In this tutorial about Submitting Multiple Forms with jQuery and Ajax, I will teach you on how to track the submitted forms without refreshing the page.
This method will allow you specify the forms that you are going to submit when you have multiple forms in the page. With this, you can avoid errors and you can post the fields according to your desire.
Submitting Multiple Forms with jQuey and Ajax Screenshots:
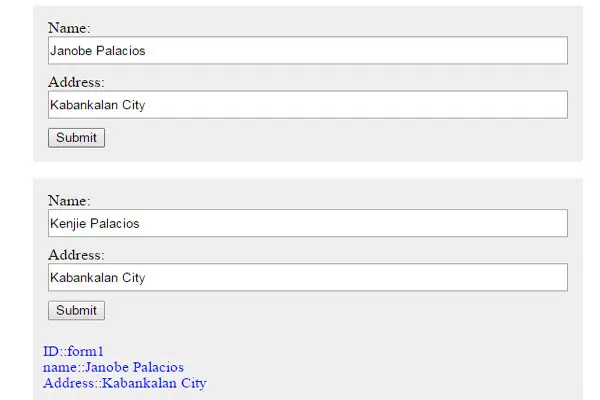
- Create a landing page and name it “index.php”.
- Create a Style for your desgn.
<style type="text/css"> @media(min-width:768px){ form{ position: inherit; margin: 0 400px 0 400px; } #results { background: #eee; padding: 10px; position: inherit; margin: 0 400px 0 400px; font-size: 15px; color: blue; } } form { background: #eee; padding: 10px; } form > div { padding:5px; } div > .label{ display: inline-block; width:auto; } div > .input { display: inline-block; height: 30px; width: 100%; } </style>
- Create the two forms and its curresponding fields.
<form name="form1" action="contact.php" method="POST" id="form1"> <div><label class="label">Name: </label><input type="text" name="name" id="name" class="input" required /></div> <div><label class="label">Address:</label> <input type="text" name="address" id="address" class="input" required /></div> <div><input type="submit" name="submit" value="Submit" /></div> </form> <br/> <form name="form2" action="contact.php" method="POST" id="form2"> <div><label class="label">Name: </label><input type="text" name="name" class="input"required /></div> <div><label class="label">Address:</label> <input type="text" name="address" class="input" required /></div> <div><input type="submit" name="submit" value="Submit" /></div> </form> <!-- Loading the result --> <div id="results"></div>
- Create a script for the method of submitting two forms.
<!-- jQuery extension --> <script type="text/javascript" src="jquery.js"></script> <script type="text/javascript"> $(document).ready(function() { $("form").submit(function() { // Getting the current form ID var frmID = $(this).attr('id'); var frmDetails = $('#'+frmID); $.ajax({ type: "POST", url: 'result.php?id=' + frmID, success: function (data) { // Displaying result into the div $('#results').html(data); }, } }); return false; }); }); </script>
- Create a result page and name it “result.php”.
<?php echo "ID::".$_GET['id'].'<br/>'; echo "name::".$_POST['name'].'<br/>'; echo "Address::".$_POST['address']; ?>
For all students who need programmer for your thesis system or anyone who needs a sourcecode in any programming languages. You can contact me @ :
Email – [email protected]
Mobile No. – 09305235027 – tnt