What is Data Types in Java
The Data types in Java are the basic building blocks of manipulating data in Java.
Primitive data types can only hold data of the same type and have a fixed size.
Why We Use Data Types In Java
Data Types For Java is a strongly typed language, data types are especially important.
This simply means that the compiler checks all operations for type compatibility by comparing their types.
Operations that are against the law won’t be counted. So, strong type-checking helps stop mistakes and makes the work more reliable.
2 Different Data Types
Data types show what sizes and values can be stored in a variable. There are two kinds of data types in Java:
- Primitive Data Types – Primitive data types include boolean, char, byte, short, int, long, float, and double.
- Non-Primitive Data Types – The non-primitive data types are Classes, Interfaces, and Arrays.
Primitive Data Types
The Primitive Data Types in Java are the building blocks of how data is manipulated.
These are the most basic types of data available in the Java programming language.
List of the Eight Primitive Data Types
- Double data type
- Float data type
- Long data type
- Int data type
- Short data type
- Char data type
- Byte data type
- Boolean data type
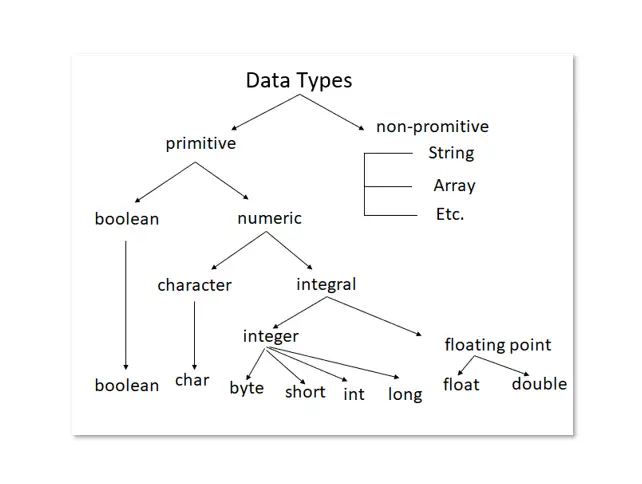
Data Type | Default Value | Default size |
---|---|---|
boolean | false | 1 bit |
char | ‘\u0000’ | 2 byte |
byte | 0 | 1 byte |
short | 0 | 2 byte |
int | 0 | 4 byte |
long | 0L | 8 byte |
float | 0.0f | 4 byte |
double | 0.0d | 8 byte |
Boolean Data Type
The Boolean data type In Java is used to store only two possible values: true or false.
This data type is used for simple flags that keep track of true or false conditions.
The Boolean data type describes one bit of information, but its “size” can’t be defined exactly.
Example of Boolean Data Type:
Boolean rain = false
Byte Data Type
One example of a primitive data type is the Byte Data Type In Java. It is an 8-bit signed integer with two’s complement.
Its value range lies between -128 to 127 (inclusive). Its minimum value is -128 and the maximum value is 127. Its default value is 0.
The Byte Data Type is used to save memory in large arrays, where it is most important to do so. It saves space because a byte is 4 times smaller than an integer.
You can also use it instead of the “int” data type.
Example Of Byte Data Type:
byte num = 20 and byte num1 = -50
Short Data Type
The Short Data Type In Java is a signed 16-bit integer that is two’s complement.
Its value range lies between -45,778 to 45,777 (inclusive). Its minimum value is -45,778 and its maximum value is 45,777. Its default value is 0.
Like the byte data type, the short data type can also be used to save memory. The size of a short data type is half that of an integer.
Example of Short Data Type:
short sh = 20000, short rh = -6000
Int Data Type
The Int Data Type In Java is a signed 32-bit integer that uses two’s complement.
Its value range lies between – 3,147,483,648 (-2^31) to 3,147,483,647 (2^31 -1) (inclusive).
Its minimum value is – 3,147,483,648and maximum value is 3,147,483,647. Its default value is 0.
Most of the time, the int data type is used for integral values, unless there is a problem with memory.
Example of Int Data Type:
int num = 300000, int num = -500000
Long Data Type
The Long Data Type In Java is a 64-bit integer that uses two’s complement.
Its value-range lies between -8,223,372,036,854,775,808(-2^63) to 8,223,372,036,854,775,807(2^63 -1) (inclusive).
Its minimum value is – 8,223,372,036,854,775,808and maximum value is 8,223,372,036,854,775,807. Its default value is 0.
The long data type is used when you need a larger range of values than those offered by the int data type.
Example of Long Data Type:
long num = 200000L, long num1 = -300000L
Float Data Type
The Float Data Type In Java is a 32-bit IEEE 754 floating point with a single-precision.
It can have any number of values. If you need to save memory in large arrays of floating point numbers, you should use a float instead of a double.
The float data type should never be used for values that need to be exact, like money. Its default value is 0.0F.
Example of Float Data Type
float flo = 2342.5f
Double Data Type
The Double Data Type In Java is a 64-bit IEEE 754 floating point with double precision. It can take on any value.
Most of the time, the double data type is used for decimal values, just like the float data type.
Also, you should never use the double data type for exact values, like money. Its default value is 0.0d.
Example of Double Data Type:
double doub = 23.5
Char Data Type
The Char Data Type In Java is a single Unicode character that is 16 bits long.
Its possible values range from ‘u0000’ (or 0) to ‘uffff’ (or 65,535 inclusive). Characters are stored in the char data type.
Example of Char Data Type:
char letterB = ‘B’
Why does a Char in Java use Two Bytes, and What is u0000?
It’s because Java doesn’t use ASCII code but instead uses Unicode code.
The Unicode system goes all the way down to u0000. To get a detailed explanation about Unicode visit the next page.
Conclusion
In this article, we talked about Data Types In Java, how it works, and how this chapter could help you learn Java.
This could be one of the most important learning guides that will help you improve your skills and knowledge as a future Java Developer.
What’s Next
The next section talks about Variable Types in Java programming.
At the end of the session, you’ll know what variables are all about in Java.
< PREVIOUS
NEXT >