Good day, ITSOURCECODERS!
In this tutorial, I will show you a complete guide for beginners on JAVA classes, including the given source code and expected output.
So let’s get started!
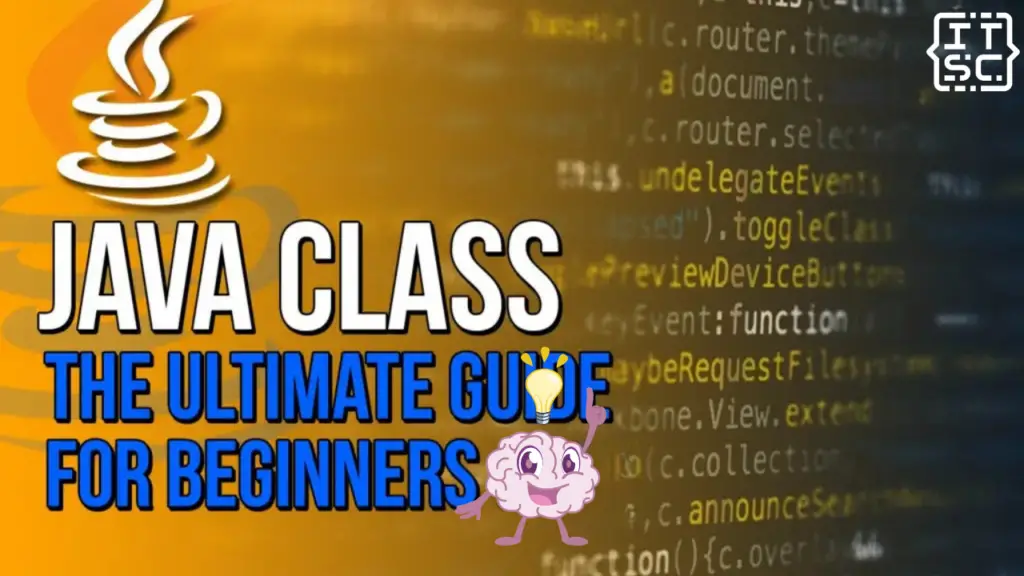
What is Java Class?
Java is a computer language that focuses on objects.
There are at least one class in every Java application you write.
A class is similar to an empty form.
To put it another way, a class is a broad description of anything.
Example of Class in Java?
A class in java is a blueprint from which individual objects are created.
Following is a sample of class:
public class Dog { String breed; int age; String color; void barking() { } void hungry() { } void sleeping() { } }
A class can contain any of the following variable types:
- Local Variables – Local variables are variables specified within methods, constructors, or blocks.
The variable will be declared and initialized within the method, and then removed once the method is finished. - Instance Variables – Instance variables are variables that exist within a class but are not associated with any methods.
When the class is created, these variables are set to their default values.
Instance variables can be accessed from within any of the class’s methods, constructors, or blocks. - Class Variables – Class variables are variables declared with the static keyword within a class, outside of any methods.
A class can have as many methods as it wants to retrieve the value of different types of methods.
Barking(), hungry(), and sleeping() are methods in the sample above.
The following are some of the most important issues to consider when studying Java classes.
Java Class Static
The keyword static can be used with variables, classes, blocks, and methods in Java.
When we put the static keyword before any of them, we’re indicating that the specified member is a member of the type.
To put it another way, a static member instance is created and shared across all instances of the class.
Can a class be static in java?
Yes, it is possible to make some Java classes static. Static Instance Variables, Static Methods, Static Blocks, and Static Classes are all supported by Java.
A class can be defined within another class in Java. Nested Classes are what they’re called. The Outer Class is the class in which the nested class is declared.
Inner classes, unlike top-level classes, can be static. Inner classes are non-static nested classes that aren’t static.
Without an instance of the outer class, an instance of the inner class cannot be created.
As a result, without utilizing a reference to the outer class instance, an inner class instance can access all of the members of its outer class.
As a result, inner classes can aid in the creation of clear and concise applications.
What are the differences between static and non-static nested classes?
- Static – It is possible to instantiate a static nested class without first instantiating its outer class.
- Non-Static – Inner classes have access to the outer class’s static and non-static members. Only the static members of the outer class are accessible to a static class.
class OuterClass { private static String msg = "IT SOURCECODE"; // Static nested class public static class NestedStaticClass { // Only static members of Outer class // is directly accessible in nested // static class public void printMessage() { // Try making 'message' a non-static // variable, there will be compiler error System.out.println( "Message from nested static class: " + msg); } } // Non-static nested class - // also called Inner class public class InnerClass { // Both static and non-static members // of Outer class are accessible in // this Inner class public void display() { System.out.println( "Message from non-static nested class: " + msg); } } } class Main { // How to create instance of static // and non static nested class? public static void main(String args[]) { // Create instance of nested Static class OuterClass.NestedStaticClass printer = new OuterClass.NestedStaticClass(); // Call non static method of nested // static class printer.printMessage(); // In order to create instance of // Inner class we need an Outer class // instance. Let us create Outer class // instance for creating // non-static nested class OuterClass outer = new OuterClass(); OuterClass.InnerClass inner = outer.new InnerClass(); // Calling non-static method of Inner class inner.display(); // We can also combine above steps in one // step to create instance of Inner class OuterClass.InnerClass innerObject = new OuterClass().new InnerClass(); // Similarly we can now call Inner class method innerObject.display(); } }
Expected Output
Message from nested static class: IT SOURCECODE Message from non-static nested class: IT SOURCECODE Message from non-static nested class: IT SOURCECODE
Java Class Path
What is java class path?
The location of user-defined classes and packages is specified by the classpath argument in the Java Virtual Machine or the Java compiler.
The parameter can be set by a command-line argument or an environment variable.
How to set classpath in java?
The CLASSPATH variable specifies the place where all of the application’s needed files can be found. CLASSPATH is used by the Java Compiler and JVM (Java Virtual Machine) to locate necessary files. If the CLASSPATH variable is not set, the Java Compiler will be unable to locate the relevant files, resulting in the following error.
Error: Could not find or load main class <class name> (e.g. ITSC)
The above error is resolved when classpath is set.
// If the following code is run when the CLASSPATH is not // set, it will throw the above error. // If it is set, we get the desired result import java.io.*; class ITSC { public static void main(String[] args) { // prints IT SOURCECODE to the console System.out.println("IT SOURCECODE!"); } }
Output:
IT SOURCECODE!
Java Class String
Character strings are represented by the String class. This class is used to implement all string literals in Java programs, such as “abc.” Strings are immutable; once formed, their values cannot be modified. Mutable strings are supported via string buffers. String objects can be shared because they are immutable.
Creating a String
String greeting = "Hello world!";
The compiler produces a String object with the value “Hello world!” whenever it sees a string literal in your code.
String objects are created using the new keyword and a constructor, just like any other object. The String class includes 11 constructors that allow you to provide the string’s initial value from various sources, such as an array of characters.
Example:
public class StringDemo { public static void main(String args[]) { char[] helloArray = { 'h', 'e', 'l', 'l', 'o', '.' }; String helloString = new String(helloArray); System.out.println( helloString ); } }
Output:
hello.
Java Class Scanner
The Scanner class, which is part of the java.util package, is used to gather user input.
To use the Scanner class, create an object of the class and call any of the methods listed in the documentation for the Scanner class.
The nextLine() method, which is used to read Strings, will be utilized in our example:
Example:
import java.util.Scanner; // Import the Scanner class class Main { public static void main(String[] args) { Scanner myObj = new Scanner(System.in); // Create a Scanner object System.out.println("Enter username"); String userName = myObj.nextLine(); // Read user input System.out.println("Username is: " + userName); // Output user input } }
Output:
Enter username jude Username is: jude
Abstract Class vs Interface Java
An abstract class allows you to create functionality that subclasses can implement or override, whereas an interface allows you to state but not implement functionality.
While a class can only extend one abstract class, it can implement several interfaces.
Parameters | Interface | Abstract class |
---|---|---|
Speed | Slow | Fast |
Multiple Inheritances | Implement several Interfaces | Only one abstract class |
Structure | Abstract methods | Abstract & concrete methods |
When to use | Future enhancement | To avoid independence |
Inheritance/ Implementation | A Class can implement multiple interfaces | The class can inherit only one Abstract Class |
Default Implementation | While adding new stuff to the interface, it is a nightmare to find all the implementors and implement newly defined stuff. | In case of Abstract Class, you can take advantage of the default implementation. |
Access Modifiers | The interface does not have access modifiers. Everything defined inside the interface is assumed public modifier. | Abstract Class can have an access modifier. |
When to use | It is better to use interface when various implementations share only method signature. Polymorphic hierarchy of value types. | It should be used when various implementations of the same kind share a common behavior. |
Data fields | the interface cannot contain data fields. | the class can have data fields. |
Multiple Inheritance Default | A class may implement numerous interfaces. | A class inherits only one abstract class. |
Implementation | An interface is abstract so that it can’t provide any code. | An abstract class can give complete, default code which should be overridden. |
Use of Access modifiers | You cannot use access modifiers for the method, properties, etc. | You can use an abstract class which contains access modifiers. |
Usage | Interfaces help to define the peripheral abilities of a class. | An abstract class defines the identity of a class. |
Defined fields | No fields can be defined | An abstract class allows you to define both fields and constants |
Inheritance | An interface can inherit multiple interfaces but cannot inherit a class. | An abstract class can inherit a class and multiple interfaces. |
Constructor or destructors | An interface cannot declare constructors or destructors. | An abstract class can declare constructors and destructors. |
Limit of Extensions | It can extend any number of interfaces. | It can extend only one class or one abstract class at a time. |
Abstract keyword | In an abstract interface keyword, is optional for declaring a method as an abstract. | In an abstract class, the abstract keyword is compulsory for declaring a method as an abstract. |
Class type | An interface can have only public abstract methods. | An abstract class has protected and public abstract methods. |
Example Code:
Following is sample code to create an interface and abstract class in Java
Interface Syntax
interface name{ //methods }
Java Interface Example
interface Pet { public void test(); } class Dog implements Pet { public void test() { System.out.println("Interface Method Implemented"); } public static void main(String args[]) { Pet p = new Dog(); p.test(); } }
Abstract Class Syntax
abstract class name{ // code }
Abstract Class Example
abstract class Shape { int b = 20; abstract public void calculateArea(); } public class Rectangle extends Shape { public static void main(String args[]) { Rectangle obj = new Rectangle(); obj.b = 200; obj.calculateArea(); } public void calculateArea() { System.out.println("Area is " + (b * b)); } }
Java Class Math
For performing simple math operations on Java variables, Java includes a collection of built-in math operators.
The Java math operators are straightforward. As a result, Java includes the Java Math class, which includes methods for conducting more complicated math computations.
This Java math tutorial will go through the math operators in Java as well as the Java Math class.
Java Math Operators
Let me begin by explaining the four basic Java math operators. The following are the Java math operators:
Math Operator | Description |
+ | Performs addition |
- | Performs subtraction |
* | Performs multiplication |
/ | Performs division |
Addition
The +
operator performs an addition of two values.
This can be an addition of two constants, a constant and a variable, or a variable and a variable. Here are a few Java addition examples:
int sum1 = 10 + 20; // adding two constant values int sum2 = sum1 + 33; // adding a variable and a constant int sum3 = sum2 + sum2; // adding two variables
The +
operator will replace the two values with the sum of the two values at runtime.
So at the place where the expression sum1 + 33
is written, at runtime this will be replaced with the sum of the value of sum1
and the constant value 33
.
You can of course create longer chains of additions and thus add more than two numbers.
Here is a en example of adding 3 or more values together:
int sum1 = 10 + 20 + 30; int sum3 = sum2 + 99 + 123 + 1191;
A commonly used math operation on variables is to set the variable equal to its own value plus another value. Here is how that looks:
int result = 10; result = result + 20;
The second line of this example sets the sum
variable equals to its own value (before being assigned the new value) + 20. Which means 10 + 20.
Since adding a value to a variable is a very common operation, Java contains a built-in operator for that specific purpose.
It is the +=
operator. Here is the example above rewritten to use the +=
operator:
int result = 10; result += 20;
The second line in this example adds 20 to the variable result
. This equivalent to the code result = result + 20;
Subtraction
The – operator performs subtraction of one value from another. This can be a subtraction of a constant value from another constant value, a constant value from a variable, or a variable from a variable. Here are a few Java subtraction examples:
int diff1 = 200 - 10; int diff2 = diff1 - 5; int diff3 = diff1 - diff2;
The -
operator will replace the two values with the difference between the two values, just like with the +
operator.
You can create longer chains of subtractions and thus subtract more values from each other. Here is an example of subtracting two values from another in a single Java statement:
int diff = 200 - 10 - 20;
This works for variables too.
Remember, minus minus equals plus, just like in normal math. Here is an example of that:
int diff = 200 - (-10);
After executing this math expression the diff
variable will contain the value 210
because - (-10)
is equal to +10
according to standard math rules.
Just like with addition, Java contains an operator specifically targeted at subtracting a value from a variable, and assigning the result to the variable. Here is first how that would look in Java without this operator:
int result = 200; result = result - 10;
Here is how the same math operation looks with the -=
operator:
int result = 200; result -= 10;
Multiplication
The *
operator performs multiplication of two values. The values can be either two constant values, a variable and a constant value, or two variables. Here are a few Java multiplication examples:
int prod1 = 10 * 20; int prod2 = prod1 * 5; int prod3 = prod1 * prod2;
The *
operator will replace the multiplication math expression with the product of the two values at runtime.
You can create longer chains of multiplication using the *
operator. Here is an example of multiplying 3 values with each other:
int prod = 10 * 20 * 30;
Multiplying a variable with a value and assigning the value back to the variable is a common math operation in Java applications. Therefore Java contains an explicit operator for this operation. The *=
operator. Here is first how the calculation would look without the *=
operator:
int result = 10; result = result * 20;
The second line of this example multiplies the value of the result
variable (before being assigned the result of this calculation) with 20 and assigns the value back to the result
variable.
Here is how the same math operation looks with the +=
operator:
int result = 10; result *= 20;
Division
The /
operator performs division of one value by another. This can be dividing one constant with another, dividing a variable with a constant, or dividing one variable by another variable. Here are a few Java division examples:
int division1 = 100 / 10; int division2 = division1 / 2; int division3 = division1 / division2;
You can chain multiple values for division, but because of the Java math operator precedence (explained later) you have to pay attention to how you chain the values.
Look at this division example:
int division = 100 / 10 / 2;
After executing this math expression the division
variable will contain the value 5. That is the result because the math expression was calculated by first dividing 100 by 10 (= 10) and then 10 by 2 (=5).
But, what if you had wanted to first divide the 10 by 2 (=5), and then divide the 100 by 5 (=20) ? You would have had to use parentheses to achieve that, like this:
int division = 100 / (10 / 2)
You will learn more about operator precedence and parentheses later in this Java math tutorial.
Java also contains a shortcut operator for dividing the value of a variable by another value, and assigning that value back to the variable.
The /=
operator. Here is first how that math operation looks in Java without the /=
operator:
int result = 100; result = result / 5;
The second line of this example divides the value of the result
variable (the value it had before being assigned the result of this calculation) by 5, and assigns the result back to the result
variable.
Here is how the same math operation looks with the /=
operator:
int result = 100; result /= 5;
Java Class Random
In Java, the Random class is used to produce pseudo-random numbers. This class’s instance is thread-safe.
This class’s instance, on the other hand, is cryptographically unsafe. This class has methods for generating various random data types such as float, double, and int.
- Random(): Creates a new random number generator
- Random(long seed): Creates a new random number generator using a single long seed
Example Code:
// Java program to demonstrate // method calls of Random class import java.util.Random; public class Test { public static void main(String[] args) { Random random = new Random(); System.out.println(random.nextInt(10)); System.out.println(random.nextBoolean()); System.out.println(random.nextDouble()); System.out.println(random.nextFloat()); System.out.println(random.nextGaussian()); byte[] bytes = new byte[10]; random.nextBytes(bytes); System.out.printf("["); for(int i = 0; i< bytes.length; i++) { System.out.printf("%d ", bytes[i]); } System.out.printf("]\n"); System.out.println(random.nextLong()); System.out.println(random.nextInt()); long seed = 95; random.setSeed(seed); // Note: Running any of the code lines below // will keep the program running as each of the // methods below produce an unlimited random // values of the corresponding type } }
Output:
4 true 0.19674934340402916 0.7372021 1.4877581394085997 [-44 75 68 89 81 -72 -1 -66 -64 117 ] 158739962004803677 -1344764816
END…
Summary
In Java, a class describes an object’s properties, which are called fields, as well as what the object can perform, which are called methods.
Classes can also have constructors, which set the values of fields, and a main method, which is where the execution begins.
Anyway, if you want to level up your programming knowledge, especially Java, try this new article I’ve made for you Best Java Projects With Source Code For Beginners Free Download.
Inquiries
If you have any questions or suggestions about Java Class: The Ultimate Guide For Beginners, please feel free to leave a comment below.