This tutorial will teach you on how to Writing Text file using Java. A text is a type of file contains a .txt extension. This tutorial uses BufferedWriter, FileWriter, IOException, and Scanner Libraries. When the program performs writing the file, the created file is located inside your Netbeans project. Netbeans project usually located in your Documents. Please follow all the steps to complete this tutorial.
Writing Text file Using Java Steps
- Create a new Java Class and name it what you want.
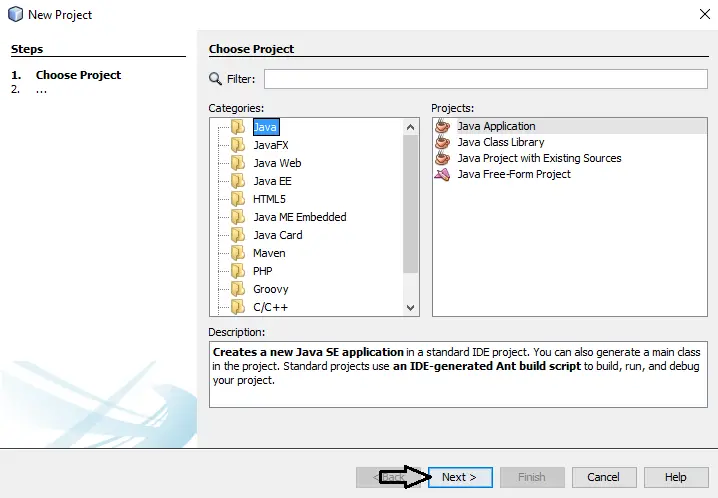
2. Insert the following import above your Class.
[java]import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;[/java]
3. Inside your main method, declare the following variables. Declare a scanner, string, and Buffer Writer variables.
[java]//scanner
Scanner input = new Scanner(System.in);
//the file to write
String fileName;
//buffer writer
BufferedWriter bWriter=null;[/java]
4. Insert the following statement for user input
[java]System.out.print(“Enter file name: “);
fileName = input.nextLine();[/java]
5. After step 4, insert the following codes below for writing the file.
[java]try{
//encoding
FileWriter fWriter = new FileWriter(fileName + “.txt”);
//file writer
bWriter = new BufferedWriter(fWriter);
//character to write
bWriter.write(“Marjohn I. Bebeloni”);
bWriter.newLine();
bWriter.write(“Tindalo St., Sainz, City of Mati”);
System.out.println(“The file name ” + fileName + ” is created!”);
}catch(IOException ex){
System.out.println(“Error writing the file”);
}finally{
//close the writer
try{
if (bWriter != null){
bWriter.close();
}
}catch(IOException e){
}
}[/java]
6. The output should look like the image below.
7. Complete Source Code of Writing Text file using Java
[java]import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Scanner;
public class FileWriting {
public static void main(String arg[]){
//scanner
Scanner input = new Scanner(System.in);
//the file to write
String fileName;
//buffer writer
BufferedWriter bWriter=null;
System.out.print(“Enter file name: “);
fileName = input.nextLine();
try{
//encoding
FileWriter fWriter = new FileWriter(fileName + “.txt”);
//file writer
bWriter = new BufferedWriter(fWriter);
//character to write
bWriter.write(“Marjohn I. Bebeloni”);
bWriter.newLine();
bWriter.write(“Tindalo St., Sainz, City of Mati”);
System.out.println(“The file name ” + fileName + ” is created!”);
}catch(IOException ex){
System.out.println(“Error writing the file”);
}finally{
//close the writer
try{
if (bWriter != null){
bWriter.close();
}
}catch(IOException e){
}
}
}
}[/java]
About The Writing a Text File In Java
Project Name: | Writing a Text File |
Language/s Used: | JAVA |
Database: | None |
Type: | Desktop Application |
Developer: | IT SOURCECODE |
Updates: | 0 |