What is inheritance in Java?
Inheritance in Java is one of the most important parts of Object-Oriented Programming (OOP).
In Java, inheritance is the process of passing on the properties of one class to another.
For instance, the relationship between a father and a son is an inheritance.
Inheritance means taking on all of the behaviors of a parent object.
In addition, the idea of inheritance is that new classes can be built on top of older ones.
When you inherit from an existing class, you can use the methods and properties from the parent class.
You can also add more fields and methods to your existing class.
The extends keyword extends a class (indicates that a class is inherited from another class). For example,
class Animals { // methods and fields } // use of extends keyword // to perform inheritance class Horse extends Animals { // methods and fields of Animals // methods and fields of Horse }
In the above example, the Horse class is made by inheriting the methods and fields from the Animals class.
The subclass is the Horse, and the superclass is Animals.
Example:
// program created by Glenn
class Animals {
// field and method of the parent class
String name;
public void eat() {
System.out.println("I can write and program");
}
}
// inherit from Animals
class Horse extends Animals {
// new method in subclass
public void display() {
System.out.println("My name is " + name);
}
}
public class Main {
public static void main(String[] args) {
// create an object of the subclass
Horse ho = new Horse();
// access field of superclass
ho.name = "Glenn Magada Azuelo";
ho.display();
// call method of superclass
// using object of subclass
ho.eat();
}
}
Output:
My name is Glenn Magada Azuelo
I can write and program
In the above example, we used the superclass Animals to make a subclass called Dogs. Pay attention to the words example below,
ho.name = "Glenn Magada Azuelo"; ho.eat();
The ho is an object of Horse. But the Animals class has members named name and eat().
Since the Horse got the field and method from Animals, we can use the object of the Horse to get to the field and method.
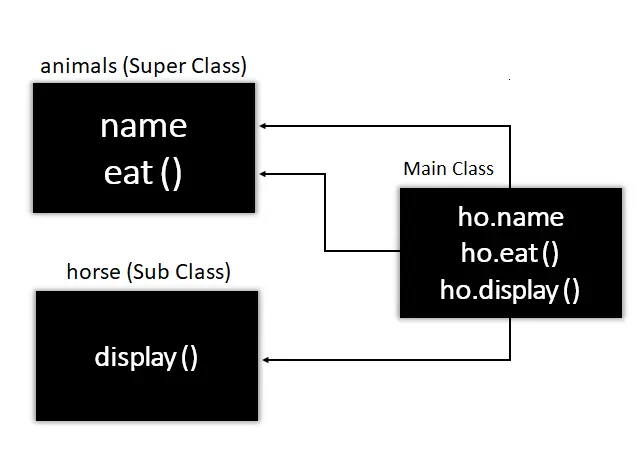
is-a relationship
In Java, inheritance is called a “is-a” relationship. That is, we only use inheritance if two classes have a “is-a” relationship.
For example:
- Apple is a Fruit
- Horse is an Animal
- A car is a Vehicle
The Car can inherit from the Vehicle class, just like the Apple can inherit from the Fruit class.
Method overriding Inheritance in Java
In Java, method overriding happens when a subclass (child class) has the same method as the parent class.
In other words, method overriding is when a subclass provides a different implementation of a method declared by one of its parent classes.
Example 1 shows that subclass objects can access superclass methods.
But what will happen if the same method is in both the superclass and the subclass?
In this case, the method in the subclass overwrites the method in the superclass.
This idea is called method overriding in Java.
Method Overriding Inheritance in Java Example Program
// program created by Glenn
class Animals {
// method in the superclass
public void eat() {
System.out.println("I can eat");
}
}
// Dog inherits Animal
class Horse extends Animals {
// overriding the eat() method
@Override
public void eat() {
System.out.println("I eat a lot of food");
}
// new method in subclass
public void kick() {
System.out.println("I am really full");
}
}
public class Main {
public static void main(String[] args) {
// create an object of the subclass
Horse ho = new Horse();
// call the eat() method
ho.eat();
ho.kick();
}
}
Output:
I eat a lot of food I am really full
In order for you to test the Java code provided in this lesson, you must test the code in your code editor.
But if you wish to run this code online, we also have an online compiler in Java for you to test your Java code for free.
You can test the above example here! ➡Java Online Compiler
In the above example, the superclass Animals and the subclass Horse have the eat() method.
Here, we’ve made a ho object of a Dog.
Now, when we use the object ho to call the method eat(), the method inside the object Horse is called.
The method in the derived class takes precedence over the method in the base class.
Super Keyword Inheritance in Java
The super keyword refers to the parent class of an object.
It is used to call superclass methods and to get to the superclass constructor.
The most common use of the super keyword is to avoid confusion between super classes and subclasses that have methods with the same name.
We have already seen that the same method in a subclass takes precedence over the same method in a superclass.
In this case, the method of the parent class is called from the method of the child class by using the super keyword.
Super Keyword Inheritance in Java Example Program
// program created by Glenn
class Animals {
// method in the superclass
public void eat() {
System.out.println("I can eat any kind of animals");
}
}
// Dog inherits Animal
class Horse extends Animals {
// overriding the eat() method
@Override
public void eat() {
// call method of superclass
super.eat();
System.out.println("I eat much food");
}
// new method in subclass
public void kick() {
System.out.println("I am really full");
}
}
public class Main {
public static void main(String[] args) {
// create an object of the subclass
Horse ho = new Horse();
// call the eat() method
ho.eat();
ho.kick();
}
}
Output:
I can eat any kind of animals
I eat much food
I am really full
You can test the above example here! ➡Java Online Compiler
In the above example, the eat() method is in both the base class Animals and the class Horse, which is derived from Animals. Notice the statement,
super.eat();
In this case, the super keyword is used to call the superclass’s eat() method.
Protected Members Inheritance in Java
A protected field or method in a public class can be accessed directly by all classes in the same package and its subclasses, even if the subclasses are in different packages.
It has fewer permissions than package or default access.
In Java, if a class has protected fields and methods, the subclass of the class can access these fields and methods.
Protected Members Inheritance in Java Example Program
// program created by Glenn
class Animals {
protected String name;
protected void display() {
System.out.println("I am Glenn Magada Azuelo.");
}
}
class Horse extends Animals {
public void getInfo() {
System.out.println("I " + name);
}
}
public class Main {
public static void main(String[] args) {
// create an object of the subclass
Horse ho = new Horse();
// access protected field and method
// using the object of subclass
ho.name = "am a Student and freelancer";
ho.display();
ho.getInfo();
}
}
Output:
I am Glenn Magada Azuelo.
I am a Student and freelancer
You can test the above example here! ➡Java Online Compiler
In the example above, we’ve made a class called Animal.
The class has a private field called name and a method called display().
The Dog class inherits the Animal class. Notice the statement,
ho.name = "am a Student and freelancer";
ho.display();
Here, we can access the protected field and method of the superclass by using the ho object of the subclass.
What are the advantages of inheritance in Java?
- The advantage of Inheritance it makes it easier to reuse code.
- The code defined in the parent class can be used by the child class without having to rewrite it.
- Inheritance can save time and effort because the main code doesn’t have to be written again.
Inheritance provides a clear model structure that is easy to understand.
Why do we need to use inheritance in Java?
- The most important thing you can do with inheritance in Java is reuse code. The code in the parent class can be used directly by the code in the child class.
- Runtime polymorphism is another name for method overriding. So, we can use inheritance to achieve polymorphism in Java.
What is inheritance in Java Real time example?
In Java, inheritance means that one class can use the features or properties of another class.
For example, we are people. Some of our abilities come from the class “Human,” like being able to talk, breathe, eat, drink, etc.
We can also use cars as an example.
What are the 5 types of inheritance in Java?
The following listed below are the 5 types of inheritance in Java
- Single inheritance
- Multi-level inheritance
- Hierarchical inheritance
- Multiple inheritance
- Hybrid inheritance
Single Inheritance in Java
The single inheritance, data, and methods are passed from a parent class to a single child class. In this case, a child class can use all of the parent class’s methods and variables.
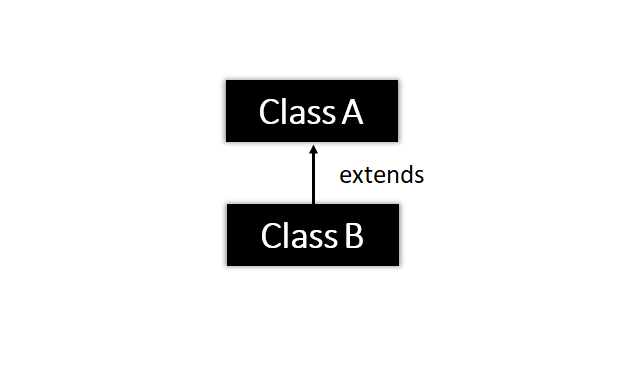
Single Inheritance in Java Example Program
// sample program by Glenn
class Father {
String familyName;
String houseaddress;
Father() {
familyName = "A Writer and Programmer";
houseaddress = "PH";
}
}
public class Son extends Father {
String name;
Son() {
name = "Glenn Magada Azuelo";
}
void printdetails() {
System.out.println("Hey my name is " + this.name + " " + this.familyName + " and I am from " + this.houseaddress);
}
public static void main(String[] args) {
Son s1 = new Son();
s1.printdetails();
}
}
Output:
Hey, my name is Glenn Magada Azuelo A Writer and Programmer and I am from PH\
You can test the above example here! ➡Java Online Compiler
Multi-level Inheritance in Java
The multi-level inheritance involves at least two and up to more than two classes.
One class gets its features from a parent class, and the newly made sub-class becomes the base class for another new class.
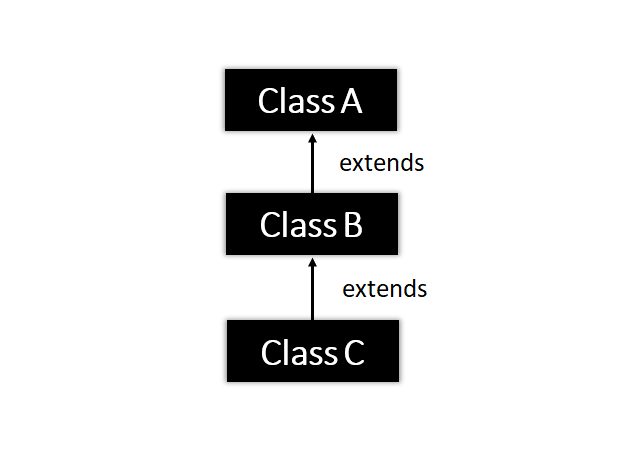
Multi-level Inheritance in Java Example Program
// program created by Glenn
class GrandFather {
GrandFather() {
System.out.println("I am the grandfather.");
}
}
class Father extends GrandFather {
String familyName;
String houseaddress;
Father() {
System.out.println("I am the father and i inherit from Grandfather.");
}
}
public class Son extends Father {
Son() {
System.out.println("I am the son and i inherit from my father.");
}
public static void main(String[] args) {
Son sOne = new Son();
}
}
Output:
I am the grandfather.
I am the father and i inherit from Grandfather.
I am the son and i inherit from my father.
You can test the above example here! ➡Java Online Compiler
Hierarchical Inheritance in Java
A Hierarchical inheritance is a type of inheritance in which multiple derived classes take on the properties of the same base class.
There are multiple child classes and only one parent class.
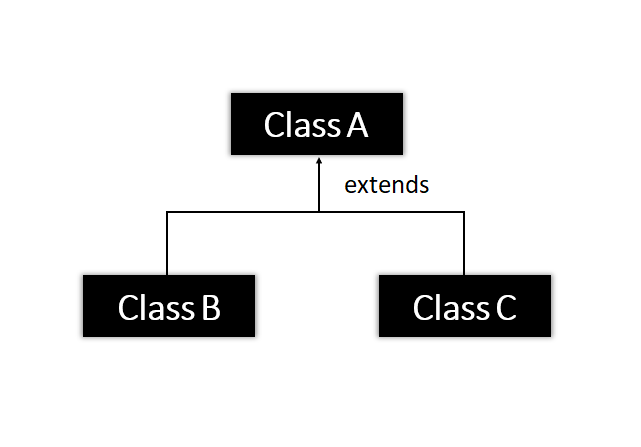
Hierarchical Inheritance in Java Example Program
// program created by Glenn
class Father {
String familyName;
String houseaddress;
Father() {
familyName = "Azuelo";
houseaddress = "PH";
}
}
class Son extends Father {
Son() {
System.out.println("I am Glenn Magada Azuelo");
System.out.println("My family name is " + this.familyName + " and I am from " + this.houseaddress);
}
}
class Daughter extends Father {
Daughter() {
System.out.println("I have 5 brothers and 1 sister");
System.out.println("My family name is " + this.familyName + " and I am from " + this.houseaddress);
}
}
public class Main {
public static void main(String[] args) {
Son s = new Son();
Daughter d = new Daughter();
}
}
Output:
I am Glenn Magada Azuelo
My family name is Azuelo and I am from PH
I have 5 brothers and 1 sister
My family name is Azuelo and I am from PH
You can test the above example here! ➡Java Online Compiler
Multiple Inheritance In Java
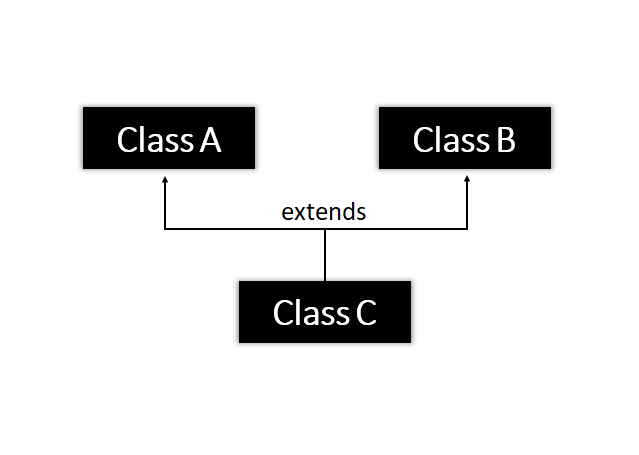
Java does not support multiple inheritance. This means a class cannot be extended by more than one class. So, the following is illegal
public class extends Animal, Mammal{}
But a class can implement one or more interfaces, so Java no longer makes it impossible to inherit from more than one class.
The extends keyword is used only once, and the parent interfaces are listed in a comma-separated list.
For example, if the Hockey interface extended both Sports and Event, it would be declared as –
public interface Hockey extends Sports, Event
Multiple Inheritance In Java Example Program
// program created by Glenn
interface Event {
public void start();
}
interface Sports {
public void play();
}
interface sepakTakraw extends Sports, Event{
public void show();
}
public class Tester{
public static void main(String[] args){
sepakTakraw sepak = new sepakTakraw() {
public void start() {
System.out.println("Start Event.");
}
public void play() {
System.out.println("Play Sports.");
}
public void show() {
System.out.println("Show Sepak Takraw.");
}
};
sepak.start();
sepak.play();
sepak.show();
}
}
Output:
Start Event.
Play Sports.
Show Sepak Takraw.
You can test the above example here! ➡Java Online Compiler
Hybrid Inheritance in Java
Hybrid inheritance is a combination of two or more types of inheritance.
The goal of using hybrid inheritance in Java is to break up the codebase into well-defined classes and make the code more reusable.
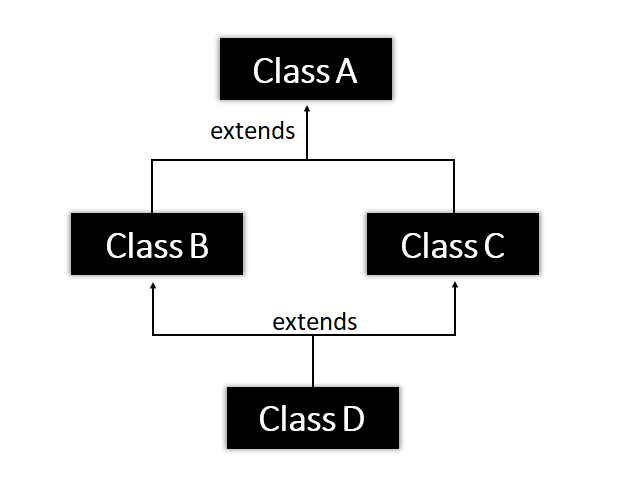
Hybrid Inheritance in Java Example Program
// program cretaed by Glenn
class A {
A() {
System.out.println("I am Glenn Magada Azuelo a Writer and Programmer");
}
}
class B extends A {
B() {
System.out.println("I am Jude Suarez a Writer and Programmer");
}
}
class C extends A {
C() {
System.out.println("I am Adonez Evangelista a Writer and Programmer");
}
}
class D extends B {
D() {
System.out.println("I am Caren Love from EnglishTutorHub");
}
}
public class Main {
public static void main(String[] args) {
D d = new D();
B b = new B();
C c = new C();
}
}
Output:
I am Glenn Magada Azuelo a Writer and Programmer
I am Jude Suarez a Writer and Programmer
I am Caren Love from EnglishTutorHub
I am Glenn Magada Azuelo a Writer and Programmer
I am Jude Suarez a Writer and Programmer
I am Glenn Magada Azuelo a Writer and Programmer
I am Adonez Evangelista a Writer and Programmer
You can test the above example here! ➡Java Online Compiler
Summary
In summary, you have learned about What Is Inheritance In Java.
This tutorial has covered the following topics: What Is Inheritance, Method Overriding, Super Keyword, Protected Members, advantages, 5 types of inheritance, Single Inheritance, Multi-level Inheritance, Hierarchical Inheritance, Multiple Inheritance and Hybrid Inheritance.
I hope this lesson has helped you learn a lot.