In this article, you will learn about binary in JavaScript and converting decimal numbers and strings to binary in JS.
We will provide detailed explanations and code examples to help you understand the concepts and implement binary conversions in your projects.
You will also learn about the different methods for converting decimal numbers and strings to binary, including using built-in methods and custom functions.
By the end of the article, you’ll have a solid understanding of how to work with binary in JavaScript.
What is binary in JavaScript?
Binary is a way of showing numbers using only two symbols: 0 and 1.
These symbols are called bits, and they can be combined to represent larger numbers.
For example, the number 5 can be represented in binary as 101, which means 12^2 + 02^1 + 1*2^0 = 4 + 0 + 1 = 5.
Binary is used in computers because it’s easy for electronic devices to distinguish between two states, such as on or off. 1 is considered true or “on,” on the other hand 0 is considered false or “off.”
This makes it possible for computers to store and process large amounts of information using binary code.
Binary Numbers in JavaScript
In JavaScript, numbers are stored in a specific format called double-precision 64-bit binary format IEEE 754.
This means that numbers are represented using binary values, with a precision of 53 bits.
JavaScript allows you to use different number systems when writing numeric literals, such as decimal (no prefix), hexadecimal (prefix 0x), and octal (prefix 0).
However, there is no built-in support for binary literals. One way to work with binary numbers in JavaScript is to use the parseInt() method with a radix parameter of 2.
Here’s an example:
const binaryString = "1110";
const number = parseInt(binaryString, 2);
console.log(number);
In our example code, we use the parseInt() method with a radix of 2 to convert the binary string “1110” to its decimal representation, which you’ll see in the output below.
Output:
14
JavaScript binary operators
JavaScript includes binary and unary operators, along with a special ternary operator known as the conditional operator.
Binary operators are operators that require two operands, one before the operator and one after the operator.
Operand1 operator operand2. For example, 5 + 3 or x * y.
Here is a complete list of the different types of binary operators in JavaScript:
Assignment operators:
=, +=, -=, *=, /=, %=, **=, <<=, >>=, >>>=, &=, ^=, |=
Comparison operators:
==, !=, ===, !==, <, >, <=, >=
Arithmetic operators:
+, -, *, /, %, **
Bitwise operators:
&, |, ^, ~, <<, >>, >>>
Logical operators:
&&, ||
String operator:
+
Conditional (ternary) operator:
? :
Comma operator:
,
Unary operators:
+, -, ++, --, !, ~, typeof,delete,void
Relational operators:
in andinstanceof
How to convert string to binary in JavaScript?
You can convert a string to binary in JavaScript by using the charCodeAt() method to get the ASCII code of each character in the string.
And then converting that code to binary using the toString() method with a radix parameter of 2.
Here’s an example function that takes a string as input and returns its binary representation:
function stringToBinary(string) {
let binaryOutput = '';
for (let i = 0; i < string.length; i++) {
binaryOutput += string[i].charCodeAt(0).toString(2) + ' '; ✅
}
return binaryOutput;
}
const sampleString = "Itsourcecode";
const binaryString = stringToBinary(sampleString);
console.log(binaryString);
Output:
1001001 1110100 1110011 1101111 1110101 1110010 1100011 1100101 1100011 1101111 1100100 1100101
Examine the provided chart and attempt to compose a word using UTF-8 binary encoding. Experiment with your own name!
Locate the 8-bit binary representation for each letter in your name, and record it with a slight gap between each group of 8 bits.
As an illustration, if your name begins with the letter “A,” your initial character would be represented as 01000001.
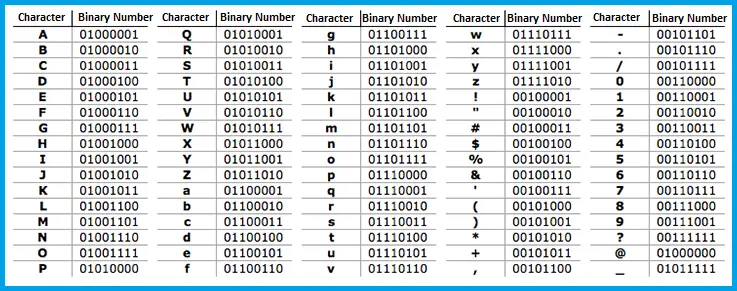
How to convert Decimal number to Binary in JavaScript
There are several ways to convert a decimal number to binary in JavaScript.
Decimal Number | Binary Number |
---|---|
0 | 0000 |
1 | 0001 |
2 | 0010 |
3 | 0111 |
4 | 0100 |
5 | 0101 |
6 | 0110 |
7 | 0111 |
8 | 1000 |
9 | 1001 |
10 | 1010 |
One way is to use the toString() method with a radix parameter of 2, which specifies that the number should be converted to base 2 (binary).
Here’s an example:
const number = 11;
const binary = number.toString(2); ✅
console.log(binary);
Output:
1011
Here’s another example:
const number = 11.5;
const binary = number.toString(2); ✅
console.log(binary);
Output:
1011.1
Another way to convert a decimal number to binary is to use a custom function that performs the conversion using mathematical operations.
Here’s an example of such a function:
function convertToBinary(x) {
let bin = 0;
let rem, i = 1, step = 1;
while (x != 0) {
rem = x % 2;
x = parseInt(x / 2);
bin = bin + rem * i;
i = i * 10;
}
return bin;
}
const number = 12;
const binary = convertToBinary(number);
console.log(binary);
This function takes a decimal number as input and returns its binary representation. It uses a while loop to repeatedly divide the input number by 2 and calculate the remainder.
The binary representation is built up by adding the remainders multiplied by increasing powers of 10
Output:
1100
Conclusion
In conclusion, this article provided a comprehensive overview of working with binary in JavaScript.
This article explained various methods to convert decimal numbers and strings to binary, including utilizing built-in functions like parseInt() and custom conversion functions.
Aside from that, we also discussed JavaScript’s binary operators and their role in performing binary computations.
We are hoping that this article provides you with enough information that helps you understand the binary in JavaScript.
If you want to dive into more JavaScript topics, check out the following articles:
Thank you for reading itsourcecoders 😊.