Stack Data Structure in Java
Data structures are ways of storing data on a computer. One of the simplest data structures is a Stack in a Java program! In this article, we will discuss the stack in java and its methods.
What is a Stack Data Structure?
A stack is a collection of elements stored in a Last-In-First-Out principle, just like getting a card from a deck or popping a candy out of a candy dispenser.
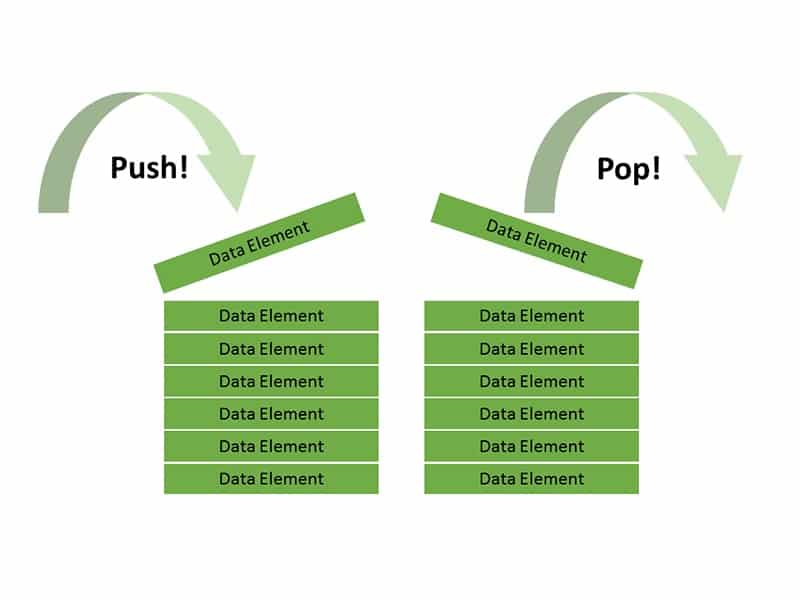
What are its methods?
The stack has two update methods and three accessor methods.
Update Methods
- push(e) – Adds element at the top of the stack.
- pop( ) – Removes and returns the top element from the stack (or null if the stack is empty).
Accessor Methods
- top( ) – Returns the top element of the stack without popping the element
- size( ) – Returns the number of elements in the stack.
- isEmpty( ) – Returns a boolean indicating whether the stack is empty.
How to implement Stacks in Java?
Stack in Java program is used by calling the java.util.Stacks library.
import java.util.Stack;
Now declare your stack.
public static void main(String[]args){ Stack<String> pupilNames = new Stack<>(); pupilNames.push("Anna"); pupilNames.push("Grace"); pupilNames.push("John"); pupilNames.push("Adam"); pupilNames.push("Ben"); System.out.println("Pupils entered the room: " + pupilNames); String lastPupil = pupilNames.pop(); System.out.println("Last pupil entered the room: " + lastPupil); }
Pupils entered the room: [Anna,Grace,John,Adam,Ben] Last pupil entered the room: Ben
About The Stack Data Structure In Java
<figure class="wp-block-table is-style-stripes">
<table>
<tbody>
<tr>
<td><strong>Project Name:</strong></td>
<td><strong>Stack Data Structure</strong></td>
</tr>
<tr>
<td><strong>Language/s Used:</strong></td>
<td>JAVA</td>
</tr>
<tr>
<td><strong>Database:</strong></td>
<td>None</td>
</tr>
<tr>
<td><strong>Type:</strong></td>
<td>Desktop Application</td>
</tr>
<tr>
<td><strong>Developer:</strong></td>
<td>IT SOURCECODE</td>
</tr>
<tr>
<td><strong>Updates:</strong></td>
<td>0</td>
</tr>
</tbody>
</table><figcaption><em><strong>Stack Data Structure In Java</strong>– Project Information</em></figcaption></figure>
Conclusion
Using this data structure is a good way to strengthen your source code and programming skills. For more information and references about data structures, check out callicoder.com and tutorialspoint.com
Enjoyed every bit of your blog article.Really looking forward to read more. Much obliged.
Thanks for sharing, this is a fantastic blog post.Really thank you! Really Cool.
Really enjoyed this article post.Thanks Again. Cool.