This article will give you the list of useful Java syntax cheat sheet every developer should know before doing the actual java programming.
What is Java?
Java is the high-level, object-oriented, robust, secure programming language, platform-independent, high-performance, Multi-threaded, and portable programming language. It was developed by James Gosling in June 1991. It can also be known as the platform as it provides its own JRE and API.
Table of contents
Java programming popularity
Jonathan Sandals in his post about Top 7 Programming Languages Of 2020 last February 7, 2020, listed that Java is being in the Top 2 Most programming language in the year 2020. The philosophy “Write once, work anywhere,” it’s very popular with businesses looking to make sure their applications have a consistent user experience. Java programs should be able to run over a network, without it mattering what operating system the user is running. based on the article published by Northeastern University Graduate programs about THE 10 MOST POPULAR PROGRAMMING LANGUAGES TO LEARN IN 2021 said that there are 29,000 jobs with an annual average salary of $104,000.
Java Systax
Here is the following Java syntax that may help you during your programming journey.
The Java code below called Main.java
, and this code will print “Hello World” to the screen:
public class Main {
public static void main(String[] args) {
System.out.println("Hello World");
}
}
Single-line Comments
// This is a comment
System.out.println("Hello World");
Java Multi-line Comments
/* The code below will print the words Hello World
to the screen, and it is amazing */
System.out.println("Hello World");
Java Variables
Variables are used to store values in computer memory. See the list below of the commonly used java variables
String
– store values such text, like as “Hello”. String values are surrounded by double quotesint
– stores integers values such (whole numbers), without decimals, like as 123 or -123float
– stores values such floating point numbers, with decimals, like as 19.99 or -19.99char
– stores values such single characters, such as ‘a’ or ‘B’. Char values are surrounded by single quotesboolean
– stores values with two states: Yes or No, On or Off, true or false
See the code below to declare java variables.
type variableName = value;
See the code below on how to assign value to java variables using different data types.
int myNum = 5;
float myFloatNum = 5.99f;
char myLetter = 'D';
boolean myBool = true;
String myText = "Hello";
Java Operators
In Java, operators are divided into the following groups.
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise operators
Arithmetic operators
Operator | Name | Description | Example |
+ | Addition | Adds together two values | x + y |
– | Subtraction | Subtracts one value from another | x – y |
* | Multiplication | Multiplies two values | x * y |
/ | Division | Divides one value by another | x / y |
% | Modulus | Returns the division remainder | x % y |
++ | Increment | Increases the value of a variable by 1 | ++x |
— | Decrement | Decreases the value of a variable by 1 | –x |
Sample Syntax using Arithmetic Operators
int sum1 = 150 + 10; // 160 (100 + 50)
int sum2 = sum1 + 300; // 460 (150 + 250)
int sum3 = sum2 + sum2; // 920 (460 + 460)
Assignment operators
Operator | Example | Same As |
= | x = 5 | x = 5 |
+= | x += 3 | x = x + 3 |
-= | x -= 3 | x = x – 3 |
*= | x *= 3 | x = x * 3 |
/= | x /= 3 | x = x / 3 |
%= | x %= 3 | x = x % 3 |
&= | x &= 3 | x = x & 3 |
|= | x |= 3 | x = x | 3 |
^= | x ^= 3 | x = x ^ 3 |
>>= | x >>= 3 | x = x >> 3 |
<<= | x <<= 3 | x = x << 3 |
The code below shows how to assign a value to a variable.
int x = 10;
Comparison operators
Operator | Name | Example |
== | Equal to | x == y |
!= | Not equal | x != y |
> | Greater than | x > y |
< | Less than | x < y |
>= | Greater than or equal to | x >= y |
<= | Less than or equal to | x <= y |
See the example code below showing the Comparison operators in java.
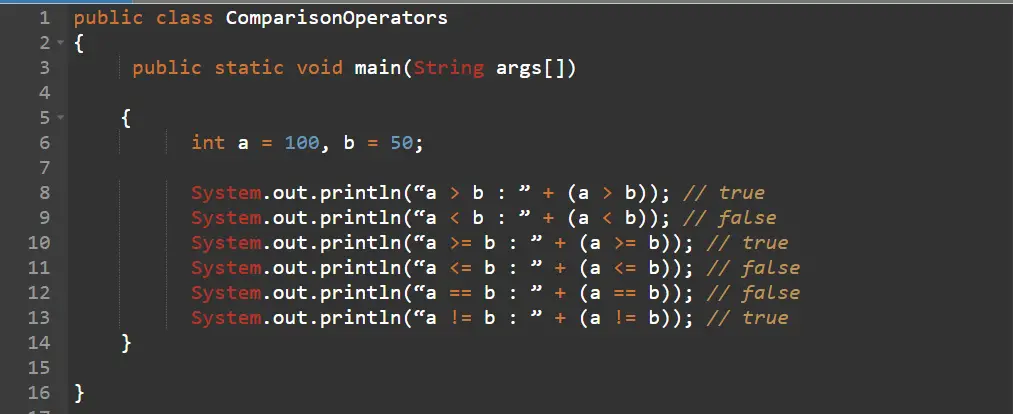
Logical operators
Operator | Name | Description | Example |
&& | Logical and | Returns true if both statements are true | x < 5 && x < 10 |
|| | Logical or | Returns true if one of the statements is true | x < 5 || x < 4 |
! | Logical not | Reverse the result, returns false if the result is true | !(x < 5 && x < 10) |
See the sample program below that shows logical operators in Java.
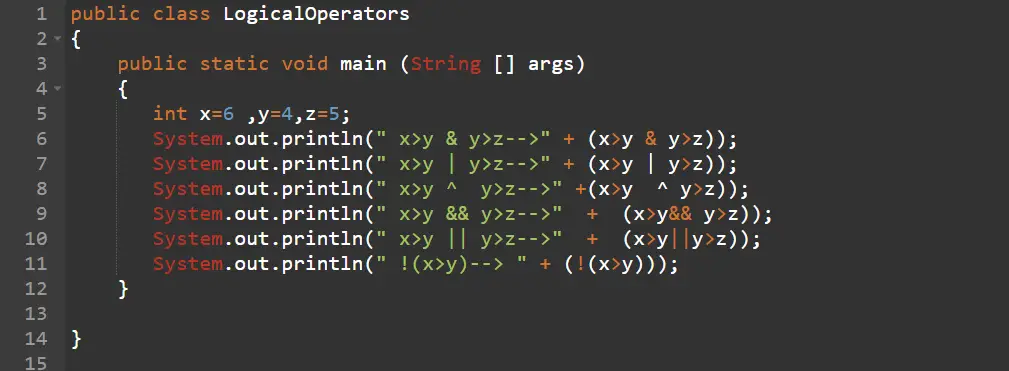
Java Conditions and If Statements
In Java, the if statement is used to evaluate the condition. It usually check the boolean condition, either true or false.
Here are the various types of if statement in Java.
The if Statement

The else Statement

The else if Statement

Short Hand If…Else (Ternary Operator)
variable = (condition) ? expressionTrue : expressionFalse;
Java Switch Statements
The switch
statement allows us to execute a block of code among many alternatives.
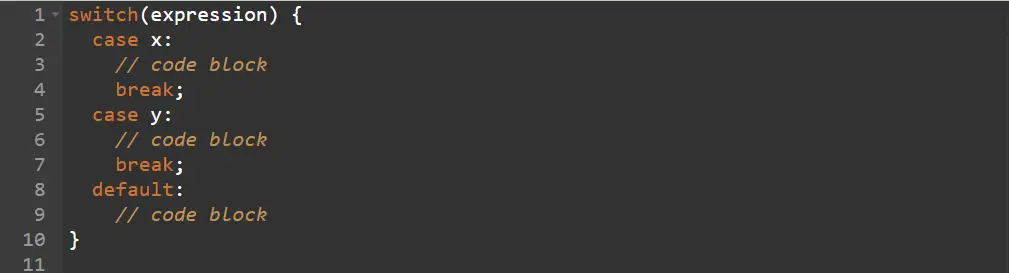
Java Loops
The Loops in Java is used to execute a block of code as long as a specified condition is reached. See the different types of loops in Java below.
Java While Loop

The Do/While Loop

Java For Loop

For-Each Loop
