What is Recursion in C?
- Recursion C is the procedure created when a function calls a duplicate of itself to work on a smaller issue.
- Recursive functions in C, which include all functions that call themselves, are also known as recursive calls.
- Recursion can be used to solve problems with sorting, searching, and traversal, for instance.
- Numerous recursive calls are made during recursion.
- It’s crucial to impose a recursion termination condition, though. Although iterative code is longer, recursive code is more complex to understand.
- C program on recursion does not apply to all problems, but it works best for those that can be broken down into related subtasks.
In a simple understanding, Recursion in C is the process of a function calling a copy of itself. To put it briefly, recursion is the process of a function calling itself.
Syntax of recursive function in C
void recursion() { ************* recursion(); ************* } int main() { ************* recursion(); ************* }
How Recursion Works in C?
Here is a diagram showing how recursion operates:
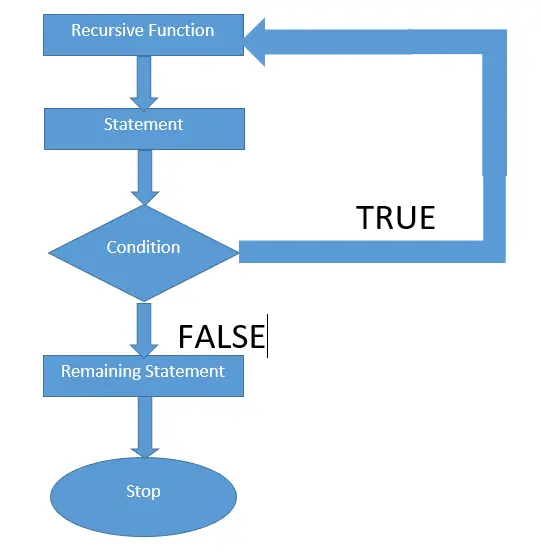
Example 1: Infinite loop through recursive function
#include<stdio.h> int main() { printf("C programming Tutorial: Infinite loop through Recursive Function!\n"); main(); return 0; }
Output of the above example:
C programming Tutorial: Infinite loop through Recursive Function!
C programming Tutorial: Infinite loop through Recursive Function!
The example given above will produce an endless loop. By invoking main() from main in the program mentioned above, we are performing recursion ().
Additionally, we did not set any requirements for the program to end. Because of this, the output will print indefinitely.
You can test the above example here! ➡ C Online Compiler
Types of Recursion C
The C programming language supports two different types of recursion.
- Direct Recursion
- Indirect Recursion
Direct Recursion C
Direct recursive functions are those that make direct calls to themselves.
Example 2: Direct Recursive function in C
int fib(int number) { if (number==1 || number==2) return 1; else return (fib(number-1)+fib(number-2)); }
The fib() method is directly recursive in the case above. because the fib() method is directly called by the statements inside of it.
Indirect C Recursion
An indirect recursive function is one that does not explicitly call itself before calling another function.
Example 3: Indirect Recursive function in C
int recursive_function(int number) { if (number<=2) return 2; else return newRecursive(number); } int newRecursive(int number) { return recursive_function(number); }
Example 4: Finding factorial of a given number
#include <stdio.h> int factorial(int); int main() { int number=5,fact; printf("C Programming Tutorial: Factorial of a number using recursion!\n\n"); fact = factorial(number); printf("Factorial of %d is: %d\n",number,fact); } int factorial(int number) { if (number==0) { return 0; } else if (number==1) { return 1; } else { return number*factorial(number-1); } }
Output of the above example:
C Programming Tutorial: Factorial of a number using recursion!
Factorial of 5 is: 120
You can test the above example here! ➡ C Online Compiler
What is a Recursive Function in C
Recursive functions in C carry out tasks by breaking them down into smaller ones.
The function has a defined termination condition, which is met by a certain subtask.
The recursion ends at this point, and the function returns the ultimate outcome.
The following pseudocode can be used to write any recursive function.
if (example_recursive) { return some_value; } else if (test_for_another_recursive) { return some_another_recursive; } else { // Statements; recursive call; }
Recursion C Examples
Here is an illustration of how to determine the nth term in the Fibonacci series.
#include<stdio.h> int fibonacci(int); void main () { int x,y; printf("Enter the value of n?"); scanf("%d",&x); y = fibonacci(x); printf("%d",y); } int fibonacci (int x) { if (x==0) { return 0; } else if (x == 1) { return 1; } else { return fibonacci(x-1)+fibonacci(x-2); } }
Output of the above example:
Enter the value of n? 12
144
You can test the above example here! ➡ C Online Compiler
Advantages of Recursion C
- creates an attractive program.
- The program code becomes more understandable, and the writing of the code takes less time.
- eases the intricacy of time.
- For challenges based on tree architectures, it works well.
Disadvantages of Recursion C
- Due to the expense of maintaining the stack, it runs slower than non-recursive programs.
- For the stack, additional RAM is needed.
- Instead of recursion, utilize loops for greater speed. so recursion moves more slowly.
Significance of Recursion C
Because recursion helps make C programs short and simple, programmers frequently utilize it in their work. It makes the code easier to read.
The run-time of the program code is also decreased with the aid of recursion.
However, iteration is another option that offers functionality that is significantly faster than recursion.
Recursion C is a powerful tool for solving a variety of issues.
Recursion can be used to quickly handle a variety of issues, including the factorial of a number, fibonacci series in a specific range, tree algorithm issues, and more.
Difference between tailed and non-tailed recursion C
A recursive function is referred to be tail-recursive if the recursive call is the final operation carried out by the function.
If not, it is referred to as a non-tailed recursion.
Summary
We studied recursion and recursive functions in this course. Recursion’s benefits and drawbacks were also covered.
The several C recursion types were also covered.
About Next Tutorial
In the following post, I’ll create a Variable Argument in C and try to explain its many components in full detail.
I hope you enjoy this post on C Recursion, in which I attempt to illustrate the language’s core grammar.
PREVIOUS