How to Add and Retrieve Multiple Images in VB.Net
In this tutorial, you will learn how to add and retrieve multiple images in VB.Net.
First, create your project and add these tools on your blank project. (see image below.)
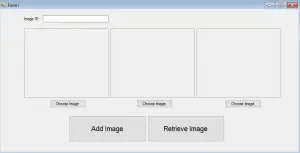
Then, create a database named retrieveimage then add this code to add image table with its columns.
[mysql]
CREATE TABLE IF NOT EXISTS `image` (
`ID` int(10) NOT NULL,
`image1` longblob NOT NULL,
`image2` longblob NOT NULL,
`image3` longblob NOT NULL,
PRIMARY KEY (`ID`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8;
[/mysql]
Then on our “choose image” button, insert this line of code:
[vbnet]
Try
Dim OFD As FileDialog = New OpenFileDialog()
OFD.Filter = “Image File (*.jpg;*)|*.jpg;*”
If OFD.ShowDialog() = DialogResult.OK Then
imgpath = OFD.FileName
PictureBox1.ImageLocation = imgpath
End If
OFD = Nothing
Catch ex As Exception
MsgBox(ex.Message.ToString())
End Try
[/vbnet]
For the next “choose image” button just change the name of PictureBox depending on its upper picture box name.
Add this line of code at the top of your Form1 code:
[vbnet]
Imports MySql.Data.MySqlClient
Dim imgpath As String
Dim arrImage() As Byte
Dim arrImage2() As Byte
Dim arrImage3() As Byte
Dim conn As MySqlConnection
Dim cmd As MySqlCommand
Dim da As MySqlDataAdapter
Dim sql As String
Dim result As Integer
[/vbnet]
Next, on the “Add Image” button, insert this code:
[vbnet]
Private Sub Button4_Click(sender As Object, e As EventArgs) Handles Button4.Click
If TextBox1.Text = “” Then
MsgBox(“Input ID!”)
ElseIf IsNothing(PictureBox1.Image) Then
MsgBox(“Select image 1!”)
ElseIf IsNothing(PictureBox2.Image) Then
MsgBox(“Select image 2!”)
ElseIf IsNothing(PictureBox3.Image) Then
MsgBox(“Select image 3!”)
Else
Try
Dim mstream As New System.IO.MemoryStream()
PictureBox1.Image.Save(mstream, System.Drawing.Imaging.ImageFormat.Jpeg)
arrImage = mstream.GetBuffer()
Dim FileSize As UInt32
FileSize = mstream.Length
mstream.Close()
conn = New MySqlConnection
conn.ConnectionString = “server=localhost; user id=root; password=; database=retrieveimage;”
conn.Open()
sql = “INSERT INTO image (`ID`, `image1`) VALUES (@ID, @Image1);”
cmd = New MySqlCommand
With cmd
.Connection = conn
.CommandText = sql
.Parameters.Clear()
.Parameters.AddWithValue(“@ID”, TextBox1.Text)
.Parameters.AddWithValue(“@Image1”, arrImage)
.ExecuteNonQuery()
End With
Catch ex As MySqlException
MsgBox(ex.Message)
Finally
conn.Close()
Try
Dim mstream As New System.IO.MemoryStream()
PictureBox2.Image.Save(mstream, System.Drawing.Imaging.ImageFormat.Jpeg)
arrImage2 = mstream.GetBuffer()
Dim FileSize As UInt32
FileSize = mstream.Length
mstream.Close()
conn = New MySqlConnection
conn.ConnectionString = “server=localhost; user id=root; password=; database=retrieveimage;”
conn.Open()
sql = “UPDATE image SET image2 = @Image2 WHERE ID = @ID;”
cmd = New MySqlCommand
With cmd
.Connection = conn
.CommandText = sql
.Parameters.Clear()
.Parameters.AddWithValue(“@ID”, TextBox1.Text)
.Parameters.AddWithValue(“@Image2”, arrImage2)
.ExecuteNonQuery()
End With
Catch ex As MySqlException
MsgBox(ex.Message)
Finally
conn.Close()
Try
Dim mstream As New System.IO.MemoryStream()
PictureBox3.Image.Save(mstream, System.Drawing.Imaging.ImageFormat.Jpeg)
arrImage3 = mstream.GetBuffer()
Dim FileSize As UInt32
FileSize = mstream.Length
mstream.Close()
conn = New MySqlConnection
conn.ConnectionString = “server=localhost; user id=root; password=; database=retrieveimage;”
conn.Open()
sql = “UPDATE image SET image3 = @Image3 WHERE ID = @ID;”
With cmd
.Connection = conn
.CommandText = sql
.Parameters.Clear()
.Parameters.AddWithValue(“@ID”, TextBox1.Text)
.Parameters.AddWithValue(“@Image3”, arrImage3)
result = .ExecuteNonQuery()
End With
Catch ex As MySqlException
MsgBox(ex.Message)
Finally
conn.Close()
If result = 0 Then
MsgBox(“Error in registering!”)
Else
MsgBox(“Successfully added images!”)
End If
TextBox1.Text = “”
PictureBox1.Image = Nothing
PictureBox2.Image = Nothing
PictureBox3.Image = Nothing
End Try
End Try
End Try
End If
End Sub
[/vbnet]
To retrieve our images from the database, double click the “Retrieve Image” button and insert this code.
[vbnet]
Private Sub Button5_Click(sender As Object, e As EventArgs) Handles Button5.Click
If TextBox1.Text = “” Then
MsgBox(“Input ID for retrieval!”)
Else
Try
conn = New MySqlConnection
conn.ConnectionString = “server=localhost; user id=root; password=; database=retrieveimage;”
conn.Open()
sql = “SELECT * FROM image WHERE ID = @ID;”
cmd = New MySqlCommand
With cmd
.Connection = conn
.CommandText = sql
.Parameters.Clear()
.Parameters.AddWithValue(“@ID”, TextBox1.Text)
.ExecuteNonQuery()
End With
Dim arrImage(), arrImage2(), arrImage3() As Byte
Dim dt As New DataTable
da = New MySqlDataAdapter
da.SelectCommand = cmd
da.Fill(dt)
If dt.Rows.Count > 0 Then
MsgBox(“Showing results!”)
arrImage = dt.Rows(0).Item(1)
arrImage2 = dt.Rows(0).Item(2)
arrImage3 = dt.Rows(0).Item(3)
Dim mstream As New System.IO.MemoryStream(arrImage)
Dim mstream2 As New System.IO.MemoryStream(arrImage2)
Dim mstream3 As New System.IO.MemoryStream(arrImage3)
PictureBox1.Image = Image.FromStream(mstream)
PictureBox2.Image = Image.FromStream(mstream2)
PictureBox3.Image = Image.FromStream(mstream3)
Else
MsgBox(“No results!”)
End If
Catch ex As MySqlException
MsgBox(ex.Message)
Finally
conn.Close()
da.Dispose()
End Try
End If
End Sub
[/vbnet]
Success! You have learned How to Add and Retrieve Multiple Images in VB.Net!
RAR Extraction Password:
Password: luffypirates
For questions or any other concerns or thesis/capstone creation with documentation, you can contact me through the following:
E-Mail: [email protected]
Facebook: facebook.com/kirk.lavapiez
Contact No.: +639771069640
To download the sample project, click here.
Ian Hero L. Lavapiez
BSIT Graduate
System Analyst and Developer
Related topic(s) that you may like: