Hello Guys! This tutorial is all about How to upload image easily in PHP using MySql. This Tutorial is very easy just follow my steps and you’re good to go!
First, we’re going to create a database that will store the location of our image.
1. Open PHPMyAdmin.
2. Create a database and name it as imagetb.
3. Create database connection and save it as “conn.php” put this code in conn.php
<?php $con = mysqli_connect("localhost","root","","imagetb"); // Check connection if (mysqli_connect_errno()) { echo "Failed to connect to MySQL: " . mysqli_connect_error(); } ?>
4.Then create a folder that will store our uploaded images. Name it as “Upload”.
5. Create a form and save it as “index.php” Put this cod in Index.php
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Easy and Simple Image Upload</title> </head> <body> <div> Uploaded Images: <?php include('conn.php'); $query=mysqli_query($con,"select * from image_tb"); while($row=mysqli_fetch_array($query)){ ?> <img src="<?php echo $row['img_location']; ?>"> <?php } ?> </div> <div> <form method="POST" action="upload.php" enctype="multipart/form-data"> <label>Image:</label><input type="file" name="image"> <button type="submit">Upload</button> </form> </div> </body> </html>
6. Lastly create a upload form and put this code.
<?php include('conn.php'); $fileinfo=PATHINFO($_FILES["image"]["name"]); $newFilename=$fileinfo['filename'] ."_". time() . "." . $fileinfo['extension']; move_uploaded_file($_FILES["image"]["tmp_name"],"upload/" . $newFilename); $location="upload/" . $newFilename; mysqli_query($con,"insert into image_tb (img_location) values ('$location')"); header('location:index.php'); ?>
<?php
include('conn.php');
$fileinfo=PATHINFO($_FILES["image"]["name"]);
$newFilename=$fileinfo['filename'] ."_". time() . "." . $fileinfo['extension'];
move_uploaded_file($_FILES["image"]["tmp_name"],"upload/" . $newFilename);
$location="upload/" . $newFilename;
mysqli_query($con,"insert into image_tb (img_location) values ('$location')");
header('location:index.php');
?>
7.Run and test the program afterwards!
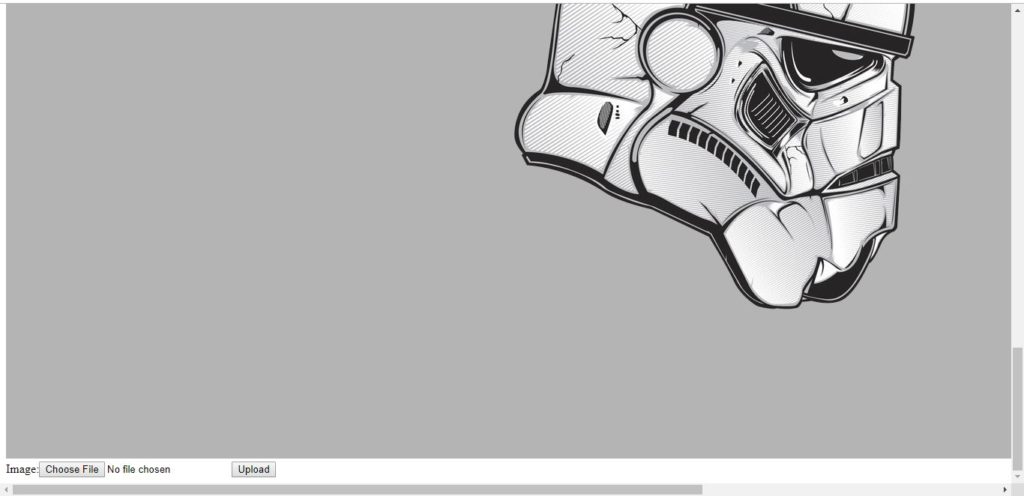
If you have any comments or suggestions about the How to upload image easily in PHP using MySql Please message us directly
Download Source code here:
Other Articles you might read also:
Hi,
I have also found one awesome free jewelry website template as the below link:
<a href="https://www.templateonweb.com/jeweler/free-14-258-download"