This tutorial is all about Encrypting and Decrypting User Inputs Using Java. This tutorial will help you on how you can create a program that encrypts and decrypt the user inputs.
What is encrypt and decrypt? Encryption is a process in which the user inputs are converted into multiple types of characters so that vulnerable users cannot understand what the value is.
The decryption is a reverse mechanism, it means that the encrypted value will be converted to the original value and it is understandable by the users.
This java program uses two methods, one for the encrypt function and one for the decrypt function. Please follow all the steps to complete this tutorial.
Encrypting and Decrypting User Input Using Java
- Create a new Form inside your source package.

2. Design your form just like the image below. The form uses a java label and a text field element.

3. Insert the following codes below inside your class. The following codes are composed of two methods that handle encrypt and decrypt functions.
[java]//encrypting value
public static String encrypt(String key) {
String result = "";
int l = key.length();
char ch;
for(int i = 0; i < l; i++){
ch = key.charAt(i);
ch += 10;
result += ch;
}
return result;
}
//decrypting value
public static String decrypt(String key) {
String result = "";
int l = key.length();
char ch;
for(int i = 0; i < l; i++){
ch = key.charAt(i);
ch -= 10;
result += ch;
}
return result;
}[/java]
4. Right-click your user input text field and select Events > Key > KeyReleased to generate the key released method.
[java]private void jTextField1KeyReleased(java.awt.event.KeyEvent evt) {
} [/java]
5. Insert the codes below inside your newly created key released method.
[java]//for encryption
String en = encrypt(jTextField1.getText());
jTextField2.setText(en);
//for decryption
String de = decrypt(jTextField2.getText());
jTextField3.setText(de);[/java]
6. Run your program and the output should look like the image below.
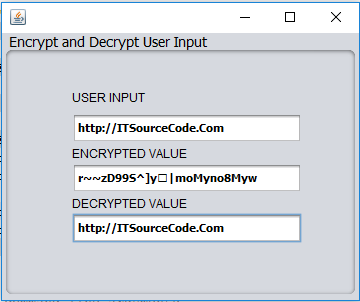
Encrypting and Decrypting values are very helpful to all programs that need security. Some programmers use the encrypt and decrypt features in the login function and user registration functions. It gives security to a program and avoids any vulnerable users.
About The Encrypting and Decrypting User Inputs In Java
<figure class="wp-block-table is-style-stripes">
<table>
<tbody>
<tr>
<td><strong>Project Name:</strong></td>
<td>Encrypting and Decrypting User Inputs</td>
</tr>
<tr>
<td><strong>Language/s Used:</strong></td>
<td>JAVA</td>
</tr>
<tr>
<td><strong>Database:</strong></td>
<td>None</td>
</tr>
<tr>
<td><strong>Type:</strong></td>
<td>Desktop Application</td>
</tr>
<tr>
<td><strong>Developer:</strong></td>
<td>IT SOURCECODE</td>
</tr>
<tr>
<td><strong>Updates:</strong></td>
<td>0</td>
</tr>
</tbody>
</table><figcaption><em><strong>Encrypting and Decrypting User Inputs In Java</strong>– Project Information</em></figcaption></figure>
After completing this tutorial, you will now learn and understand how your program is equipped with encrypt and decrypt features needed in your program and database security.
If you have questions or suggestions regarding the encrypt and decrypt features, feel free to use our contact information.
Related Articles You May Like: