This tutorial entitled “Allow Only Number Inputs in Java” will help you on how your text field accept only a number inputs.
This features is very helpful to a program that received contact information from a user to avoid wrong contact information stored in database.
The functions will execute if there is a key event happening in the java program. The program uses KeyListener, KeyAdapter, and KeyEvent.
Also uses KeyAdapter and KeyEvent Libararies. Follow all the steps to complete this tutorial.
Allow Only Number Inputs in Java Steps
- Create a new JFrame Form into your source package.

2. Design your form just like the image below. In my case, I want to accept three types of contact numbers, the home, work, and mobile numbers.
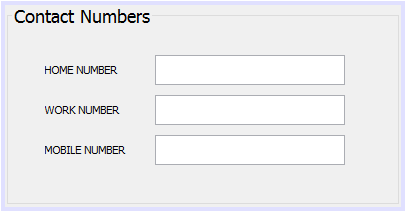
3. Insert the following codes to access the required libraries.
[java]import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;[/java]
4. Insert the following codes below inside your public method.
[java]//for home number
jTextField1.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e){
char c = e.getKeyChar();
if (!((c >= '0') && (c <= '9') ||
(c == KeyEvent.VK_BACK_SPACE) ||
(c == KeyEvent.VK_DELETE))) {
getToolkit().beep();
e.consume();
}
}
});
//for work number
jTextField2.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e){
char c = e.getKeyChar();
if (!((c >= '0') && (c <= '9') ||
(c == KeyEvent.VK_BACK_SPACE) ||
(c == KeyEvent.VK_DELETE))) {
getToolkit().beep();
e.consume();
}
}
});
//for jTextField3 number
jTextField1.addKeyListener(new KeyAdapter() {
@Override
public void keyTyped(KeyEvent e){
char c = e.getKeyChar();
if (!((c >= '0') && (c <= '9') ||
(c == KeyEvent.VK_BACK_SPACE) ||
(c == KeyEvent.VK_DELETE))) {
getToolkit().beep();
e.consume();
}
}
});[/java]
5. Run your program and the output should look like the image below.
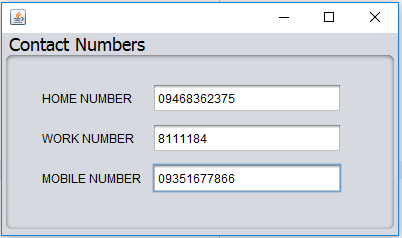
About The Allow Only Number Inputs In Java
<figure class="wp-block-table is-style-stripes">
<table>
<tbody>
<tr>
<td><strong>Project Name:</strong></td>
<td><strong>Allow Only Number Inputs</strong></td>
</tr>
<tr>
<td><strong>Language/s Used:</strong></td>
<td>JAVA</td>
</tr>
<tr>
<td><strong>Database:</strong></td>
<td>None</td>
</tr>
<tr>
<td><strong>Type:</strong></td>
<td>Desktop Application</td>
</tr>
<tr>
<td><strong>Developer:</strong></td>
<td>IT SOURCECODE</td>
</tr>
<tr>
<td><strong>Updates:</strong></td>
<td>0</td>
</tr>
</tbody>
</table><figcaption><em><strong>Allow Only Number Inputs In Java</strong>– Project Information</em></figcaption></figure>
After completing this tutorial, you are now understand the importance of restricting the text field especially in when handling contact information.
We can also allow text field to accept only letters. If you have comments about Allow only number inputs in Java, feel free to contact us.
Related Articles You May Like:
Create MySQL Connection in Java