How to Get Value Member in a ComboBox Using C# and SQL Server
Now, in this tutorial, I will show you how to get the value member in a ComboBox by using C# and SQL Server. This method is very important in selecting the exact data that has been listed in the ComboBox.
For example, when you want to display the list of books in the ComboBox that came from the database but you only want to get its id. That’s where you use the value member to get the id of a book that is listed in the ComboBox.
Database : Click here for the database of this project.
Let’s begin:
Open Microsoft Visual Studio and create a new windows application for C#. Then, do the form just like this.
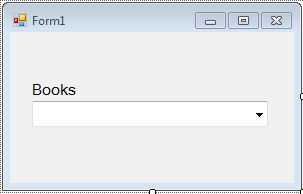
After setting up the form, go to the solution explorer and hit “code view” to fire the code editor.
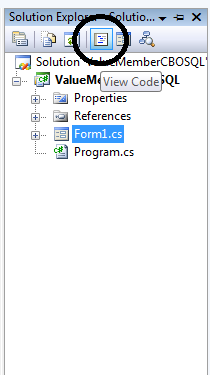
In the code editor, declare all the classes and variable that are needed.
Note: Put “using System.Data.SqlClient;” above the namespace to access sql server library.
//initializes new instance SqlConnection con = new SqlConnection(); SqlDataAdapter da = new SqlDataAdapter(); SqlCommand cmd = new SqlCommand(); DataTable dt = new DataTable(); //declare a variable string query;
After that, create a method for filling data in the list control of a ComboBox.
//a method for filling data in the combobox private void LoadBooks(string query, ComboBox cbo) { //opening connection con.Open(); try { //initialize a new instance of sqlcommand cmd = new SqlCommand(); //set a connection used by this instance of sqlcommand cmd.Connection = con; //set the sql statement to execute at the data source cmd.CommandText = query; //initialize a new instance of sqlDataAdapter da = new SqlDataAdapter(); //set the sql statement or stored procedure to execute at the data source da.SelectCommand = cmd; //initialize a new instance of DataTable dt = new DataTable(); //add or resfresh rows in the certain range in the datatable to match those in the data source. da.Fill(dt); //Get and set the data source of a comboBox cbo.DataSource = dt; //set the field of a table to display in the list control of a combo box cbo.DisplayMember = "BooksName"; //'SET THE ACTUAL VALUE OF THE //' ITEMS IN THE COMBOBOX cbo.ValueMember = "ID"; //Display first in the list control cbo.Text = "Select"; } catch (Exception ex) { //catching error MessageBox.Show(ex.Message); } //release all resources used by the component da.Dispose(); //closing connection con.Close(); }
Go back to the design view, double-click the form and do the following codes to establish the connection between SQL Server 2005 Express Edition and C#.net.
//set a connection between SQL server and Visual C# con.ConnectionString = "Data Source=.\\SQLEXPRESS;Database=dbbooks;trusted_connection=true;"; //set up a retrieve query query = "Select * From tblbooks"; LoadBooks(query, comboBox1 );//call a load method
Go back to the design view again, double-click a ComboBox and do the following codes for getting the value member of a ComboBox when selected.
MessageBox.Show("Book Id : " + comboBox1.SelectedValue.ToString());
Output:
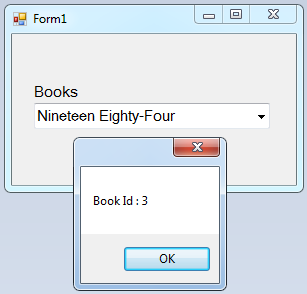
For all students who need a programmer for your thesis system or anyone who needs a source code in any a programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – TNT
ABOUT PROJECT | PROJECT DETAILS |
---|---|
Project Name : | How to Get Value Member in a ComboBox Using C# and SQL Server |
Project Platform : | C# |
Programming Language Used: | C# Programming Language |
Developer Name : | itsourcecode.com |
IDE Tool (Recommended): | Visual Studio 2019 |
Project Type : | Desktop Application |
Database: | MySQL Database |
Upload Date and Time: | July 1, 2016- 9:01 am |