Hello guys! today i will teach you How to Create PDF file and send as attachment with email in PHP.
Creating a pdf file from mysql database and sending that dynamically generated pdf file as attachment with email is a very common feature in most of enterprise level of web application.
If any large web application we have to send email with dynamically generated pdf file for providing such type of data information.
In this tutorial we will discuss this topic in which we have learn how can we create dynamic pdf file from mysql database by using domPDF library and then after we will send that dynamically created pdf file will send as attachment with email using PHPMailer in PHP script.
So, here we have learn PHP send email with PDF attachment. I will describe how to fetch data from Mysql database and create PDF file from that data and attach that PDF file to an HTML email and lastly send it.
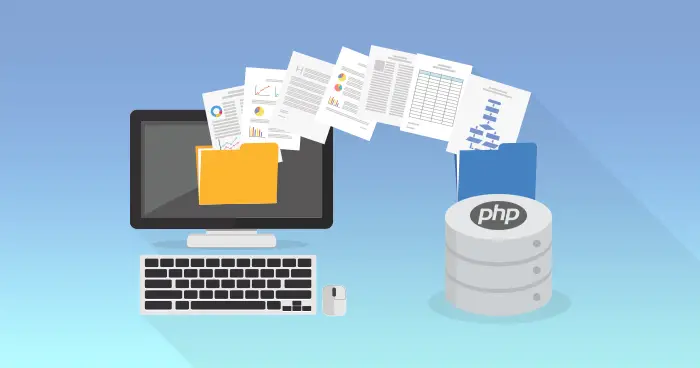
Insert this code in Index.ph
<?php //index.php $message = ''; $connect = new PDO("mysql:host=localhost;dbname=testing", "root", ""); function fetch_customer_data($connect) { $query = "SELECT * FROM tbl_customer"; $statement = $connect->prepare($query); $statement->execute(); $result = $statement->fetchAll(); $output = '
Name | Address | City | Postal Code | Country |
---|---|---|---|---|
‘.$row[“CustomerName”].’ | ‘.$row[“Address”].’ | ‘.$row[“City”].’ | ‘.$row[“PostalCode”].’ | ‘.$row[“Country”].’ |
'; return $output; } if(isset($_POST["action"])) { include('pdf.php'); $file_name = md5(rand()) . '.pdf'; $html_code = '<link rel="stylesheet" href="bootstrap.min.css">'; $html_code .= fetch_customer_data($connect); $pdf = new Pdf(); $pdf->load_html($html_code); $pdf->render(); $file = $pdf->output(); file_put_contents($file_name, $file); require 'class/class.phpmailer.php'; $mail = new PHPMailer; $mail->IsSMTP(); //Sets Mailer to send message using SMTP $mail->Host = 'smtpout.secureserver.net'; //Sets the SMTP hosts of your Email hosting, this for Godaddy $mail->Port = '80'; //Sets the default SMTP server port $mail->SMTPAuth = true; //Sets SMTP authentication. Utilizes the Username and Password variables $mail->Username = 'xxxxxxxxxx'; //Sets SMTP username $mail->Password = 'xxxxxxxxxx'; //Sets SMTP password $mail->SMTPSecure = ''; //Sets connection prefix. Options are "", "ssl" or "tls" $mail->From = 'itsourcecode.com'; //Sets the From email address for the message $mail->FromName = 'itsourcecode.com'; //Sets the From name of the message $mail->AddAddress('[email protected]', 'Name'); //Adds a "To" address $mail->WordWrap = 50; //Sets word wrapping on the body of the message to a given number of characters $mail->IsHTML(true); //Sets message type to HTML $mail->AddAttachment($file_name); //Adds an attachment from a path on the filesystem $mail->Subject = 'Customer Details'; //Sets the Subject of the message $mail->Body = 'Please Find Customer details in attach PDF File.'; //An HTML or plain text message body if($mail->Send()) //Send an Email. Return true on success or false on error { $message = '<label class="text-success">Customer Details has been send successfully...</label>'; } unlink($file_name); } ?> <!DOCTYPE html> <html> <head> <title>Create Dynamic PDF Send As Attachment with Email in PHP</title> http://jquery.min.js <link rel="stylesheet" href="bootstrap.min.css" /> http://bootstrap.min.js </head> <body> <br />
Create Dynamic PDF Send As Attachment with Email in PHP
<br /> <br /> </body> </html>
Add this code in pdf.php
<?php
//pdf.php
require_once 'dompdf/autoload.inc.php';
use Dompdf\Dompdf;
class Pdf extends Dompdf{
public function __construct(){
parent::__construct();
}
}
?>
If you have any comments or suggestions about How to Create PDF file and send as attachment with email in PHP Message us directly:
Download Source code here:
Other articles you might read also: