arguments: the option element to add, and the index at which to insert the new option.
If no index is specified, the new option will be inserted at the end of the list. Here’s an example:
var x = document.getElementById("mySelect");
var option = document.createElement("option");
option.text = "subjects";
x.add(option);
Here’s the complete code using the add() method:
<!DOCTYPE html>
<html>
<head>
<title>Add Options to Select in JavaScript</title>
<style>
/* Center the content */
body {
text-align: center;
}
</style>
</head>
<body>
<h1>How to Add Options to Select in JavaScript</h1>
<select id="mySelect"></select>
<script>
var x = document.getElementById("mySelect");
var subjects = ["Math", "English", "Science", "History", "Statistics"];
for (var i = 0; i < subjects.length; i++) {
var option = document.createElement("option");
option.text = subjects[i];
x.add(option);
}
</script>
</body>
</html>
Output:
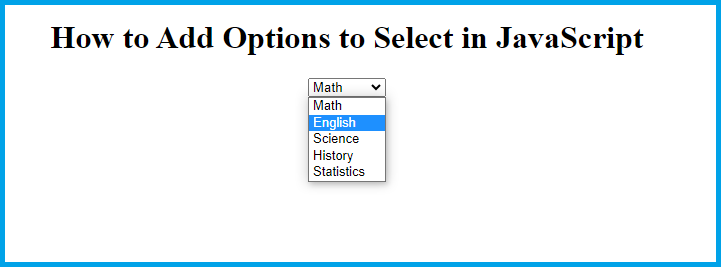
Solution 3: Inserting options at a specific index
You can also use the add() method to insert options at a specific index in the list.
Here’s an example code that adds a “Kiwi” option at index position “2” of a drop-down list:
var x = document.getElementById("mySelect");
var option = document.createElement("option");
option.text = "Kiwi";
x.add(option, x[2]);
Here’s the complete code:
<!DOCTYPE html>
<html>
<head>
<title>Add Options to Select in JavaScript</title>
<style>
body {
text-align: center;
}
</style>
</head>
<body>
<h1>How to Add Option to Select in JavaScript</h1>
<select id="mySelect">
<option>Apple</option>
<option>Banana</option>
<option>Mango</option>
<option>Orange</option>
</select>
<script>
var x = document.getElementById("mySelect");
var option = document.createElement("option");
option.text = "Kiwi";
x.add(option, x[2]);
</script>
</body>
</html>
This code creates a select element with the id “mySelect” and four pre-existing options: “Apple,” “Banana,” “Mango,” and “Orange.”
Output:
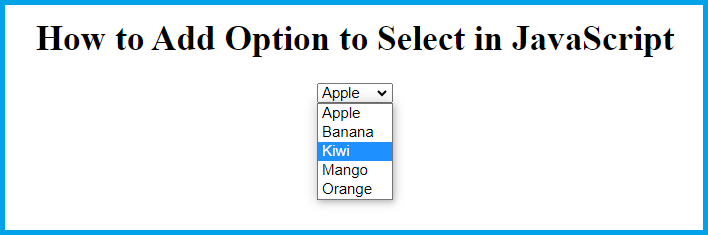
It then adds a “Kiwi” option at index position “2” using the add() method of the select object. The new option is created using the createElement() method, its text property is set to “Kiwi,” and it is inserted at index position “2” using the second argument of the add() method.
Conclusion
In conclusion, this article shows you three easy ways to add options to select in the dropdown list in JavaScript.
You can use a loop to create and add options one by one or use the “add()” method to insert options at the end or a specific position.
These methods help you dynamically populate dropdown lists with options on your website.
We are hoping that this article provides you with enough information that help you understand the JavaScript add option to select.
You can also check out the following article:
Thank you for reading itsourcecoders 😊.