In this tutorial about Upload and Show Image Using PHP/MYSQL, you will learn on how to upload an image in the database and show it in a page after uploading the image.
First, create a database and name it as any name you desire. Then create a table, name it as “photos“.
[sql]CREATE TABLE `photos` ( `photo_id` int(11) NOT NULL, `photo_link` text NOT NULL ) ENGINE=MyISAM DEFAULT CHARSET=latin1;[/sql]
On the index page, put the following codes.
[php]<?php include 'connection.php'; ?>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Upload Photo in PHP & MYSQL</title>
</head>
<body>
<h3>Upload and Show Photo in PHP & MYSQL</h3>
<?php
if (isset($_GET['upload_action'])) {
if ($_GET['upload_action'] == "success") { ?>
<b>Photo Successfully Uploaded</b>
<?php }
}
?>
<form method="post" action="upload.php" enctype="multipart/form-data">
<label>Choose Photo:</label><br>
<input type="file" name="photo" /><br><br>
<input type="submit" value="Upload" />
</form><br>
<?php
$photos = $mysqli->query("SELECT * FROM photos");
?>
<b><?php echo $photos->num_rows ?></b> total photos uploaded<br><br>
<?php
while ($photo_data = $photos->fetch_assoc()) { ?>
<img src="<?php echo $photo_data['photo_link']; ?>" width="200px" height="200px" />
<?php }
?>
</body>
</html>[/php]
Create an “upload.php” file then put the following codes.
[php]<?php
include 'connection.php';
if ($_FILES['photo']['name'] != null) {
move_uploaded_file($_FILES['photo']['tmp_name'], "images/" . $_FILES['photo']['name']);
$img_link = "images/" . $_FILES['photo']['name'];
$upload = $mysqli->query("INSERT INTO photos (photo_link) VALUES ('$img_link')") or die($mysqli->error);
if ($upload) {
header("Location: index.php?upload_action=success");
} else if (!$upload) {
echo $mysqli->error;
}
} else if ($_FILES['photo']['name'] == null) {
echo 'Please choose a photo.';
}
?>[/php]
Screenshot:
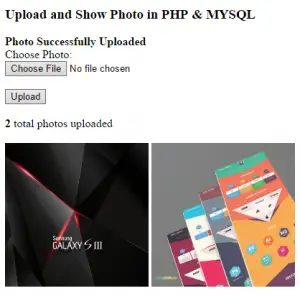
If you have any questions or suggestion about Upload and Show Image Using PHP/MYSQL, please feel free to contact us at our contact page.